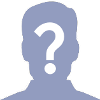
Lista enlazada y pérdidas de memoria
Publicado por Chris (7 intervenciones) el 30/07/2023 14:05:39
Buenas a todos,
estoy intentando implementar la lista enlazada de cero en C++ para una pequeña práctica, una agenda de contactos. La gestión de memoria me trae de cabeza, no consigo deshacerme de todos los memory leaks que detecta el depurador.
Os doy un poco de contexto:
Estoy en Windows con VSCode, usando el compilador MSVC.
tracking_memory.h
contact.h
list.h
list.cpp
main.cpp
Esta es la salida del depurador:
Detected memory leaks!
Dumping objects ->
{157} normal block at 0x011591D8, 8 bytes long.
Data: < 5 > E0 35 CD 00 00 00 00 00
{156} normal block at 0x011593D0, 8 bytes long.
Data: < 5 > BC 35 CD 00 00 00 00 00
{155} normal block at 0x01159440, 8 bytes long.
Data: < 5 > 88 35 CD 00 00 00 00 00
Object dump complete.
Creo que he liberado toda la memoria que he reservado, si no indicaría el archivo y la linea donde está el new que no fue liberado.
Llegados a este punto me pregunto si me es productivo seguir estudiando ED y Algoritmos en C++, llevo 2 días empleando más tiempo en la gestión de memoria que en estudiar el temario.
Gracias, un saludo.
estoy intentando implementar la lista enlazada de cero en C++ para una pequeña práctica, una agenda de contactos. La gestión de memoria me trae de cabeza, no consigo deshacerme de todos los memory leaks que detecta el depurador.
Os doy un poco de contexto:
Estoy en Windows con VSCode, usando el compilador MSVC.
tracking_memory.h
1
2
3
4
5
#ifdef _DEBUG
#define new new( _NORMAL_BLOCK , __FILE__ , __LINE__ )
#else
#define new new
#endif
contact.h
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
#ifndef CONTACT_H
#define CONTACT_H
#include <string>
const std::string BD = "bd.txt";
struct tContact
{
std::string name;
std::string surname;
std::string email;
int phone;
};
#endif //CONTACT_H
list.h
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
#ifndef LIST_H
#define LIST_H
#include "contact.h"
struct tNode;
typedef tNode *tList;
struct tNode
{
tContact contact;
tList next;
};
void printList(tList list);
void add(tList &list, tContact contact, bool &ok);
bool remove(tList &list, int index);
tList find(tList list, int code);
void loadData(tList &list);
void exportData(tList list);
void removeList(tList &list);
#endif //LIST_H
list.cpp
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
#include "list.h"
#include "tracking_memory.h"
void loadData(tList &list){
ifstream file;
char aux;
file.open(BD);
if(file.is_open()){
tContact contact;
tList last = NULL;
getline(file, contact.name);
while(contact.name != "-1"){
getline(file, contact.surname);
getline(file, contact.email);
file >> contact.phone;
file.get(aux);
file.get(aux);
if(list == NULL){
list = new tNode;
last = list;
}
else{
last->next = new tNode;
last = last->next;
}
last->contact = contact;
last->next = NULL;
getline(file, contact.name);
}
file.close();
}
}
void removeList(tList &list){
tList runner;
while(list != NULL){
runner = list;
list = list->next;
delete runner;
}
}
main.cpp
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
#define _CRTDBG_MAP_ALLOC
#include <stdlib.h>
#include <crtdbg.h>
#include <iostream>
using namespace std;
#include "list.h"
int main(){
tList list = NULL;
loadData(list);
removeList(list);
_CrtSetReportMode(_CRT_WARN, _CRTDBG_MODE_DEBUG);
_CrtDumpMemoryLeaks();
return 0;
}
Esta es la salida del depurador:
Detected memory leaks!
Dumping objects ->
{157} normal block at 0x011591D8, 8 bytes long.
Data: < 5 > E0 35 CD 00 00 00 00 00
{156} normal block at 0x011593D0, 8 bytes long.
Data: < 5 > BC 35 CD 00 00 00 00 00
{155} normal block at 0x01159440, 8 bytes long.
Data: < 5 > 88 35 CD 00 00 00 00 00
Object dump complete.
Creo que he liberado toda la memoria que he reservado, si no indicaría el archivo y la linea donde está el new que no fue liberado.
Llegados a este punto me pregunto si me es productivo seguir estudiando ED y Algoritmos en C++, llevo 2 días empleando más tiempo en la gestión de memoria que en estudiar el temario.
Gracias, un saludo.
Valora esta pregunta


0