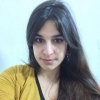
Como reproducir un stream y mp3 a la vez
Publicado por Gabriela (2 intervenciones) el 25/04/2023 17:01:39
Hola a todos, soy nueva por aquí... estoy estudiando programación, y es mi primer año. Necesito de su ayuda. Tengo el siguiente código:
Funciona genial el reproductor, y lo que deseo hacer es que cuando se haga clic en Play, tambien se cargue un archivo mp3 donde tengo una voz en off que se escucharía sobre la radio, como una marca de agua.
El código que me pasaron es este:
Los agrego juntos pero no puedo lograr que funcione, alguien me puede ayudar. Necesito que se active el mp3 cuando se escuche las emisoras de radio.
El código original del reproductor está https://ide.geeksforgeeks.org/online-html-editor/T3gdWUn4aX
Ojala me puedan ayudar como lo hacen siempre, besos!
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Music Player</title>
<!-- Load FontAwesome icons -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.13.0/css/all.min.css">
<!-- CSS style -->
<style>
body {
background-color: lightgreen;
<body>
<div class="player">
<div class="details">
<div class="now-playing">PLAYING x OF y</div>
<div class="track-art"></div>
<div class="track-name">Track Name</div>
<div class="track-artist">Track Artist</div>
</div>
<div class="buttons">
<div class="prev-track" onclick="prevTrack()"><i class="fa fa-step-backward fa-2x"></i></div>
<div class="playpause-track" onclick="playpauseTrack()"><i class="fa fa-play-circle fa-5x"></i></div>
<div class="next-track" onclick="nextTrack()"><i class="fa fa-step-forward fa-2x"></i></div>
</div>
<div class="slider_container">
<div class="current-time">00:00</div>
<input type="range" min="1" max="100" value="0" class="seek_slider" onchange="seekTo()">
<div class="total-duration">00:00</div>
</div>
<div class="slider_container">
<i class="fa fa-volume-down"></i>
<input type="range" min="1" max="100" value="99" class="volume_slider" onchange="setVolume()">
<i class="fa fa-volume-up"></i>
</div>
</div>
<!-- Main script for the player -->
<script>
let now_playing = document.querySelector(".now-playing");
let track_art = document.querySelector(".track-art");
let track_name = document.querySelector(".track-name");
let track_artist = document.querySelector(".track-artist");
let playpause_btn = document.querySelector(".playpause-track");
let next_btn = document.querySelector(".next-track");
let prev_btn = document.querySelector(".prev-track");
let seek_slider = document.querySelector(".seek_slider");
let volume_slider = document.querySelector(".volume_slider");
let curr_time = document.querySelector(".current-time");
let total_duration = document.querySelector(".total-duration");
let track_index = 0;
let isPlaying = false;
let updateTimer;
// Create new audio element
let curr_track = document.createElement('audio');
// Define the tracks that have to be played
let track_list = [
{
name: "Night Owl",
artist: "Broke For Free",
image: "https://images.pexels.com/photos/2264753/pexels-photo-2264753.jpeg?auto=compress&cs=tinysrgb&dpr=3&h=250&w=250",
path: "https://files.freemusicarchive.org/storage-freemusicarchive-org/music/WFMU/Broke_For_Free/Directionless_EP/Broke_For_Free_-_01_-_Night_Owl.mp3"
},
{
name: "Enthusiast",
artist: "Tours",
image: "https://images.pexels.com/photos/3100835/pexels-photo-3100835.jpeg?auto=compress&cs=tinysrgb&dpr=3&h=250&w=250",
path: "https://files.freemusicarchive.org/storage-freemusicarchive-org/music/no_curator/Tours/Enthusiast/Tours_-_01_-_Enthusiast.mp3"
},
{
name: "Shipping Lanes",
artist: "Chad Crouch",
image: "https://images.pexels.com/photos/1717969/pexels-photo-1717969.jpeg?auto=compress&cs=tinysrgb&dpr=3&h=250&w=250",
path: "https://files.freemusicarchive.org/storage-freemusicarchive-org/music/ccCommunity/Chad_Crouch/Arps/Chad_Crouch_-_Shipping_Lanes.mp3",
},
];
function loadTrack(track_index) {
clearInterval(updateTimer);
resetValues();
// Load a new track
curr_track.src = track_list[track_index].path;
curr_track.load();
// Update details of the track
track_art.style.backgroundImage = "url(" + track_list[track_index].image + ")";
