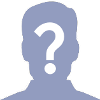
Ayuda Para realizar un programa
Publicado por Novato (10 intervenciones) el 11/03/2013 11:49:32
El programa que necesito hacer es un juego de loteria en el que el programa pide al usuario 6 numeros al azar comprendidos entre 1 y 49 y que sean introducidos en forma de cadena de caracteres separados por comas .
el programa que tengo hace eso pero pide al usuario los numeros uno a uno
¿Como puedo solucionarlo?
package primitiva;
import java.io.IOException;
import java.util.Random;
import java.util.Scanner;
public class Primitiva {
public static void main(String[] args) throws NumeroMal {
int[] jugada = jugar();
System.out.print("Combinación jugada: ");
imprimir(jugada);
int[] sorteo = sortear();
System.out.print("\nCombinación ganadora ");
imprimir(sorteo);
System.out.println("\nNúmero de aciertos " + comprobar(jugada, sorteo));
}
public static final int NUMEROS = 6;
public static Scanner entrada= new Scanner(System.in);
public static int[] jugar() throws NumeroMal {
int[] combinacion = new int[NUMEROS];
try{
for (int i=0; i<NUMEROS; i++) {
do {
System.out.print("introduzca un numero(" + i + ") entre el 1 y el 49: ");
combinacion[i] = entrada.nextInt();
} while ((combinacion[i] < 1) || (combinacion[i] > 49) || contiene(combinacion, combinacion[i], i));
}throw new NumeroMal("no admite otros numeros.");
}catch(NumeroMal nm){
System.out.println("\n"+nm.getMessage());
}
//catch () {
// System.out.println("\nError de entrada/salida: " + ioe.toString());
// }
catch (Exception e) {
// Captura cualquier otra excepción
System.out.println("Excepción: " + e.toString());
}
return combinacion;
}
public static boolean contiene(int[] array, int numero, int rellenos) {
boolean resultado = false;
for (int i=0; i<rellenos;i++) {
if (numero == array[i]) {
resultado = true;
}
}
return resultado;
}
public static int[] sortear() {
int combinacion[] = new int[NUMEROS];
Random random = new Random();
for (int i=0; i<NUMEROS; i++) {
do {
combinacion[i] = random.nextInt(49)+1;
} while (contiene(combinacion, combinacion[i], i));
}
return combinacion;
}
public static int comprobar(int[] combinacion1, int[] combinacion2) {
int aciertos = 0;
for (int i=0; i<NUMEROS; i++){
if (contiene(combinacion2, combinacion1[i], NUMEROS)) {
aciertos++;
}
}
return aciertos;
}
public static void imprimir(int[] combinacion) {
for (int i=0; i<combinacion.length; i++) {
System.out.print(combinacion[i] + " ");
}
}
}
class NumeroMal extends Exception{
public NumeroMal(){
super("Excepcion definida por el usuario:NUMERO INCORRECTO");
}
public NumeroMal(String msg){
super("Excepcion definida por el usuario:"+msg);
}
}
el programa que tengo hace eso pero pide al usuario los numeros uno a uno
¿Como puedo solucionarlo?
package primitiva;
import java.io.IOException;
import java.util.Random;
import java.util.Scanner;
public class Primitiva {
public static void main(String[] args) throws NumeroMal {
int[] jugada = jugar();
System.out.print("Combinación jugada: ");
imprimir(jugada);
int[] sorteo = sortear();
System.out.print("\nCombinación ganadora ");
imprimir(sorteo);
System.out.println("\nNúmero de aciertos " + comprobar(jugada, sorteo));
}
public static final int NUMEROS = 6;
public static Scanner entrada= new Scanner(System.in);
public static int[] jugar() throws NumeroMal {
int[] combinacion = new int[NUMEROS];
try{
for (int i=0; i<NUMEROS; i++) {
do {
System.out.print("introduzca un numero(" + i + ") entre el 1 y el 49: ");
combinacion[i] = entrada.nextInt();
} while ((combinacion[i] < 1) || (combinacion[i] > 49) || contiene(combinacion, combinacion[i], i));
}throw new NumeroMal("no admite otros numeros.");
}catch(NumeroMal nm){
System.out.println("\n"+nm.getMessage());
}
//catch () {
// System.out.println("\nError de entrada/salida: " + ioe.toString());
// }
catch (Exception e) {
// Captura cualquier otra excepción
System.out.println("Excepción: " + e.toString());
}
return combinacion;
}
public static boolean contiene(int[] array, int numero, int rellenos) {
boolean resultado = false;
for (int i=0; i<rellenos;i++) {
if (numero == array[i]) {
resultado = true;
}
}
return resultado;
}
public static int[] sortear() {
int combinacion[] = new int[NUMEROS];
Random random = new Random();
for (int i=0; i<NUMEROS; i++) {
do {
combinacion[i] = random.nextInt(49)+1;
} while (contiene(combinacion, combinacion[i], i));
}
return combinacion;
}
public static int comprobar(int[] combinacion1, int[] combinacion2) {
int aciertos = 0;
for (int i=0; i<NUMEROS; i++){
if (contiene(combinacion2, combinacion1[i], NUMEROS)) {
aciertos++;
}
}
return aciertos;
}
public static void imprimir(int[] combinacion) {
for (int i=0; i<combinacion.length; i++) {
System.out.print(combinacion[i] + " ");
}
}
}
class NumeroMal extends Exception{
public NumeroMal(){
super("Excepcion definida por el usuario:NUMERO INCORRECTO");
}
public NumeroMal(String msg){
super("Excepcion definida por el usuario:"+msg);
}
}
Valora esta pregunta


1