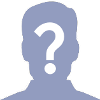
Atascado programa multiplicación para niños
Publicado por Albert (13 intervenciones) el 09/11/2017 09:31:59
Hola buenos días, estoy intentando hacer un programa de multiplicación para niños, se trata de que se pone el número de multiplicaciones que se quiere hacer y el numero máximo a multiplicar, una vez escrito le das a "Practicar" y a la parte de abajo te sale la multiplicación y puedes poner la respuesta y darle a "Comprobar", si es correcto te sale el mensaje bueno, sino te sale incorrecto. El problema es que estoy totalmente atascado en la parte del número de multiplicaciones a hacer, lo he intentado con un do while pero no lo he conseguido, alguien que pueda ayudarme??
Muchas gracias
Muchas gracias
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.events.TouchListener;
import org.eclipse.swt.events.TouchEvent;
import java.util.Random;
public class Calcular {
private static Text txtNmero, txtMxim, textNumMult, textMaxim, numerou, numerodos, resultat, textF, multiplicacio, igual;
private static Button btnComprovar;
/**
* Launch the application.
* @param args
*/
public static void main(String[] args) {
Display display = Display.getDefault();
Shell shell = new Shell();
shell.setSize(385, 400);
shell.setText("SWT Application");
Random r = new Random();
txtNmero = new Text(shell, SWT.NONE);
txtNmero.setEnabled(false);
txtNmero.setEditable(false);
txtNmero.setText("N\u00FAmero multiplicacions");
txtNmero.setBounds(28, 32, 179, 26);
txtMxim = new Text(shell, SWT.NONE);
txtMxim.setEnabled(false);
txtMxim.setEditable(false);
txtMxim.setText("M\u00E0xim");
txtMxim.setBounds(28, 74, 78, 26);
textNumMult = new Text(shell, SWT.BORDER);
textNumMult.setBounds(249, 29, 89, 26);
textMaxim = new Text(shell, SWT.BORDER);
textMaxim.setBounds(249, 71, 89, 26);
numerou = new Text(shell, SWT.BORDER);
numerou.setEnabled(false);
numerou.setEditable(false);
numerou.setBounds(28, 223, 64, 26);
numerodos = new Text(shell, SWT.BORDER);
numerodos.setEnabled(false);
numerodos.setEditable(false);
numerodos.setBounds(138, 223, 69, 26);
resultat = new Text(shell, SWT.BORDER);
resultat.setBounds(249, 223, 89, 26);
resultat.setEnabled(false);
resultat.setEditable(false);
textF = new Text(shell, SWT.BORDER);
textF.setBounds(28, 317, 310, 26);
textF.setEnabled(false);
textF.setEditable(false);
multiplicacio = new Text(shell, SWT.NONE);
multiplicacio.setText("X");
multiplicacio.setEnabled(false);
multiplicacio.setEditable(false);
multiplicacio.setBounds(104, 226, 17, 26);
igual = new Text(shell, SWT.NONE);
igual.setText("=");
igual.setEnabled(false);
igual.setEditable(false);
igual.setBounds(217, 226, 26, 26);
Button Practicar = new Button(shell, SWT.NONE);
Practicar.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
int maxim = 0;
maxim = Integer.parseInt(textMaxim.getText());
textNumMult.setEnabled(false);
textMaxim.setEnabled(false);
int aleatori = r.nextInt(maxim)+1;
numerou.setText(""+aleatori);
aleatori = r.nextInt(maxim)+1;
numerodos.setText(""+aleatori);
resultat.setEnabled(true);
resultat.setEditable(true);
}
});
Practicar.setBounds(249, 114, 78, 30);
Practicar.setText("Practicar");
btnComprovar = new Button(shell, SWT.NONE);
btnComprovar.setBounds(249, 269, 90, 30);
btnComprovar.setText("Comprovar");
btnComprovar.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
int numeroP, numeroS, resultatU, maxim;
maxim = Integer.parseInt(textMaxim.getText());
numeroP = Integer.parseInt(numerou.getText());
numeroS = Integer.parseInt(numerodos.getText());
resultatU = Integer.parseInt(resultat.getText());
if(numeroP * numeroS == resultatU){
textF.setText("Correcte");
} else {
textF.setText("Incorrecte: Torna-ho a provar");
}
int aleatori = r.nextInt(maxim)+1;
numerou.setText(""+aleatori);
aleatori = r.nextInt(maxim)+1;
numerodos.setText(""+aleatori);
}
});
shell.open();
shell.layout();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
}
public int esEnter(Text resultat) {
int numero = 0;
try {
numero = Integer.parseInt(resultat.getText());
} catch (NumberFormatException ex) {
txtNmero.setFocus();
txtNmero.selectAll();
textF.setText("El format introduit no és correcte");
}
return numero;
}
}
Valora esta pregunta


0