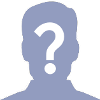
Ordenamiento con Punteros
Publicado por Antonio (12 intervenciones) el 07/06/2012 02:32:22
Hola. Tengo este problema de punteros y no se bien como resolverlo.
Insertar elementos enteros en forma ordenada (Ascendente). La lista debe ser doblemente enlazada y sin carro nulo. Los elementos deben visualizarse en forma Ascendente y Descendente.
Debe dar la opción de borrar por el final de la lista
Bien. Yo declaré la lista asi:
Y el procedimiento borrar asi:
Que supongo que están bien.
Pero mi problema es al ordenar los vectores. ¿Debería insertarlos como vienen y después ordenarlos o ir ordenando a medida que ingresa cada uno?
Esto es lo que intente hacer para el procedimiento Insertar:
De mas está decir que ese código no funciona pero espero que con eso se entienda la idea de lo que trato de hacer.
Necesitaría 3 procedimientos mas que son Insertar (Ascendente), Visualizar Ascendente y Visualizar Descendente.
Gracias.
Insertar elementos enteros en forma ordenada (Ascendente). La lista debe ser doblemente enlazada y sin carro nulo. Los elementos deben visualizarse en forma Ascendente y Descendente.
Debe dar la opción de borrar por el final de la lista
Bien. Yo declaré la lista asi:
1
2
3
4
5
6
7
8
type
tElem: Integer;
tLista: ^tNodo
tNodo = Record
Contenido: tElem;
Anterior: tLista;
Siguiente: tLista;
end;
Y el procedimiento borrar asi:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
Procedure Borrar (var Lista: tLista);
var
ListaAux: tLista;
begin
ListaAux := Lista;
if Lista = nil then
Writeln ('no hay elementos');
if Lista^.Siguiente = nil then
Lista:= nil
else
begin
While Lista^.Siguiente <> nil do
begin
Lista:= Lista^.Siguiente;
ListaAux:= nil
end;
end;
end;
Que supongo que están bien.
Pero mi problema es al ordenar los vectores. ¿Debería insertarlos como vienen y después ordenarlos o ir ordenando a medida que ingresa cada uno?
Esto es lo que intente hacer para el procedimiento Insertar:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
Procedure Agregar (Var Lista: tLista; Elem: tElem);
var
ListaAux: tLista;
begin
if Lista= nil then {Pregunta si la Lista está vacía}
begin
Lista^.Contenido:= Elem;
Lista^.Siguiente:= Nil;
Lista^.Anterior:= Nil
end;
If Lista^.Siguiente:= Nil then {Pregunta si hay un solo elemento}
begin
ListaAux:= Lista;
New (Lista);
Lista^.Contenido:= Elem;
If ListaAux^.Contenido < Lista^.Contenido then {Si lo que ingresamos ahora es mayor que lo que teníamos antes}
Lista^.Anterior:= ListaAux {Lo pone adelante}
else
Lista^.Siguiente:= ListaAux {Lo pone atras}
end;
De mas está decir que ese código no funciona pero espero que con eso se entienda la idea de lo que trato de hacer.
Necesitaría 3 procedimientos mas que son Insertar (Ascendente), Visualizar Ascendente y Visualizar Descendente.
Gracias.
Valora esta pregunta


0