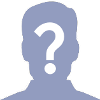
Error al implementar codigo PHP ayuda!
Publicado por Alex (2 intervenciones) el 15/06/2017 05:04:00
Tengo el siguiente problema con un ejercicio resuelto en PHP, el ejercicio contempla el registro de trabajadores dependiendo de si estos son empleados o docentes, el ejercicio propone implementar el codigo del archivo registroController.php para que el programa funcione. Lo he implementado como aparece en pantalla y al momento de grabar el trabajador no tengo problemas, el problema aparece cuando selecciono la vista planilla el navegador me devuelve lo siguiente:
Cual es el error que estoy cometiendo?, como indico todo el codigo ya estaba en el libro solo pide implementar el codigo de registroController.php para darle funcionalidad al programa.
Soy nuevo en PHP, gracias por la respuesta de antemano.
registroController.php
ITrabajador.php
Trabajador.php
Docente.php
Empleado.php
Planilla.php
main.php
planilla.php
registro.php
1
Fatal error: Uncaught Error: Call to undefined method Docente::getLista() in C:\xampp\htdocs\Curso PHP\poo\ejm\PeruTraining\view\planilla.php:38 Stack trace: #0 {main} thrown in C:\xampp\htdocs\Curso PHP\poo\ejm\PeruTraining\view\planilla.php on line 38
Cual es el error que estoy cometiendo?, como indico todo el codigo ya estaba en el libro solo pide implementar el codigo de registroController.php para darle funcionalidad al programa.
Soy nuevo en PHP, gracias por la respuesta de antemano.
registroController.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
<?php
session_start();
require_once '../model/Docente.php';
require_once '../model/Empleado.php';
require_once '../model/Planilla.php';
if (isset($_REQUEST["btnGrabar"])) {
// DATOS
if ($_REQUEST["tipo"] == "E") {
$trabajador = new Empleado(
$_REQUEST["apellidos"],
$_REQUEST["nombres"],
$_REQUEST["cargo"]);
}
else
if ($_REQUEST["tipo"] == "D") {
$trabajador = new Docente(
$_REQUEST["apellidos"],
$_REQUEST["nombres"],
$_REQUEST["horas"]);
}
// ACCESO AL OBJETO PLANILLA
if (isset($_SESSION["planilla"])) {
$planilla = unserialize($_SESSION["planilla"]);
} else {
$planilla = new Planilla();
}
// REGISTRAR TRABAJADOR
$planilla->agregarTrabajador($trabajador);
// REGISTRAR EL OBJETO TRABAJADOR EN SESION
$_SESSION["planilla"] = serialize($trabajador);
// DOCUMENTO DESTINO
$destino = "../view/registro.php";
}
header("location: $destino");
?>
ITrabajador.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
<?php
interface ITrabajador {
const TIPO_EMPLEADO = "Empleado";
const TIPO_DOCENTE = "Docente";
const EMPL_COORDINADOR = "Coordinador";
const EMPL_ASISTENTE = "Asistente";
const EMPL_SECRETARIA = "Secretaria";
const SUELDO_COORDINADOR = 3500.0;
const SUELDO_ASISTENTE = 2500.0;
const SUELDO_SECRETARIA = 1800;
const DOCENTE_PAGO_HORA = 50.0;
const BONO_EMPLEADO = 1.00; //100%
const BONO_DOCENTE = 0.60; //60%
function getSueldo();
function getBono();
}
?>
Trabajador.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
<?php
abstract class Trabajador {
private $tipo;
private $apellidos;
private $nombres;
function __construct($apellidos = null, $nombres = null) {
$this->setApellidos($apellidos);
$this->setNombres($nombres);
}
public function getTipo() {
return $this->tipo;
}
public function setTipo($tipo) {
$this->tipo = $tipo;
}
public function getApellidos() {
return $this->apellidos;
}
public function setApellidos($apellidos) {
$this->apellidos = $apellidos;
}
public function getNombres() {
return $this->nombres;
}
public function setNombres($nombres) {
$this->nombres = $nombres;
}
}
?>
Docente.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
<?php
require_once '../design/ITrabajador.php';
require_once '../design/Trabajador.php';
class Docente extends Trabajador implements ITrabajador {
private $horas;
function __construct($apellidos = null, $nombres = null, $horas = 0){
parent::__construct($apellidos, $nombres);
$this->setTipo(ITrabajador::TIPO_DOCENTE);
$this->setHoras($horas);
}
public function getHoras() {
return $this->horas;
}
public function setHoras($horas){
$this->horas = $horas;
}
public function getSueldo(){
$sueldo = $this->getHoras() * ITrabajador::DOCENTE_PAGO_HORA;
return $sueldo;
}
public function getBono(){
$bono = $this->getSueldo() * ITrabajador::BONO_DOCENTE;
return $bono;
}
}
// $obj = new Docente("Rosas", "Jose", 2);
// print_r($obj->getSueldo());
// echo "<br>";
// print_r($obj->getBono());
?>
Empleado.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
<?php
require_once '../design/ITrabajador.php';
require_once '../design/Trabajador.php';
class Empleado extends Trabajador implements ITrabajador {
private $cargo;
function __construct($apellidos = null, $nombres = null, $cargo = null) {
parent::__construct($apellidos, $nombres);
