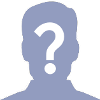
como puedo convertir este arraylist en hashmap
Publicado por Javier (1 intervención) el 16/05/2016 18:44:13
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
package Clases;
import java.io.*;
import java.util.*;
public class RegistroVehiculos {
private static ArrayList<Vehiculo> vehiculo = new ArrayList<Vehiculo>();
//Comprueba si existe el vehiculo en el ArrayList
public static boolean existeVehiculo(Vehiculo objProd){
boolean existe = false;
if (vehiculo.contains(objProd)) existe=true;
else{
for (int i=0;i<vehiculo.size();i++){
if (vehiculo.get(i).getMatricula().equals(objProd.getMatricula())){
existe = true;
break;
}
}
}
if (existe) return true;
else return false;
}
public static void altaVehiculo(Vehiculo objProd) {
vehiculo.add(objProd);
ordenarVehiculo();
}
public static ArrayList<Vehiculo> ordenarVehiculo(){
Comparator MatriProd = new Comparator() {
public int compare(Object o1, Object o2) {
Vehiculo p1 = (Vehiculo) o1;
Vehiculo p2 = (Vehiculo) o2;
return p1.getMatricula().compareTo(p2.getMatricula());
}
};
//Ordenamos el array
Collections.sort(vehiculo, MatriProd);
return vehiculo;
}
//(Metodo no implementado en el programa)
public static void borrarVehiculoPorMatricula(Vehiculo objProd){
for (int i=0;i<vehiculo.size();i++){
if (vehiculo.get(i).getMatricula().equals(objProd.getMatricula())){
vehiculo.remove(i);
break;
}
}
}
public static boolean borrarVehiculo(Vehiculo objProd){
if (vehiculo.contains(objProd)){
vehiculo.remove(objProd);
return true;
} else return false;
}
//Realiza la consulta por matricula
public static int consultaVehiculoPorMatricula(String matricula) {
Comparator MatriProdComp = new Comparator() {
public int compare(Object o1, Object o2) {
Vehiculo p1 = (Vehiculo) o1;
Vehiculo p2 = (Vehiculo) o2;
return p1.getMatricula().compareTo(p2.getMatricula());
}
};
// Ordenamos el array
Collections.sort(vehiculo, MatriProdComp);
//creamos un producto con el codigo a buscar
Vehiculo p = new Vehiculo();
p.setMatricula(matricula);
int pos = Collections.binarySearch(vehiculo, p, MatriProdComp);
return pos;
}
//Realiza la consulta por marca
public static int consultaVehiculoPorMarca(String marca) {
Comparator MarcProdComp = new Comparator() {
public int compare(Object o1, Object o2) {
Vehiculo p1 = (Vehiculo) o1;
Vehiculo p2 = (Vehiculo) o2;
return p1.getMarca().compareTo(p2.getMarca());
}
};
//Ordenamos el array
Collections.sort(vehiculo, MarcProdComp);
//Creamos un producto con el codigo a buscar
Vehiculo p = new Vehiculo();
p.setMarca(marca);
int pos = Collections.binarySearch(vehiculo, p, MarcProdComp);
return pos;
}
//Realiza la consulta por modelo
public static int consultaVehiculoPorModelo(String modelo) {
Comparator ModProdComp = new Comparator() {
public int compare(Object o1, Object o2) {
Vehiculo p1 = (Vehiculo) o1;
Vehiculo p2 = (Vehiculo) o2;
return p1.getModelo().compareTo(p2.getModelo());
}
};
//Ordenamos el array
Collections.sort(vehiculo, ModProdComp);
//Creamos un producto con el codigo a buscar
Vehiculo p = new Vehiculo();
p.setModelo(modelo);
int pos = Collections.binarySearch(vehiculo, p, ModProdComp);
return pos;
}
public static int getNumTotalVehiculo(){
return vehiculo.size();
}
public static Vehiculo getVehiculoPorPosArray(int posicion){
return vehiculo.get(posicion);
}
public static void eliminarVehiculo(int pos) {
vehiculo.remove(pos);
}
//Guarda los datos en un fichero
public static void guardarDatos() {
try {
if (!vehiculo.isEmpty()) {
try (FileOutputStream ostreamProd = new FileOutputStream("Vehiculo.dat")) {
ObjectOutputStream oosProd = new ObjectOutputStream(ostreamProd);
oosProd.writeObject(vehiculo);
}
} else {
System.out.println("Error: No hay datos que guardar...");
}
} catch (IOException ioe) {
System.out.println("Error de IO: " + ioe.getMessage());
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
}
//Carga datos guardados
public static void cargarDatos() {
try {
try (FileInputStream istreamPer = new FileInputStream("Vehiculo.dat")) {
ObjectInputStream oisPer = new ObjectInputStream(istreamPer);
vehiculo = (ArrayList) oisPer.readObject();
}
} catch (IOException ioe) {
System.out.println("Error de IO: " + ioe.getMessage());
} catch (ClassNotFoundException cnfe) {
System.out.println("Error de clase no encontrada: " + cnfe.getMessage());
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
}
}
Valora esta pregunta


0