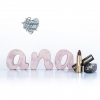
Modificar el existente para que máx nunca sea null, básicamente tengo que quitar código, me ayudáis?
Publicado por Ana (1 intervención) el 28/02/2018 23:39:38
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
public class AgeRange
{
private static IEnumerable<AgeRange> FacebookAgeRanges => new[]
{
AgeRange.New(13, 17),
AgeRange.New(18, 24),
AgeRange.New(25, 34),
AgeRange.New(35, 44),
AgeRange.New(45, 54),
AgeRange.New(55, 64),
AgeRange.New(65, null)
};
public int MinAge { get; private set; }
public int? MaxAge { get; private set; }
private AgeRange()
{
}
public static AgeRange New(
int minAge, int? maxAge = null)
{
return new AgeRange
{
MinAge = minAge,
MaxAge = maxAge
};
}
public static IEnumerable<AgeRange> GetRanges(
int minAge, int? maxAge = null, int splitBy = 0) // check limits
{
if (splitBy > 0)
{
while (minAge < maxAge.GetValueOrDefault(65))
{
var upperLimit = Math.Min(minAge + splitBy - 1, maxAge.GetValueOrDefault(64));
yield return AgeRange.New(minAge, upperLimit);
minAge = upperLimit + 1;
}
if (!maxAge.HasValue)
yield return AgeRange.New(65, null);
}
else
{
foreach (var ageRange in FacebookAgeRanges)
{
if (ageRange.Includes(minAge, maxAge))
yield return AgeRange.New(
Math.Max(ageRange.MinAge, minAge),
maxAge.HasValue
? Math.Min(maxAge.Value, ageRange.MaxAge.GetValueOrDefault(65))
: ageRange.MaxAge);
}
}
}
internal bool Includes(int minAge, int? maxAge)
{
if (minAge <= MinAge && MinAge <= maxAge.GetValueOrDefault(65)) return true;
if (!MaxAge.HasValue) return !maxAge.HasValue;
if (minAge <= MaxAge.Value && MaxAge.Value <= maxAge.GetValueOrDefault(65)) return true;
return false;
}
Valora esta pregunta


0