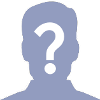
programa para registrar los productos, ayuda por favor
Publicado por carlos alexis (8 intervenciones) el 02/05/2022 03:43:23
Se requiere crear una aplicación que permita registrar productos, los atributos que define un producto
son:
1. Código, tipo de dato entero
2. Descripción, tipo de dato, string.
3. Cantidad en existencia, tipo de dato double
4. Cantidad mínima, tipo de dato, double
5. Cantidad máxima, tipo de dato, double.
6. Activo, tipo de dato, boleano.
La aplicación debe permitir:
a) Registrar productos nuevos.
b) Modificar productos existentes.
c) Consultar un producto por código.
d) Listar todos los productos ordenados por la descripción.
e) El programa debe utilizar un vector el cual contendrá los productos,
Std::vector<StProducto> lstProducto;
Requisitos.
a) El código del producto no se puede repetir.
b) El campo descripción debe tener un mínimo de cuatro caracteres.
c) Los Atributos: cantidad en existencia, cantidad minina y cantidad máxima no admiten valores
negativos.
d) El valor del campo o atributo cantidad mínima debe ser un valor menor o igual al valor del
campo cantidad máxima.
e) Debe crear un programa (archivo) que contenga
a. la especificación del producto.
b. Las funciones
i. Adicionar
ii. Modificar
iii. Consultar
iv. Listar
c. Cada funcionalidad debe recibir por referencia el vector de productos.
f) Debe crear un programa que utilice las funcionalidades del punto (e).
Diseño pantalla.
--- Registro de productos –
1. Nuevo
2. Modificar
3. Consultar
4. Listar
5. Salir
Opción : __
--- Nuevo Registro de producto –
Código : 1
Descripción : Martillo neumatico
Cantidades
En existencia : 4
Mínima : 1
Máxima : 2
El programa debe realizar las validaciones de lugar, si todos los valores de los atributos son
correctos entonces se incluirá el producto en el vector en caso contrario se desplegara el
mensaje <El producto no puede ser registrado, Existe un producto con el código introducido> .
El programa retornara el control al punto donde se invocó la funcionalidad.
--- Modificar Registro de productos –
Código : 1
Descripción : Martillo de percusión mecánica
Cantidades
En existencia : 4
Mínima : 1
Máxima : 2
El programa debe realizar las validaciones de lugar, si todos los valores de los atributos son
correctos y existe en el vector un producto con el código introducido entonces se reemplazaran
los datos de los atributos con los nuevos valores, en caso de no existir un producto con el código
introducido el programa desplegara el mensaje <El producto no puede ser modificado, NO
Existe un producto con el código introducido> .
El programa retornara el control al punto donde se invocó la funcionalidad.
--- Consultar Registro de productos –
Código : 1 [ Si existe en el vector un producto con código 1 entonces la aplicación desplegara
los datos <valores de los atributos> de dicho producto ]
Descripción : Martillo de percusión mecánica
Cantidades
En existencia : 4
Mínima : 1
Máxima : 2
[Si no existe un producto registrado con el código introducido se desplegara el mensaje :
Producto no existe , retornando el control del programa al lugar donde se invocó la
funcionalidad [Consultar]
--- Listar Registro de productos –
Código Descripción CantExistencia CantMaxima CantMinima Activo
10 Martillo Neumatico 4 6 2 S
11 Taladro XYZ 10 20 4 N
…. …………… .. .. .. ..
1. Luego de desplegar los productos el control del programa debe ser retornado al punto donde se
invocó la funcionalidad [Listar
son:
1. Código, tipo de dato entero
2. Descripción, tipo de dato, string.
3. Cantidad en existencia, tipo de dato double
4. Cantidad mínima, tipo de dato, double
5. Cantidad máxima, tipo de dato, double.
6. Activo, tipo de dato, boleano.
La aplicación debe permitir:
a) Registrar productos nuevos.
b) Modificar productos existentes.
c) Consultar un producto por código.
d) Listar todos los productos ordenados por la descripción.
e) El programa debe utilizar un vector el cual contendrá los productos,
Std::vector<StProducto> lstProducto;
Requisitos.
a) El código del producto no se puede repetir.
b) El campo descripción debe tener un mínimo de cuatro caracteres.
c) Los Atributos: cantidad en existencia, cantidad minina y cantidad máxima no admiten valores
negativos.
d) El valor del campo o atributo cantidad mínima debe ser un valor menor o igual al valor del
campo cantidad máxima.
e) Debe crear un programa (archivo) que contenga
a. la especificación del producto.
b. Las funciones
i. Adicionar
ii. Modificar
iii. Consultar
iv. Listar
c. Cada funcionalidad debe recibir por referencia el vector de productos.
f) Debe crear un programa que utilice las funcionalidades del punto (e).
Diseño pantalla.
--- Registro de productos –
1. Nuevo
2. Modificar
3. Consultar
4. Listar
5. Salir
Opción : __
--- Nuevo Registro de producto –
Código : 1
Descripción : Martillo neumatico
Cantidades
En existencia : 4
Mínima : 1
Máxima : 2
El programa debe realizar las validaciones de lugar, si todos los valores de los atributos son
correctos entonces se incluirá el producto en el vector en caso contrario se desplegara el
mensaje <El producto no puede ser registrado, Existe un producto con el código introducido> .
El programa retornara el control al punto donde se invocó la funcionalidad.
--- Modificar Registro de productos –
Código : 1
Descripción : Martillo de percusión mecánica
Cantidades
En existencia : 4
Mínima : 1
Máxima : 2
El programa debe realizar las validaciones de lugar, si todos los valores de los atributos son
correctos y existe en el vector un producto con el código introducido entonces se reemplazaran
los datos de los atributos con los nuevos valores, en caso de no existir un producto con el código
introducido el programa desplegara el mensaje <El producto no puede ser modificado, NO
Existe un producto con el código introducido> .
El programa retornara el control al punto donde se invocó la funcionalidad.
--- Consultar Registro de productos –
Código : 1 [ Si existe en el vector un producto con código 1 entonces la aplicación desplegara
los datos <valores de los atributos> de dicho producto ]
Descripción : Martillo de percusión mecánica
Cantidades
En existencia : 4
Mínima : 1
Máxima : 2
[Si no existe un producto registrado con el código introducido se desplegara el mensaje :
Producto no existe , retornando el control del programa al lugar donde se invocó la
funcionalidad [Consultar]
--- Listar Registro de productos –
Código Descripción CantExistencia CantMaxima CantMinima Activo
10 Martillo Neumatico 4 6 2 S
11 Taladro XYZ 10 20 4 N
…. …………… .. .. .. ..
1. Luego de desplegar los productos el control del programa debe ser retornado al punto donde se
invocó la funcionalidad [Listar
Valora esta pregunta


-1