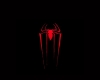
Ayuda con mi practica
Publicado por Sam (3 intervenciones) el 23/03/2022 21:12:50
Hola, espero me puedan ayudar a completar mi programa, en la cual me piden hacer una clase llamada Producto y después crear otra clase llamada ProductoConCaducidad en la cual herede la clase producto y esta deba capturar los datos anteriores, ahí lo tengo todo pero me pide que en la misma clase ProductoConCaducidad ponga la fecha de caducidad y ahí ya no se como hacerlo, espero y me puedan ayudar
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
#include <iostream>
#include <cmath>
using namespace std;
class Producto{
protected:
int SKU;
char Nombre[50];
float Precio;
public:
//constructor
virtual void leer()
{
cout<<"captura el SKU: ";
cin>>SKU;
fflush(stdin);
cout<<"digite el Nombre: "<<endl;
cin.getline(Nombre,50);
cout<<"capturar el Precio: ";
cin>>Precio;
}
virtual float CalcularProducto()
{
return Precio*0.16;
}
virtual void imprimir()
{
cout<<"Datos: "<<endl;
cout<<"SKU: "<<SKU<<endl;
cout<<"Nombre: " << Nombre <<endl;
cout<<"Precio: "<<Precio<<endl;
cout<<"Producto: "<<CalcularProducto()<<endl;
}
//destructor
};
class ProductoConCaducidad : Producto
{
public:
void leer()
{
ProductoConCaducidad::leer();
}
float CalcularCaducidad()
{
return 30;
}
void imprimir()
{
cout<<"Datos: "<<endl;
cout<<"SKU: "<<SKU<<endl;
cout<<"Nombre: " << Nombre << endl;
cout<<"Precio: "<<Precio<<endl;
cout<<"Caducidad: "<<CalcularCaducidad()<<endl;
}
};
int main(){
Producto *apProducto[20];
int n,i;
char respuesta;
do{
cout<<"¿Cuantos productos son?: ";
cin>>n;
}while (n<1 || n>20);
for(i=0; i<n; i++){
fflush(stdin);
cout<<"¿Caduce S/N?: " ;
cin>>respuesta;
if(respuesta =='S' || respuesta =='s'){
apProducto[i] = new Producto;
}
else{
apProducto[i] = new Producto;
}
apProducto[i]->leer();
}
system("CLS");
for(i=0; i<n; i++){
apProducto[i]->imprimir();
}
return 0;
}
Valora esta pregunta


0