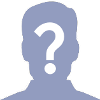
Web Scrapping EventBrite
Publicado por mariona (17 intervenciones) el 28/11/2023 13:45:48
Hola, quiero scrappear eventos de EventBrite.
Quiero coger titulo evento, imagen del evento, fecha evento, hora evento, localización evento, descripción evento y categorías evento.
Estoy haciendo el código en PyCharm y tengo el siguiente código:
from selenium import webdriver
from bs4 import BeautifulSoup
import csv
from dateutil import parser
from datetime import datetime, timedelta
from dateutil import tz
# Definir la función para normalizar fechas
def normalize_date(input_date):
if "today" in input_date.lower():
return datetime.now().date()
elif "tomorrow" in input_date.lower():
return (datetime.now() + timedelta(days=1)).date()
elif any(day in input_date.lower() for day in ["monday", "tuesday", "wednesday", "thursday", "friday", "saturday", "sunday"]):
# Encontramos un día de la semana, calculamos la próxima fecha
current_date = datetime.now().date()
days_until_next_day = (current_date.weekday() - ["monday", "tuesday", "wednesday", "thursday", "friday", "saturday", "sunday"].index(input_date.lower())) % 7
return (current_date + timedelta(days=days_until_next_day)).strftime("%a, %b %d")
try:
# Cambiar el formato de fecha para omitir las comillas
return datetime.strptime(input_date, "%a, %b %d").date().replace(year=datetime.now().year).strftime("%Y-%m-%d")
except ValueError:
try:
# Intentar convertir a formato de fecha directamente
return datetime.strptime(input_date, "%Y-%m-%d").date()
except ValueError:
return input_date # Devuelve la entrada original si no coincide con ninguno de los casos anteriores
# URL base de Eventbrite para eventos en Madrid
base_url = "https://www.eventbrite.com/d/spain--madrid/free--events--next-month/?page=1"
# Inicializa el controlador web de Selenium
driver = webdriver.Chrome() # Necesitas tener Chrome y chromedriver instalados
# Número de páginas a buscar
num_pages = 5
# Conjunto para evitar eventos duplicados
seen_events = set()
# Lista para almacenar los datos de los eventos
data = []
for page_number in range(1, num_pages + 1):
# Construye la URL de la página actual
url = f"{base_url}{page_number}"
# Realiza la solicitud HTTP para obtener la página
driver.get(url)
# Espera a que la página se cargue completamente (puedes ajustar el tiempo según sea necesario)
driver.implicitly_wait(10)
# Obtiene el contenido de la página después de que se haya ejecutado JavaScript
page_source = driver.page_source
# Parsea la página con BeautifulSoup
soup = BeautifulSoup(page_source, 'html.parser')
# Encuentra todos los elementos que contienen detalles de eventos
event_elements = soup.find_all("div", class_="Stack_root__1ksk7")
for event_element in event_elements:
# Encuentra el nombre del evento y la imagen directamente de la página principal
event_name_element = event_element.find("h2", class_="Typography_root__4bejd")
event_name = event_name_element.text.strip() if event_name_element else "Título no disponible"
# IMAGEN
image_element = soup.find("img", {"class": "event-card-image"})
# Extracta la URL de la imagen
image_url = image_element['src'] if image_element else "URL de imagen no disponible"
# print(image_url)
# URL DEL EVENTO
url_element = event_element.find("a", class_="event-card-link")
event_url = url_element['href'] if url_element else "URL de evento no disponible"
# DESCRIPCIÓN
description = event_url # Utiliza la URL del evento como descripción
# LOCALIZACIÓN
# Encuentra el elemento del párrafo de la ubicación
location_element = soup.find("p",
class_="Typography_root__4bejd #585163 Typography_body-md__4bejd event-card__clamp-line--one Typography_align-match-parent__4bejd")
# Extrae el texto de la ubicación sin las etiquetas HTML y sin la clase
location = location_element.text.strip() if location_element else "Information not available"
# FECHA
date_element = soup.find("p",
class_="Typography_root__4bejd #3a3247 Typography_body-md-bold__4bejd Typography_align-match-parent__4bejd")
# Extrae el texto de la fecha sin las etiquetas HTML y sin la clase
date_text = date_element.text.strip() if date_element else "Fecha no disponible"
# Procesar la fecha para obtener el formato deseado
date_parts = date_text.split('•')
date = normalize_date(date_parts[0].strip()) if date_parts else normalize_date(date_text)
# HORA
time = date_parts[1].strip() if date_parts else date_text
# Convertir a formato de 24 horas y manejar la zona horaria
try:
# Utilizar dateutil.parser para manejar diferentes formatos de hora y zona horaria
parsed_time = parser.parse(time)
# Convertir la hora a formato de 24 horas
time = parsed_time.strftime("%H:%M")
except ValueError:
# En caso de error al convertir, dejar el valor original
pass
# Verifica si el evento ya ha sido procesado
if event_name not in seen_events:
# Agrega los datos a la lista y al conjunto
data.append([event_name, image_url, event_url, location, date, time])
seen_events.add(event_name)
# Guardar datos en CSV
with open('eventbrite_main_info.csv', 'w', newline='', encoding='utf-8') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(["Name", "Image URL", "Event URL", "Location", "Date", "Time"])
for event in data:
writer.writerow(event)
print("Scraping completado. Los datos han sido guardados en eventbrite_main_info.csv.")
# Cerrar el controlador de Selenium
driver.quit()
Pero no consigo acceder a la url de cada evento para coger información de dentro de su url.
Por ejemplo, la variable descripción del evento y categorias evento las tendría que coger de dentro de la url del evento, no de la url base con el listado de los eventos.
No sé cómo hacer el código, ya que no soy programadora.
