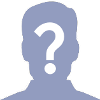
php code veryfication
Publicado por alberto (1 intervención) el 04/07/2021 17:31:49
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
<?php
//register.php
include('database_connection.php');
if(isset($_SESSION['user_id']))
{
header("location:index.php");
}
$message = '';
if(isset($_POST["register"]))
{
$query = "
SELECT * FROM register_user
WHERE user_email = :user_email
";
$statement = $connect->prepare($query);
$statement->execute(
array(
':user_email' => $_POST['user_email']
)
);
$no_of_row = $statement->rowCount();
if($no_of_row > 0)
{
$message = '<label class="text-danger">Email Already Exits</label>';
}
else
{
$user_password = rand(100000,999999);
$user_encrypted_password = password_hash($user_password, PASSWORD_DEFAULT);
$user_activation_code = md5(rand());
$insert_query = "
INSERT INTO register_user
(user_name, user_email, user_password, user_activation_code, user_email_status)
VALUES (:user_name, :user_email, :user_password, :user_activation_code, :user_email_status)
";
$statement = $connect->prepare($insert_query);
$statement->execute(
array(
':user_name' => $_POST['user_name'],
':user_email' => $_POST['user_email'],
':user_password' => $user_encrypted_password,
':user_activation_code' => $user_activation_code,
':user_email_status' => 'not verified'
)
);
$result = $statement->fetchAll();
if(isset($result))
{
$base_url = "http://localhost/tutorial/email-address-verification-script-using-php/"; //change this baseurl value as per your file path
$mail_body = "
<p>Hi ".$_POST['user_name'].",</p>
<p>Thanks for Registration. Your password is ".$user_password.", This password will work only after your email verification.</p>
<p>Please Open this link to verified your email address - ".$base_url."email_verification.php?activation_code=".$user_activation_code."
<p>Best Regards,<br />Webslesson</p>
";
require 'class/class.phpmailer.php';
$mail = new PHPMailer;
$mail->IsSMTP(); //Sets Mailer to send message using SMTP
$mail->Host = 'smtpout.secureserver.net'; //Sets the SMTP hosts of your Email hosting, this for Godaddy
$mail->Port = '80'; //Sets the default SMTP server port
$mail->SMTPAuth = true; //Sets SMTP authentication. Utilizes the Username and Password variables
$mail->Username = 'xxxxxxxx'; //Sets SMTP username
$mail->Password = 'xxxxxxxx'; //Sets SMTP password
$mail->SMTPSecure = ''; //Sets connection prefix. Options are "", "ssl" or "tls"
$mail->From = 'info@webslesson.info'; //Sets the From email address for the message
$mail->FromName = 'Webslesson'; //Sets the From name of the message
$mail->AddAddress($_POST['user_email'], $_POST['user_name']); //Adds a "To" address
$mail->WordWrap = 50; //Sets word wrapping on the body of the message to a given number of characters
$mail->IsHTML(true); //Sets message type to HTML
$mail->Subject = 'Email Verification'; //Sets the Subject of the message
$mail->Body = $mail_body; //An HTML or plain text message body
if($mail->Send()) //Send an Email. Return true on success or false on error
{
$message = '<label class="text-success">Register Done, Please check your mail.</label>';
}
}
}
}
?>
<!DOCTYPE html>
<html>
<head>
<title>PHP Register Login Script with Email Verification</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<body>
<br />
<div class="container" style="width:100%; max-width:600px">
<h2 align="center">PHP Register Login Script with Email Verification</h2>
<br />
<div class="panel panel-default">
<div class="panel-heading"><h4>Register</h4></div>
<div class="panel-body">
<form method="post" id="register_form">
<?php echo $message; ?>
<div class="form-group">
<label>User Name</label>
<input type="text" name="user_name" class="form-control" pattern="[a-zA-Z ]+" required />
</div>
<div class="form-group">
<label>User Email</label>
<input type="email" name="user_email" class="form-control" required />
</div>
<div class="form-group">
<input type="submit" name="register" id="register" value="Register" class="btn btn-info" />
</div>
</form>
<p align="right"><a href="login.php">Login</a></p>
</div>
</div>
</div>
</body>
</html>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
<?php
include('database_connection.php');
$message = '';
if(isset($_GET['activation_code']))
{
$query = "
SELECT * FROM register_user
WHERE user_activation_code = :user_activation_code
";
$statement = $connect->prepare($query);
$statement->execute(
array(
':user_activation_code' => $_GET['activation_code']
)
);
$no_of_row = $statement->rowCount();
if($no_of_row > 0)
{
$result = $statement->fetchAll();
foreach($result as $row)
{
if($row['user_email_status'] == 'not verified')
{
$update_query = "
UPDATE register_user
SET user_email_status = 'verified'
WHERE register_user_id = '".$row['register_user_id']."'
";
$statement = $connect->prepare($update_query);
$statement->execute();
$sub_result = $statement->fetchAll();
if(isset($sub_result))
{
$message = '<label class="text-success">Your Email Address Successfully Verified <br />You can login here - <a href="login.php">Login</a></label>';
}
}
else
{
$message = '<label class="text-info">Your Email Address Already Verified</label>';
}
}
}
else
{
$message = '<label class="text-danger">Invalid Link</label>';
}
}
?>
<!DOCTYPE html>
<html>
<head>
<title>PHP Register Login Script with Email Verification</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<h1 align="center">PHP Register Login Script with Email Verification</h1>
<h3><?php echo $message; ?></h3>
</div>
</body>
</html>
me dice code invalid al registrarme! help!
Valora esta pregunta


0