$this->setTipo(ITrabajador::TIPO_EMPLEADO);
$this->setCargo($cargo);
}
public function getCargo(){
return $this->cargo;
}
public function setCargo($cargo){
$this->cargo = $cargo;
}
public function getSueldo(){
$sueldo = 0.0;
$cargo = $this->getCargo();
if ($cargo == ITrabajador::EMPL_COORDINADOR) {
$sueldo = ITrabajador::SUELDO_COORDINADOR;
} elseif ($cargo == ITrabajador::EMPL_ASISTENTE) {
$sueldo = ITrabajador::SUELDO_ASISTENTE;
} elseif ($cargo == ITrabajador::EMPL_SECRETARIA) {
$sueldo = ITrabajador::SUELDO_SECRETARIA;
}
return $sueldo;
}
public function getBono(){
$bono = $this->getSueldo() * ITrabajador::BONO_EMPLEADO;
return $bono;
}
}
// $obj = new Empleado("Fernandez", "Rosa", ITrabajador::EMPL_COORDINADOR);
// print_r($obj->getSueldo());
?>
Planilla.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
<?php
class Planilla {
private $lista;
public function __construct(){
$this->lista = array();
}
public function agregarTrabajador(Trabajador $trab) {
$this->lista[] = $trab;
}
public function getLista(){
return $this->lista;
}
public function getTotal(){
$total = 0.0;
foreach ($this->lista as $trab) {
$total += ($trab->getSueldo() * $trab->getBono());
}
return $total;
}
}
?>
main.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
<?php
session_start();
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Peru Training</title>
</head>
<body>
<h1>Peru Training</h1>
<table border="1">
<tr>
<td><a href="registro.php">Registro</a></td>
<td><a href="planilla.php">Planilla</a></td>
<td><a href="salir.php">Salir</a></td>
</tr>
</table>
<p> </p>
<p> </p>
</body>
</html>
planilla.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
<?php
session_start();
require_once '../model/Docente.php';
require_once '../model/Empleado.php';
require_once '../model/Planilla.php';
if (isset($_SESSION["planilla"])) {
$planilla = unserialize($_SESSION["planilla"]);
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Peru Training</title>
</head>
<body>
<h1>Peru Training</h1>
<table border="1">
<tr>
<td><a href="registro.php">Registro</a></td>
<td><a href="planilla.php">Planilla</a></td>
<td><a href="salir.php">Salir</a></td>
</tr>
</table>
<h2>Planilla General</h2>
<?php if(isset($planilla)) { ?>
<table border="1">
<tr>
<td>Tipo</td>
<td>Apellidos</td>
<td>Nombres</td>
<td>Sueldo</td>
<td>Bono</td>
<td>Total</td>
</tr>
<?php foreach($planilla->getLista() as $obj) { ?>
<tr>
<td><?php echo($obj->getTipo()); ?></td>
<td><?php echo($obj->getApellidos()); ?></td>
<td><?php echo($obj->getNombres()); ?></td>
<td><?php echo($obj->getSueldo()); ?></td>
<td><?php echo($obj->getBono()); ?></td>
<td><?php echo($obj->getSueldo() + $obj->getBono()); ?></td>
</tr>
<?php } ?>
</table>
<p>Total: <?php echo($planilla->getTotal()); ?></p>
<?php } else { ?>
<p>No Existen trabajadores en planilla</p>
<?php } ?>
</body>
</html>
registro.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
<?php
session_start();
require_once '../design/ITrabajador.php';
if (isset($_REQUEST["msg"])) {
$msg = $_REQUEST["msg"];
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Peru Training</title>
<script type="text/javascript">
function funcCambioTipo(){
var tipo = document.getElementById("tipo").value;
var divCargo = document.getElementById("divCargo");
var divHoras = document.getElementById("divHoras");
if (tipo == "D") {
divCargo.style.display = "none";
divHoras.style.display = "";
} else {
divCargo.style.display = "";
divHoras.style.display = "none";
}
}
</script>
</head>
<body>
<h1>Peru Training</h1>
<table border="1">
<tr>
<td><a href="registro.php">Registro</a></td>
<td><a href="planilla.php">Planilla</a></td>
<td><a href="salir.php">Salir</a></td>
</tr>
</table>
<h2>Registro de Trabajador</h2>
<form name="form1" method="post" action="../controller/registroController.php">
Tipo
<select name="tipo" id="tipo" onchange="funcCambioTipo()">
<option value="D" selected>Docente</option>
<option value="E">Empleado</option>
</select>
Apellidos
<input type="text" name="apellidos">
Nombre
<input type="text" name="nombres">
<div id="divCargo" style="display:none;">
Cargo
<select name="cargo" id="cargo">
<option value="<?php echo(ITrabajador::EMPL_COORDINADOR); ?>">
<?php echo(ITrabajador::EMPL_COORDINADOR); ?>
</option>
<option value="<?php echo(ITrabajador::EMPL_ASISTENTE); ?>">
<?php echo(ITrabajador::EMPL_ASISTENTE); ?>
</option>
<option value="<?php echo(ITrabajador::EMPL_SECRETARIA); ?>">
<?php echo(ITrabajador::EMPL_SECRETARIA); ?>
</option>
</select>
</div>
<div id="divHoras">
Horas
<input type="text" name="horas" id="horas">
</div>
<input type="submit" value="Grabar" name="btnGrabar">
</form>
<?php if (isset($msg)) {
echo("<p>$msg</p>");
} ?>
</body>
</html>
Valora esta pregunta


0