Si alguien me pudiera ayudar le estaría muy agradecida.
Quiero coger titulo evento, imagen del evento, fecha evento, hora evento, localización evento, descripción evento y categorías evento.
Estoy haciendo el código en PyCharm y tengo el siguiente código:
from selenium import webdriver
from bs4 import BeautifulSoup
import csv
from dateutil import parser
from datetime import datetime, timedelta
from dateutil import tz
# Definir la función para normalizar fechas
def normalize_date(input_date):
if "today" in input_date.lower():
return datetime.now().date()
elif "tomorrow" in input_date.lower():
return (datetime.now() + timedelta(days=1)).date()
elif any(day in input_date.lower() for day in ["monday", "tuesday", "wednesday", "thursday", "friday", "saturday", "sunday"]):
# Encontramos un día de la semana, calculamos la próxima fecha
current_date = datetime.now().date()
days_until_next_day = (current_date.weekday() - ["monday", "tuesday", "wednesday", "thursday", "friday", "saturday", "sunday"].index(input_date.lower())) % 7
return (current_date + timedelta(days=days_until_next_day)).strftime("%a, %b %d")
try:
# Cambiar el formato de fecha para omitir las comillas
return datetime.strptime(input_date, "%a, %b %d").date().replace(year=datetime.now().year).strftime("%Y-%m-%d")
except ValueError:
try:
# Intentar convertir a formato de fecha directamente
return datetime.strptime(input_date, "%Y-%m-%d").date()
except ValueError:
return input_date # Devuelve la entrada original si no coincide con ninguno de los casos anteriores
# URL base de Eventbrite para eventos en Madrid
base_url = "https://www.eventbrite.com/d/spain--madrid/free--events--next-month/?page=1"
# Inicializa el controlador web de Selenium
driver = webdriver.Chrome() # Necesitas tener Chrome y chromedriver instalados
# Número de páginas a buscar
num_pages = 5
# Conjunto para evitar eventos duplicados
seen_events = set()
# Lista para almacenar los datos de los eventos
data = []
for page_number in range(1, num_pages + 1):
# Construye la URL de la página actual
url = f"{base_url}{page_number}"
# Realiza la solicitud HTTP para obtener la página
driver.get(url)
# Espera a que la página se cargue completamente (puedes ajustar el tiempo según sea necesario)
driver.implicitly_wait(10)
# Obtiene el contenido de la página después de que se haya ejecutado JavaScript
page_source = driver.page_source
# Parsea la página con BeautifulSoup
soup = BeautifulSoup(page_source, 'html.parser')
# Encuentra todos los elementos que contienen detalles de eventos
event_elements = soup.find_all("div", class_="Stack_root__1ksk7")
for event_element in event_elements:
# Encuentra el nombre del evento y la imagen directamente de la página principal
event_name_element = event_element.find("h2", class_="Typography_root__4bejd")
event_name = event_name_element.text.strip() if event_name_element else "Título no disponible"
# IMAGEN
image_element = soup.find("img", {"class": "event-card-image"})
# Extracta la URL de la imagen
image_url = image_element['src'] if image_element else "URL de imagen no disponible"
# print(image_url)
# URL DEL EVENTO
url_element = event_element.find("a", class_="event-card-link")
event_url = url_element['href'] if url_element else "URL de evento no disponible"
# DESCRIPCIÓN
description = event_url # Utiliza la URL del evento como descripción
# LOCALIZACIÓN
# Encuentra el elemento del párrafo de la ubicación
location_element = soup.find("p",
class_="Typography_root__4bejd #585163 Typography_body-md__4bejd event-card__clamp-line--one Typography_align-match-parent__4bejd")
# Extrae el texto de la ubicación sin las etiquetas HTML y sin la clase
location = location_element.text.strip() if location_element else "Information not available"
# FECHA
date_element = soup.find("p",
class_="Typography_root__4bejd #3a3247 Typography_body-md-bold__4bejd Typography_align-match-parent__4bejd")
# Extrae el texto de la fecha sin las etiquetas HTML y sin la clase
date_text = date_element.text.strip() if date_element else "Fecha no disponible"
# Procesar la fecha para obtener el formato deseado
date_parts = date_text.split('•')
date = normalize_date(date_parts[0].strip()) if date_parts else normalize_date(date_text)
# HORA
time = date_parts[1].strip() if date_parts else date_text
# Convertir a formato de 24 horas y manejar la zona horaria
try:
# Utilizar dateutil.parser para manejar diferentes formatos de hora y zona horaria
parsed_time = parser.parse(time)
# Convertir la hora a formato de 24 horas
time = parsed_time.strftime("%H:%M")
except ValueError:
# En caso de error al convertir, dejar el valor original
pass
# Verifica si el evento ya ha sido procesado
if event_name not in seen_events:
# Agrega los datos a la lista y al conjunto
data.append([event_name, image_url, event_url, location, date, time])
seen_events.add(event_name)
# Guardar datos en CSV
with open('eventbrite_main_info.csv', 'w', newline='', encoding='utf-8') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(["Name", "Image URL", "Event URL", "Location", "Date", "Time"])
for event in data:
writer.writerow(event)
print("Scraping completado. Los datos han sido guardados en eventbrite_main_info.csv.")
# Cerrar el controlador de Selenium
driver.quit()
Pero no consigo acceder a la url de cada evento para coger información de dentro de su url.
Por ejemplo, la variable descripción del evento y categorias evento las tendría que coger de dentro de la url del evento, no de la url base con el listado de los eventos.
No sé cómo hacer el código, ya que no soy programadora.
Si alguien me pudiera ayudar le estaría muy agradecida.
Valora esta pregunta


0