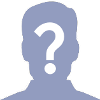
Manejo de Archivos en java
Publicado por Alex (1 intervención) el 01/03/2018 16:20:48
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
package ejercicios.de.prueba;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class EjerciciosDePrueba {
public static void main(String[] args) throws IOException {
// Se declaran las variales con los archivos que se usaran
String precio="Preciosventa";
String vendedor="vendedor";
String venta="ventas";
//variables auxiliares para recuperar valores
int idvendedor=0, productoA=0,precioP=0,act=0, pre=0, id=0;
//se declaran las hash map
Map<Integer, String> MPrecio = new HashMap<Integer, String>();
Map<Integer, String> MVendedor = new HashMap<Integer, String>();
Map<Integer, String> MCompras = new HashMap<Integer, String>();
//se declara la matriz con la que se realizan los calculos
String[][] MVenta = new String[4][5];
//se manda el archivo y se recibe el hashmap
MPrecio=leer(precio);
//se declara un iterador para mostrar el contenido de la hashmap
Iterator it = MPrecio.keySet().iterator();
//ciclo para mostrar el contenido del hashmap
while(it.hasNext()){
Integer key = (Integer) it.next();
System.out.println("Clave: " + key + " -> Valor: " + MPrecio.get(key));
}
//se recibe el hashmap del vendedor
MVendedor=leer(vendedor);
//se muestra el hashmap del vendedor
it = MVendedor.keySet().iterator();
while(it.hasNext()){
Integer key = (Integer) it.next();
System.out.println("Clave: " + key + " -> Valor: " + MVendedor.get(key));
}
//se recibe la matriz con los datos de lo que vendio cada vendedor
MVenta=leer2(venta);
for (int x=0; x < 5; x++) {//ciclo para recorrer la matriz de lo que se vendio
if (MVenta[x][0] != null) {//se valida que en el hash mventa no tenga nullos para poder seguir con los calculos
idvendedor = Integer.parseInt(MVenta[x][0]); //se recupera el id de vendedor del hash mventas
id=idvendedor;//se guarda el dia recuperado
System.out.print(" ID de vendedor " +MVenta[x][0]);
for (int y=0; y < 5; y++) { //ciclo para seguir recorriendo la matriz mventa
//se valida que el valor de la cilumna que sigue no se nulo
if (MVenta[x][y+1] != null) {
//se recupera el identificador del producto actual en la matriz
productoA= Integer.parseInt(MVenta[x][y+1]);
act=productoA;//se guarada el identificador del producto actual
precioP= Integer.parseInt(MPrecio.get(productoA));//se recupera el precio con el identificador antes recuperado
pre=pre+precioP;//se van sumando los precios de los productos
System.out.print(","+act+","+pre);
}//se incertan el hash mcompras el identificador del vendedor junto con el total de lo que vendio
MCompras.put(id, Integer.toString(pre));
}
}//se recetean las variables act (actual) y pre (precio)
act=0;
pre=0;
}
//se declaran los iteradores para mostar el contenido del hash comparas y vendedores
it = MCompras.keySet().iterator();
Iterator itt = MVendedor.keySet().iterator();
// se muetsra en forma de tabla
System.out.println("\n\n\n\nNombre del Vendedor \t Total de Ventas");
while(it.hasNext()){
Integer key = (Integer) it.next();
while(itt.hasNext()){
Integer key2 = (Integer) itt.next();
System.out.println(MVendedor.get(key2)+"\t\t\t" + "->" + MCompras.get(key2));
}
}
}
static String [][] leer2(String ar) throws IOException{
//esta funcion se usa para pasar el archivo de ventas en una matriz con la cual se realizaran los calculos
try {//se lee el archivo
FileReader fr=new FileReader("C:\\Users\\Acer E1\\Documents\\NetBeansProjects\\ejercicios de prueba\\"+ar+".txt");
BufferedReader rArchivo =new BufferedReader(fr);
//se lee la linea del archivo
String linea =rArchivo.readLine();
// System.out.print(linea);
String [][] matriz= new String[20][20]; //se declara la matriz que se regresara
int contador=0;
while(linea != null){//se valida que no esta vacia la lienea
String []valores = linea.split(",");//se separan los valores
for (int i = 0; i < valores.length; i++) {//con el ciclo se llenan los valores en la matriz
matriz[contador][i]= valores[i];
// System.out.print(matriz[contador][i]);
}
// System.out.println("");
contador++;
linea = rArchivo.readLine();//se sigue leyendo el archivo
}
rArchivo.close();
/* for (int i = 0; i < 4; i++) {
for (int j = 0; j < 10; j++) {
System.out.print(matriz[i][j]);
}
System.out.println("");
}*/
return(matriz);//se regresa la matriz
} catch (IOException | NumberFormatException e) {
e.printStackTrace();
}
return null;
}
static HashMap leer(String ar ) throws IOException{
try {//se declara el hashmap para regresar los valores de los arcivos precio y vendedor
Map<Integer, String> Map = new HashMap<Integer, String>();
//se lee el archivo que se recive
FileReader fr=new FileReader("C:\\Users\\Acer E1\\Documents\\NetBeansProjects\\ejercicios de prueba\\"+ar+".txt");
// FileReader fr=new FileReader("C:\\Users\\Acer E1\\Documents\\NetBeansProjects\\ejercicios de prueba\\VENTAS.txt");
BufferedReader rArchivo =new BufferedReader(fr);
//se saca del archivo cada linea
String linea =rArchivo.readLine();
int contador=1;
//se valida que la linea no esta vacia
while(linea != null){//se separan los valores del archivo con el identificador de (,)
String []valores = linea.split(",");
//se realiza un ciclo para vaciar los valores del archivo en el hashmap
for (int i = 0; i < valores.length; i++) {
//se llena el hashmap con los valores
Map.put(contador, valores[i]);
}
contador++;
linea = rArchivo.readLine();//se siguen leyendo las lineas
}
/* Iterator it = MapPrecio.keySet().iterator();
while(it.hasNext()){
Integer key = (Integer) it.next();
System.out.println("Clave: " + key + " -> Valor: " + MapPrecio.get(key));
}*/
rArchivo.close();//se cierra el archivo
return(HashMap) (Map);//se regresa el hashmap con los valores dle archivo txt
} catch (IOException | NumberFormatException e) {
e.printStackTrace();
}
return null;
}
}
Valora esta pregunta


0