Facturacion de productos y servicios con php
Publicado por Ramiro (20 intervenciones) el 19/02/2019 21:09:44
Hola gente necesito hacer un carrito de compras de servicios y productos. Alguien me podría ayudar con la parte de servicios que es la que no puedo hacer. Acá les dejo el código:
index.php
Data.php
agregar.php
Producto.php
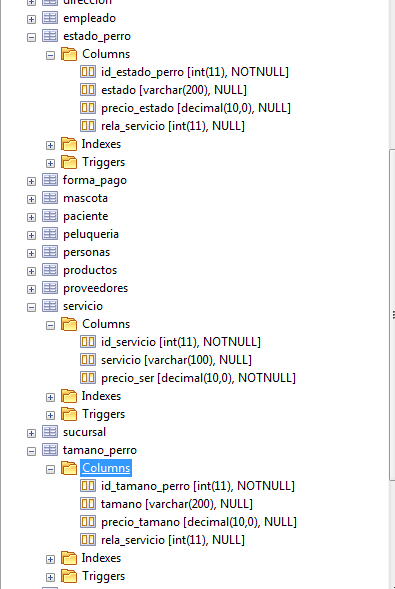
Esta es la parte de servicios esta relacionada con otras tablas, lo que quiero hacer es que a la hora de elegir un servicio cualquiera y elija el tamaño y el estado del perro me sumen todo en la tabla ventas
index.php
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
<?php
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<h1>Listado de Productos</h1>
<table border='1'>
<tr>
<th>Productos</th>
<th>Unidad</th>
<th>Categoria</th>
<th>Stock</th>
<th>Precio</th>
<th>Acccion</th>
</tr>
<?php
require_once "Data.php";
$d = new Data();
$productos = $d->getProductos();
foreach ($productos as $p) {
echo "<tr>";
echo "<td>".$p->nombre_pro."</td>";
echo "<td>".$p->unidad_medida."</td>";
echo "<td>".$p->categoria."</td>";
echo "<td>".$p->stock."</td>";
echo "<td>".$p->precio."</td>";
echo "<td>";
echo "<form action='agregar.php' method='post'>";
echo "<input type='hidden' name='txtId' value='".$p->id_producto."'>";
echo "<input type='hidden' name='txtNombre' value='".$p->nombre_pro."'>";
echo "<input type='hidden' name='txtMarca' value='".$p->marca."'>";
echo "<input type='hidden' name='txtDescripcion' value='".$p->descripcion."'>";
echo "<input type='hidden' name='txtStock' value='".$p->stock."'>";
echo "<input type='hidden' name='txtUnidad' value='".$p->unidad_medida."'>";
echo "<input type='hidden' name='txtCategoria' value='".$p->categoria."'>";
echo "<input type='hidden' name='txtImagen' value='".$p->imagen."'>";
echo "<input type='hidden' name='txtPrecio' value='".$p->precio."'>";
echo "<input type='number' name='txtCantidad'>";
echo "<input type='submit' name='btnAnadir' value='Añadir'>";
echo "</form>";
echo "</td>";
echo "</tr>";
}
?>
</table>
<h1>Listado de Servicios</h1>
<table border='1'>
<tr>
<th>Servicios</th>
<th>Precio base</th>
<th>Estado</th>
<th>Stock</th>
<th>Precio</th>
<th>Acccion</th>
</tr>
<?php
require_once "Datas.php";
$d = new Datas();
$servicio = $d->getServicios();
foreach ($servicio as $p) {
echo "<tr>";
echo "<td>".$p->servicio."</td>";
echo "<td>".$p->precio_ser."</td>";
}
?>
<td><select id="contacto" class="form-control col-md-9" name="contacto">
<option value = "">Seleccione una Opcion</option>
<?php
include "../Include/conexion.php";
$buscar = mysql_query ("select * from estado_perro;",$conexion) or
die ("Error en la consulta SQL");
while ($row = mysql_fetch_array ($buscar))
{
echo '<option value = "'.$row['id_estado_perro'].'">Estado: '.$row['estado'].' Precio: '.$row['precio_estado'].'</option>';
}
?>
</select></td>
</tr>
</table>
<?php
error_reporting(0);
session_start();
if (isset($_SESSION["carrito"])) {
$carrito = $_SESSION["carrito"];
echo "<h1>Listado de compras </h1>";
