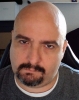
Error update registro en tabla
Publicado por Miguel (1 intervención) el 11/02/2024 16:20:17
Hola equipo,
Quería ver si vosotros podéis ver que está ocurriendo con un desarrollo que estoy haciendo que no doy con el error.
Tengo instalado:
Laravel 10.39.0
PHP 8.1.10
Es algo simple, creo yo, pero es simplemente la edición de un registro de una tabla,
tengo un formulario para editar que es el siguiente:
Esta es mi tabla de rutas:
Mi controller es el siguiente
y por ultimo mi Requests
La cosa es que puedo llegar al formulario de actualización (edit.blande.php) pero cuando le doy a actualizar no me actualiza el registro lo deja igual.
Ya estoy desesperado porque sé que es una cosa sencilla, pero no la logro ver, por favor a ver si alguien ve que ocurre, ya que o no entiendo que esta fallando.
Quería ver si vosotros podéis ver que está ocurriendo con un desarrollo que estoy haciendo que no doy con el error.
Tengo instalado:
Laravel 10.39.0
PHP 8.1.10
Es algo simple, creo yo, pero es simplemente la edición de un registro de una tabla,
tengo un formulario para editar que es el siguiente:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
@extends('layout')
@section('title', 'Actualizar Cliente')
@section('content')
<h3>Actualizar los datos del nuevo cliente</h3>
@if($errors->any())
<ul>
@foreach($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
@endif
<form method="POST" action="{{ route('customer.update', $customer) }}">
@csrf
@method('PATCH')
<div>
<label for="customer">Nombre Cliente:</label>
<input type="text" name="customer" id="customer" value="{{ $customer->customer, old('customer') }}">
{!! $errors->first('customer', '<br><small>:message</small>') !!}
</div>
<div>
<label for="email">Email:</label>
<input type="email" name="email" id="email" value="{{ $customer->email, old('email') }}">
{!! $errors->first('email', '<br><small>:message</small>') !!}
</div>
<div>
<h4>CustomerKey:</h4>
<div>
<label for="customerkeypro">Producción:</label>
<input type="text" name="customerkeypro" id="customerkeypro" value="{{ $customer->customerkeypro, old('customerkeypro') }}">
{!! $errors->first('customerkeypro', '<br><small>:message</small>') !!}
</div>
<div>
<label for="customerkeypre">PreProducción:</label>
<input type="text" name="customerkeypre" id="customerkeypre" value="{{ $customer->customerkeypre, old('customerkeypre') }}">
{!! $errors->first('customerkeypre', '<br><small>:message</small>') !!}
</div>
<button type="submit">Actualizar</button>
</form>
@endsection
Esta es mi tabla de rutas:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
<?php
use Faker\Guesser\Name;
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\CustomerController;
Route::view('/', 'home')->name('home');
Route::controller(CustomerController::class)->group(function () {
Route::get('/Clientes', 'index')->name('customer.index');
Route::get('/Clientes/crear', 'create')->name('customer.create');
Route::get('/Clientes/{id}/editar', 'edit')->name('customer.edit');
Route::patch('/Clientes/{id}', 'update')->name('customer.update');
Route::post('/Clientes/crear', 'store')->name('customer.store');
Route::get('/Clientes/{id}', 'show')->name('customer.show');
});
Route::view('/Procesados', 'showprocess')->name('showprocess');
Route::view('/Configuracion', 'showconfig')->name('showconfig');
Mi controller es el siguiente
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Mail\NewCustomer;
use App\Models\Customer;
use App\Http\Requests\SaverCustomerRequest;
use Illuminate\Http\RedirectResponse;
class CustomerController extends Controller
{
/**
* Display a listing of the resource.
*/
public function index()
{
return view('customers.index', [
/** para organizar por fecha Customer::orderBy('created_at', 'DESC')->paginate() */
'customers' => Customer::orderby('id', 'DESC')->paginate()
]);
}
/**
* Show the form for creating a new resource.
*/
public function create()
{
return view('customers.create');
}
/**
* Store a newly created resource in storage.
*/
public function store(SaverCustomerRequest $request)
{
Customer::create($request->validated());
/**
* Para que use App\Mail\NewCustomer; ponemos la \ antes de Mail
*/
\Mail::to('mrobiras@gmail.com')->queue(new NewCustomer($request->validated()));
return redirect()->route('customer.index');
}
/**
* Display the specified resource.
*/
public function show(string $id)
{
return view('customers.show', [
'customer' => Customer::findOrFail($id)
]);
}
/**
* Show the form for editing the specified resource.
*/
public function edit(string $id)
{
return view('customers.edit', [
'customer' => Customer::findOrFail($id)
]);
}
/**
* Update the specified resource in storage.
*/
public function update(Customer $customer, SaverCustomerRequest $request, string $id)
{
$customer->update( $request->validated() );
return redirect()->route('customer.show', $id);
}
/**
* Remove the specified resource from storage.
*/
public function destroy(string $id)
{
//
}
}
y por ultimo mi Requests
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
<?php
namespace App\Http\Requests;
use Illuminate\Foundation\Http\FormRequest;
class SaverCustomerRequest extends FormRequest
{
/**
* Determine if the user is authorized to make this request.
*/
public function authorize(): bool
{
return true;
}
/**
* Get the validation rules that apply to the request.
*
* @return array<string, \Illuminate\Contracts\Validation\ValidationRule|array<mixed>|string>
*/
public function rules(): array
{
return [
'customer' => 'required',
'email' => 'required|email',
'customerkeypro' => 'required|min:8',
'customerkeypre' => 'required|min:8'
];
}
/**
* Mensajes de Validación
*/
public function messages()
{
return [
'customer.required' => 'Debe indicar un nombre de Cliente'
];
}
}
La cosa es que puedo llegar al formulario de actualización (edit.blande.php) pero cuando le doy a actualizar no me actualiza el registro lo deja igual.
Ya estoy desesperado porque sé que es una cosa sencilla, pero no la logro ver, por favor a ver si alguien ve que ocurre, ya que o no entiendo que esta fallando.
Valora esta pregunta


0