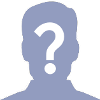
Ayuda con suma
Publicado por Carlos (1 intervención) el 07/04/2015 20:56:01
Necesito sacar el total de usuarios de un juego, para esto el programador usó PHP, pero ahora quiero sacar el número total de usuarios.
/php/MinecraftQuery.class.php
Esta es la página donde muestra los datos:
y para sacar usuarios y maximo de usuarios.
Y quedaría así: 100/200
Pero necesito sumar la cantidad de jugadorees conectados, un ejemplo, así: Query1 $infoPlayers + Query2 $infoPlayers
Gracias de antemano.
/php/MinecraftQuery.class.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
<?php
class MinecraftQueryException extends Exception
{
// Exception thrown by MinecraftQuery class
}
class MinecraftQuery
{
/*
* Class written by xPaw
*
* Website: http://xpaw.ru
* GitHub: https://github.com/xPaw/PHP-Minecraft-Query
*/
const STATISTIC = 0x00;
const HANDSHAKE = 0x09;
private $Socket;
private $Players;
private $Info;
public function Connect( $Ip, $Port = 25565, $Timeout = 3 )
{
if( !is_int( $Timeout ) || $Timeout < 0 )
{
throw new InvalidArgumentException( 'Timeout must be an integer.' );
}
$this->Socket = @FSockOpen( 'udp://' . $Ip, (int)$Port, $ErrNo, $ErrStr, $Timeout );
if( $ErrNo || $this->Socket === false )
{
throw new MinecraftQueryException( 'Could not create socket: ' . $ErrStr );
}
Stream_Set_Timeout( $this->Socket, $Timeout );
Stream_Set_Blocking( $this->Socket, true );
try
{
$Challenge = $this->GetChallenge( );
$this->GetStatus( $Challenge );
}
// We catch this because we want to close the socket, not very elegant
catch( MinecraftQueryException $e )
{
FClose( $this->Socket );
throw new MinecraftQueryException( $e->getMessage( ) );
}
FClose( $this->Socket );
}
public function GetInfo( )
{
return isset( $this->Info ) ? $this->Info : false;
}
public function GetPlayers( )
{
return isset( $this->Players ) ? $this->Players : false;
}
private function GetChallenge( )
{
$Data = $this->WriteData( self :: HANDSHAKE );
if( $Data === false )
{
throw new MinecraftQueryException( 'Failed to receive challenge.' );
}
return Pack( 'N', $Data );
}
private function GetStatus( $Challenge )
{
$Data = $this->WriteData( self :: STATISTIC, $Challenge . Pack( 'c*', 0x00, 0x00, 0x00, 0x00 ) );
if( !$Data )
{
throw new MinecraftQueryException( 'Failed to receive status.' );
}
$Last = '';
$Info = Array( );
$Data = SubStr( $Data, 11 ); // splitnum + 2 int
$Data = Explode( "\x00\x00\x01player_\x00\x00", $Data );
if( Count( $Data ) !== 2 )
{
throw new MinecraftQueryException( 'Failed to parse server\'s response.' );
}
$Players = SubStr( $Data[ 1 ], 0, -2 );
$Data = Explode( "\x00", $Data[ 0 ] );
// Array with known keys in order to validate the result
// It can happen that server sends custom strings containing bad things (who can know!)