track_name.textContent = track_list[track_index].name;
track_artist.textContent = track_list[track_index].artist;
now_playing.textContent = "PLAYING " + (track_index + 1) + " OF " + track_list.length;
// Set an interval of 1000 milliseconds for updating the seek slider
updateTimer = setInterval(seekUpdate, 1000);
// Move to the next track if the current one finishes playing
curr_track.addEventListener("ended", nextTrack);
// Apply a random background color
random_bg_color();
}
function random_bg_color() {
// Get a random number between 64 to 256 (for getting lighter colors)
let red = Math.floor(Math.random() * 256) + 64;
let green = Math.floor(Math.random() * 256) + 64;
let blue = Math.floor(Math.random() * 256) + 64;
// Construct a color withe the given values
let bgColor = "rgb(" + red + "," + green + "," + blue + ")";
// Set the background to that color
document.body.style.background = bgColor;
}
// Reset Values
function resetValues() {
curr_time.textContent = "00:00";
total_duration.textContent = "00:00";
seek_slider.value = 0;
}
function playpauseTrack() {
if (!isPlaying) playTrack();
else pauseTrack();
}
function playTrack() {
curr_track.play();
isPlaying = true;
// Replace icon with the pause icon
playpause_btn.innerHTML = '<i class="fa fa-pause-circle fa-5x"></i>';
}
function pauseTrack() {
curr_track.pause();
isPlaying = false;
// Replace icon with the play icon
playpause_btn.innerHTML = '<i class="fa fa-play-circle fa-5x"></i>';;
}
function nextTrack() {
if (track_index < track_list.length - 1)
track_index += 1;
else track_index = 0;
loadTrack(track_index);
playTrack();
}
function prevTrack() {
if (track_index > 0)
track_index -= 1;
else track_index = track_list.length;
loadTrack(track_index);
playTrack();
}
function seekTo() {
seekto = curr_track.duration * (seek_slider.value / 100);
curr_track.currentTime = seekto;
}
function setVolume() {
curr_track.volume = volume_slider.value / 100;
}
function seekUpdate() {
let seekPosition = 0;
// Check if the current track duration is a legible number
if (!isNaN(curr_track.duration)) {
seekPosition = curr_track.currentTime * (100 / curr_track.duration);
seek_slider.value = seekPosition;
// Calculate the time left and the total duration
let currentMinutes = Math.floor(curr_track.currentTime / 60);
let currentSeconds = Math.floor(curr_track.currentTime - currentMinutes * 60);
let durationMinutes = Math.floor(curr_track.duration / 60);
let durationSeconds = Math.floor(curr_track.duration - durationMinutes * 60);
// Adding a zero to the single digit time values
if (currentSeconds < 10) { currentSeconds = "0" + currentSeconds; }
if (durationSeconds < 10) { durationSeconds = "0" + durationSeconds; }
if (currentMinutes < 10) { currentMinutes = "0" + currentMinutes; }
if (durationMinutes < 10) { durationMinutes = "0" + durationMinutes; }
curr_time.textContent = currentMinutes + ":" + currentSeconds;
total_duration.textContent = durationMinutes + ":" + durationSeconds;
}
}
// Load the first track in the tracklist
loadTrack(track_index);
</script>
</body>
</html>
Funciona genial el reproductor, y lo que deseo hacer es que cuando se haga clic en Play, tambien se cargue un archivo mp3 donde tengo una voz en off que se escucharía sobre la radio, como una marca de agua.
El código que me pasaron es este:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
<script>
var aud2 = new Audio();
aud2.loop = true; // reproducir en bucle
var src = document.createElement("source");
src.type = "audio/mpeg";
src.src = "https://sitio.com/radio/voice.mp3"
aud2.appendChild(src);
document.getElementById("miaudio").onplay = function(){
aud2.play()
};
document.getElementById("miaudio").onpause = function(){
aud2.pause();
};
</script>
Los agrego juntos pero no puedo lograr que funcione, alguien me puede ayudar. Necesito que se active el mp3 cuando se escuche las emisoras de radio.
El código original del reproductor está https://ide.geeksforgeeks.org/online-html-editor/T3gdWUn4aX
Ojala me puedan ayudar como lo hacen siempre, besos!
Valora esta pregunta


0