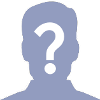
Abrir una ventana desde un boton en un JPanel
Publicado por Manuel (9 intervenciones) el 06/10/2014 04:40:45
Tengo una venta de un inicio de sesión, lo que quiero es que cuando yo de click en el botón "ingresar", la ventana de inicio de sesión desaparezca y se abra otra. Lo he intentado pero el código que pongo no funciona.
En esta ultima parte del código es donde deberia estar el error.
Saben cual es el error o que podría hacer para me funcionara? Les agradezco muchísimo.
Muchas Gracias
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
package vista;
import java.awt.BorderLayout;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.awt.LayoutManager;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
/**
*
* @author Manuel F. Del Toro
*
*/
public class VentanaInicioSesion extends JFrame {
JButton buttonIngresar;
public static void main(String[] args) {
JFrame login = new JFrame("Sistema de Información Yamantaka");
PanelImagen_Login imagen = new PanelImagen_Login();
PanelDatos_Login datos = new PanelDatos_Login();
login.add(imagen, BorderLayout.NORTH);
login.add(datos, BorderLayout.CENTER);
login.setSize(380, 365);
login.setVisible(true);
login.setResizable(false);
login.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
package vista;
import java.awt.Color;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
import javax.swing.border.Border;
public class PanelDatos_Login extends JPanel implements ActionListener{
private JComboBox<String> comboTipoUsuario;
private JLabel labelNombreUsuario;
private JTextField textNombreUsuario;
private JLabel labelContraseña;
private JPasswordField passUsuario;
private JButton buttonIngresar;
public PanelDatos_Login(){
String[] tipoUsuario = {"Cliente", "Administrador", "Asesor de Ventas"};
comboTipoUsuario = new JComboBox<String>(tipoUsuario);
labelNombreUsuario = new JLabel("Nombre de Usuario:");
textNombreUsuario = new JTextField(10);
labelContraseña = new JLabel("Contraseña:");
passUsuario = new JPasswordField(10);
buttonIngresar = new JButton("Ingresar");
buttonIngresar.addActionListener(this);
// Borde
Color colorBorde = new Color(84,33,29);
Border interior = BorderFactory.createLineBorder(colorBorde, 1, false);
Border bordeInterior = BorderFactory.createTitledBorder(interior, "Inicio de Sesión", 0, 0);
Border bordeExterior = BorderFactory.createEmptyBorder(5, 5, 10, 10);
setBorder(BorderFactory.createCompoundBorder(bordeExterior, bordeInterior));
/// GridBagLayout
setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridx = 0; // Fila
gbc.gridy = 0; // Columna
gbc.gridwidth = 2; // Columnas que ocupa
gbc.gridheight =1; // Filas que ocupa
//gbc.weightx = 1;
//gbc.weighty = 0;
gbc.insets = new Insets(5, 15, 15, 1);
gbc.anchor = GridBagConstraints.NORTH;
gbc.fill = GridBagConstraints.CENTER;
this.add(comboTipoUsuario, gbc);
gbc.gridx = 0;
gbc.gridy = 1;
gbc.gridwidth = 1;
gbc.gridheight = 1;
gbc.weightx = 1;
gbc.weighty = 0;
gbc.insets = new Insets(5, 15, 5, 1);
gbc.anchor = GridBagConstraints.NORTH;
gbc.fill = GridBagConstraints.BOTH;
this.add(labelNombreUsuario, gbc);
gbc.gridx = 1;
gbc.gridy = 1;
gbc.fill = GridBagConstraints.BOTH;
gbc.insets = new Insets(5, 15, 4, 1);
this.add(textNombreUsuario, gbc);
gbc.gridx = 0;
gbc.gridy = 2;
gbc.fill = GridBagConstraints.BOTH;
gbc.insets = new Insets(1, 15, 4, 1);
this.add(labelContraseña,gbc);
gbc.gridx = 1;
gbc.gridy = 2;
gbc.fill = GridBagConstraints.BOTH;
gbc.insets = new Insets(5, 15, 10, 1);
this.add(passUsuario, gbc);
gbc.gridx = 1;
gbc.gridy = 3;
gbc.fill = GridBagConstraints.BOTH;
gbc.insets = new Insets(15, 4, 4, 10);
this.add(buttonIngresar, gbc);
}
public void actionPerformed(ActionEvent e){
if (e.getSource() == buttonIngresar) {
VentanaPrincipal vp = new VentanaPrincipal();
VentanaInicioSesion vlogin = new VentanaInicioSesion();
vp.setVisible(true);
vlogin.setVisible(false);
}
}
En esta ultima parte del código es donde deberia estar el error.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
package vista;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.SwingConstants;
public class PanelImagen_Login extends JPanel {
private JLabel imagen;
public PanelImagen_Login(){
Icon titulo = new ImageIcon(getClass().getResource("titulo2.gif"));
imagen = new JLabel(titulo);
add(imagen);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
package vista;
import javax.swing.JFrame;
/**
*
* @author Manuel F. Del Toro
*
*/
public class VentanaPrincipal extends JFrame{
public static void main(String[] args) {
JFrame ventanaPrincipal = new JFrame("Sistema de Información Yamantaka");
ventanaPrincipal.setVisible(true);
ventanaPrincipal.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
ventanaPrincipal.setSize(800,600);
}
}
Saben cual es el error o que podría hacer para me funcionara? Les agradezco muchísimo.
Muchas Gracias
Valora esta pregunta


0