$Keys = Array(
'hostname' => 'HostName',
'gametype' => 'GameType',
'version' => 'Version',
'plugins' => 'Plugins',
'map' => 'Map',
'numplayers' => 'Players',
'maxplayers' => 'MaxPlayers',
'hostport' => 'HostPort',
'hostip' => 'HostIp'
);
foreach( $Data as $Key => $Value )
{
if( ~$Key & 1 )
{
if( !Array_Key_Exists( $Value, $Keys ) )
{
$Last = false;
continue;
}
$Last = $Keys[ $Value ];
$Info[ $Last ] = '';
}
else if( $Last != false )
{
$Info[ $Last ] = $Value;
}
}
// Ints
$Info[ 'Players' ] = IntVal( $Info[ 'Players' ] );
$Info[ 'MaxPlayers' ] = IntVal( $Info[ 'MaxPlayers' ] );
$Info[ 'HostPort' ] = IntVal( $Info[ 'HostPort' ] );
// Parse "plugins", if any
if( $Info[ 'Plugins' ] )
{
$Data = Explode( ": ", $Info[ 'Plugins' ], 2 );
$Info[ 'RawPlugins' ] = $Info[ 'Plugins' ];
$Info[ 'Software' ] = $Data[ 0 ];
if( Count( $Data ) == 2 )
{
$Info[ 'Plugins' ] = Explode( "; ", $Data[ 1 ] );
}
}
else
{
$Info[ 'Software' ] = 'Vanilla';
}
$this->Info = $Info;
if( $Players )
{
$this->Players = Explode( "\x00", $Players );
}
}
private function WriteData( $Command, $Append = "" )
{
$Command = Pack( 'c*', 0xFE, 0xFD, $Command, 0x01, 0x02, 0x03, 0x04 ) . $Append;
$Length = StrLen( $Command );
if( $Length !== FWrite( $this->Socket, $Command, $Length ) )
{
throw new MinecraftQueryException( "Failed to write on socket." );
}
$Data = FRead( $this->Socket, 2048 );
if( $Data === false )
{
throw new MinecraftQueryException( "Failed to read from socket." );
}
if( StrLen( $Data ) < 5 || $Data[ 0 ] != $Command[ 2 ] )
{
return false;
}
return SubStr( $Data, 5 );
}
}
Esta es la página donde muestra los datos:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
<?php
define( 'MQ_SERVER_ADDR1', '192.95.23.41' );
define( 'MQ_SERVER_PORT1', 25530 );
define( 'MQ_SERVER_ADDR2', '192.95.10.88' );
define( 'MQ_SERVER_PORT2', 25531 );
define( 'MQ_SERVER_ADDR3', 'ts-mc.net' );
define( 'MQ_SERVER_PORT3', 25565 );
define( 'MQ_SERVER_ADDR4', 'ts-mc.net' );
define( 'MQ_SERVER_PORT4', 25565 );
define( 'MQ_SERVER_ADDR5', 'ts-mc.net' );
define( 'MQ_SERVER_PORT5', 25565 );
define( 'MQ_SERVER_ADDR6', 'ts-mc.net' );
define( 'MQ_SERVER_PORT6', 25565 );
define( 'MQ_TIMEOUT', 1 );
Error_Reporting( E_ALL | E_STRICT );
Ini_Set( 'display_errors', true );
require __DIR__ . '/php/MinecraftQuery.class.php';
$Timer = MicroTime( true );
$Query1 = new MinecraftQuery( );
try
{
$Query1->Connect( MQ_SERVER_ADDR1, MQ_SERVER_PORT1, MQ_TIMEOUT );
}
catch( MinecraftQueryException $e )
{
$Exception = $e;
}
$Timer = Number_Format( MicroTime( true ) - $Timer, 4, '.', '' );
$Query2 = new MinecraftQuery( );
try
{
$Query2->Connect( MQ_SERVER_ADDR2, MQ_SERVER_PORT2, MQ_TIMEOUT );
}
catch( MinecraftQueryException $e )
{
$Exception = $e;
}
$Query3 = new MinecraftQuery( );
try
{
$Query3->Connect( MQ_SERVER_ADDR3, MQ_SERVER_PORT3, MQ_TIMEOUT );
}
catch( MinecraftQueryException $e )
{
$Exception = $e;
}
$Query4 = new MinecraftQuery( );
try
{
$Query4->Connect( MQ_SERVER_ADDR1, MQ_SERVER_PORT1, MQ_TIMEOUT );
}
catch( MinecraftQueryException $e )
{
$Exception = $e;
}
$Timer = Number_Format( MicroTime( true ) - $Timer, 4, '.', '' );
$Query5 = new MinecraftQuery( );
try
{
$Query5->Connect( MQ_SERVER_ADDR2, MQ_SERVER_PORT2, MQ_TIMEOUT );
}
catch( MinecraftQueryException $e )
{
$Exception = $e;
}
$Query6 = new MinecraftQuery( );
try
{
$Query6->Connect( MQ_SERVER_ADDR3, MQ_SERVER_PORT3, MQ_TIMEOUT );
}
catch( MinecraftQueryException $e )
{
$Exception = $e;
}
?>
y para sacar usuarios y maximo de usuarios.
1
2
3
4
5
6
7
8
<?php
$info = $Query1->GetInfo();
echo $info['Players'];
?> /
<?php
$info = $Query1->GetInfo();
echo $info['MaxPlayers'];
?>
Pero necesito sumar la cantidad de jugadorees conectados, un ejemplo, así: Query1 $infoPlayers + Query2 $infoPlayers
1
2
3
4
5
6
7
8
9
<?php
$info = $Query1->GetInfo();
echo $info['Players'];
?>
sumas
<?php
$info = $Query2->GetInfo();
echo $info['Players'];
?>
Gracias de antemano.
Valora esta pregunta


0