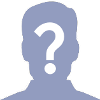
Tengo una duda(Ayudarme por fabor)
Publicado por Rubén (1 intervención) el 22/07/2015 16:12:32
Buenas, soy nuevo en el foro, hace unos meses que jugueteo con java y tengo una consulta.
Estoy haciendo la parte de sockets y server. Estoy intentando tener en frames separados un inicio de sesión básico y un chat. La idea era que con el inicio de sesion introduzcas un usuario y en cuanto este entrar al chat. Bien he ido provando formas y tengo algo que medio se me asemeja. Ahí va el codigo.
Bien, con esto me pasa una cosa compleja. Y es que quiero hacer que el inicio de sesión vaya primero y luego al cerrar abra el chat. La ventana principal es la del chat y abre el inicio de sesión para pedir usuario, luego hace dispose(). Intenté que el inicio de sesión fuese principal y chat secundario pero he tenido fallos con la conexión de esta manera.
Ahora lo que quiero que me ayudéis si podeis es en esto, ¿como puedo hacer que inicio de sesión se abra primero y luego abra chat? ¿como podría hacer que el programa sepa si inicio de sesión esta dispose()?¿como ordenarían el código o escribirían el código con sentido?
Estoy haciendo la parte de sockets y server. Estoy intentando tener en frames separados un inicio de sesión básico y un chat. La idea era que con el inicio de sesion introduzcas un usuario y en cuanto este entrar al chat. Bien he ido provando formas y tengo algo que medio se me asemeja. Ahí va el codigo.
Inicio sesion
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
public class Inicio_Sesion {
public JFrame frame;
private JTextField textField;
public Inicio_Sesion() {
initialize();
}
private void initialize() {
frame = new JFrame();
frame.setBounds(100, 100, 314, 100);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().setLayout(null);
textField = new JTextField();
textField.setFont(new Font("Tahoma", Font.PLAIN, 14));
textField.setBounds(10, 11, 170, 32);
frame.getContentPane().add(textField);
textField.setColumns(10);
textField.addKeyListener(new KeyListener() {
public void keyPressed(KeyEvent e) {
if(e.getKeyCode()==KeyEvent.VK_ENTER){
if(!textField.getText().equals("")){
Cliente nombre = new Cliente(textField.getText());
frame.dispose();
}else{
JOptionPane.showMessageDialog(null, "Introduce un Nombre");
}
}
}
public void keyReleased(KeyEvent e) {
}
public void keyTyped(KeyEvent e) {
}
});
JButton btnAcceder = new JButton("Acceder");
btnAcceder.setFont(new Font("Tahoma", Font.BOLD, 14));
btnAcceder.setBounds(190, 11, 89, 32);
frame.getContentPane().add(btnAcceder);
btnAcceder.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(!textField.getText().equals("")){
frame.dispose();
}else{
JOptionPane.showMessageDialog(null, "Introduce un Nombre");
}
}
});
}
}
Chat
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
public class Cliente {
ObjectOutputStream salida;
ObjectInputStream entrada;
String texto,server,received;
static String nombre;
Socket socket;
boolean fin = false;
JTextArea textArea;
public JFrame frame;
private JTextField textField;
/**
* Launch the application.
*/
public static void main(String[] args) {
Cliente window = new Cliente(nombre);
window.frame.setVisible(true);
Inicio_Sesion init = new Inicio_Sesion();
init.frame.setVisible(true);
window.ejecutarConexion();
}
/**
* Create the application.
* @param string
*/
public Cliente(String string) {
this.nombre=string;
initialize();
}
/**
* Initialize the contents of the frame.
*/
private void initialize() {
frame = new JFrame();
frame.setBounds(100, 100, 420, 480);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().setLayout(null);
JScrollPane scrollPane = new JScrollPane();
scrollPane.setBounds(10, 11, 384, 366);
frame.getContentPane().add(scrollPane);
textArea = new JTextArea();
textArea.setEnabled(false);
textArea.setEditable(false);
scrollPane.setViewportView(textArea);
textField = new JTextField();
textField.setFont(new Font("Tahoma", Font.PLAIN, 16));
textField.setBounds(10, 388, 280, 42);
frame.getContentPane().add(textField);
textField.setColumns(10);
textField.addKeyListener(new KeyListener() {
public void keyPressed(KeyEvent e) {
if(e.getKeyCode()==KeyEvent.VK_ENTER){
try{
enviarDatos(textField.getText());
}catch(Exception ex){
System.out.println("Error: "+ex.getMessage());
}
textField.setText("");
}
}
public void keyReleased(KeyEvent e) {
}
public void keyTyped(KeyEvent e) {
}
});
JButton btnEnviar = new JButton("Enviar");
btnEnviar.setFont(new Font("Tahoma", Font.BOLD, 14));
btnEnviar.setBounds(298, 388, 96, 42);
frame.getContentPane().add(btnEnviar);
btnEnviar.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try{
enviarDatos(textField.getText());
}catch(Exception ex){
System.out.println("Error: "+ex.getMessage());
}
textField.setText("");
}
});
}
private void ejecutarConexion() {
try{
server = "localhost";
socket = new Socket(server, 5001);
salida = new ObjectOutputStream(socket.getOutputStream());
salida.flush();
entrada = new ObjectInputStream(socket.getInputStream());
textArea.setText("Conexion establecida\n");
//System.out.println("Conexion Establecida\n");
do{
received = (String) entrada.readObject();
textArea.setText(textArea.getText()+" <<<"+ received+"\n");
}while(!fin);
entrada.close();
salida.close();
socket.close();
textArea.setText(textArea.getText()+" Finalizando conexion\n");
}catch (Exception e) {
System.out.println("No se ha podido ejecutar la conexion"+e.getMessage());
}
}
private void enviarDatos(String mensaje){
try{
salida.writeObject(nombre + ": "+mensaje+"\n");
salida.flush();
textArea.setText(textArea.getText() + " >>> " + nombre + ": " + mensaje + "\n");
if(mensaje.equals("fin"))fin=true;
}catch(IOException ex){
System.out.println("\nError al escribir el objeto: " + ex.getMessage());
}
}
}
fin codigo
Bien, con esto me pasa una cosa compleja. Y es que quiero hacer que el inicio de sesión vaya primero y luego al cerrar abra el chat. La ventana principal es la del chat y abre el inicio de sesión para pedir usuario, luego hace dispose(). Intenté que el inicio de sesión fuese principal y chat secundario pero he tenido fallos con la conexión de esta manera.
Ahora lo que quiero que me ayudéis si podeis es en esto, ¿como puedo hacer que inicio de sesión se abra primero y luego abra chat? ¿como podría hacer que el programa sepa si inicio de sesión esta dispose()?¿como ordenarían el código o escribirían el código con sentido?
Valora esta pregunta


0