echo "<table border ='1'>";
echo "<tr>";
echo "<th>Productos/Servicio</th>";
echo "<th>Unidad</th>";
echo "<th>Categoria</th>";
echo "<th>Stock</th>";
echo "<th>Precio</th>";
echo "<th>Cantidad</th>";
echo "<th>Subtotal</th>";
echo "</tr>";
$total = 0;
foreach ($carrito as $p) {
echo "<tr>";
echo "<td>".$p->nombre_pro."</td>";
echo "<td>".$p->unidad_medida."</td>";
echo "<td>".$p->categoria."</td>";
echo "<td>".$p->stock."</td>";
echo "<td>".$p->precio."</td>";
echo "<td>".$p->cantidad."</td>";
echo "<td>$".$p->subTotal."</td>";
$total += $p->subTotal;
echo "</tr>";
}
echo "<tr>";
echo "<td colspan='6'><b>Total:</b></td>";
echo "<td><b>$$total</b></td>";
echo "</tr>";
echo "</table>";
}
?>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
<?php
require_once "conexion.php";
require_once "Producto.php";
class Data{
private $con;
public function __construct(){
$this->con = new Conexion("localhost", "sistema_veterinario", "root", "");
}
public function getProductos(){
$productos = array();
$query = "select *
from productos as p
join unidad_medida as u
on p.rela_unmedida = u.id_unmedida
join categoria as c
on p.rela_categoria= c.id_categoria";
$res = $this->con->ejecutar($query);
while ($reg = mysql_fetch_array($res)){
$p = new Producto();
$p->id_producto = $reg['id_producto'];
$p->nombre_pro = $reg['nombre_pro'];
$p->marca = $reg['marca'];
$p->descripcion = $reg['descripcion'];
$p->stock = $reg['stock'];
$p->unidad_medida = $reg['unidad_medida'];
$p->categoria = $reg['categoria'];
$p->imagen = $reg['imagen'];
$p->precio = $reg['precio'];
array_push($productos, $p);
}
return $productos;
}
}
?>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
<?php
require_once "Producto.php";
$p = new Producto();
$p->cantidad = $_POST["txtCantidad"];
$p->id_producto = $_POST["txtId"];
$p->nombre_pro = $_POST["txtNombre"];
$p->marca = $_POST["txtMarca"];
$p->descripcion = $_POST["txtDescripcion"];
$p->stock = $_POST["txtStock"];
$p->unidad_medida = $_POST["txtUnidad"];
$p->categoria = $_POST["txtCategoria"];
$p->imagen = $_POST["txtImagen"];
$p->precio = $_POST["txtPrecio"];
$p->subTotal = $p->precio * $p->cantidad;
session_start();
if(isset($_SESSION["carrito"])){
$carrito = $_SESSION["carrito"];
}else {
$carrito = array();
}
array_push($carrito, $p);
$_SESSION["carrito"] = $carrito;
header("location: index.php");
?>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
<?php
class Producto{
public $id_producto;
public $nombre_pro;
public $marca;
public $descripcion;
public $stock;
public $imagen;
public $precio;
public $unidad_medida;
public $categoria;
public $subtotal;
public $servicio;
public $precio_ser;
public $estado;
public $precio_estado;
public $tamano;
public $precio_tamano;
}
?>
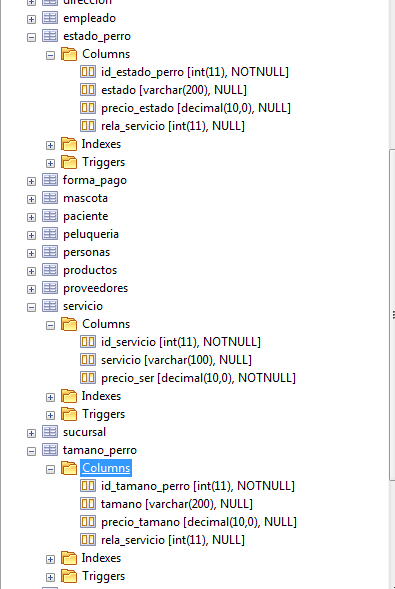
Esta es la parte de servicios esta relacionada con otras tablas, lo que quiero hacer es que a la hora de elegir un servicio cualquiera y elija el tamaño y el estado del perro me sumen todo en la tabla ventas
Valora esta pregunta


0