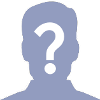
Problema leyendo fichero .bin con java
Publicado por Fulgencio (2 intervenciones) el 30/10/2020 21:39:36
Por qué no lo lee bien? Gracias
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package accesodatos_tarea23;
import java.io.EOFException;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.RandomAccessFile;
/**
*
* @author Usuario
*/
public class AccesoDatos_Tarea23 {
private static Object file;
/**
* @param args the command line arguments
*/
public static void main(String[] args) throws FileNotFoundException, Exception {
// variables
String lenguaje; // Los caracteres tienen 2 bytes (máximo 8 caracteres)
double porcentaje; // Un double coupa 8 bytes.
// Preparamos el string con la ruta:
String carpeta = "C:\\AaDd\\";
String fichero = carpeta + "tiobe.bin";
System.out.println("Empezamos a escribir en el fichero...");
// Comprobamos que exista la ruta de envío y, si no existe, la creamos
File path = new File(carpeta);
if (!path.exists())
path.mkdir();
// Creamos el fichero en modo lectura/escritura. Si ya existiese, se borraría antes
// de crearlo. Mucho OJO si lo que queremos es AÑADIR al fichero y no CREARLO.
RandomAccessFile file = new RandomAccessFile(new File (fichero), "rw");
// Guardamos la informacion
//
// C 15.95
lenguaje = "C";
porcentaje = 15.95;
Guarda_Info(file, lenguaje, porcentaje);
// Java 13.48
lenguaje = "Java";
porcentaje = 13.48;
Guarda_Info(file, lenguaje, porcentaje);
// Python 10.47
lenguaje = "Python";
porcentaje = 10.47;
Guarda_Info(file, lenguaje, porcentaje);
// C++ 7.11
lenguaje = "C++";
porcentaje = 7.11;
Guarda_Info(file, lenguaje, porcentaje);
// C# 4.58
lenguaje = "C#";
porcentaje = 4.58;
Guarda_Info(file, lenguaje, porcentaje);
file.close();
System.out.println("Terminamos con la escritura en el fichero");
System.out.println("Vamos a leer el fichero");
int registros_leidos=0, leidos=0;
//int entero_actual, doble_actual;
// Preparamos el string con la ruta:
//String carpeta = "C:\\AaD\\";
//String fichero_TELEFONOS = carpeta + "telefonos.bin";
// Comprobamos si el fichero existe. Si no es así, podemos terminar:
File existe_Fichero = new File (fichero);
if (!existe_Fichero.exists())
{
System.out.println("ERROR: El fichero " + fichero + " no existe.");
System.exit(0);
}
// Como estamos aquí es que no nos hemos escapado en el exit (). Eso
// quiere decir que el fichero SÍ existe. Para complicarnos la vida
// vamos a hacer la lectura en 3 etapas diferentes. En cada una
// de ellas tendremos que abrir, leer y cerrar.
// ATENCIÓN: No queremos leer siempre la misma secuencia. Como lo
// vamos a hacer en 3 etapas, tiene que suceder que en la 1ª etapa
// se lean ciertos registros, en la 2ª etapa se lean los siguientes y
// en la 3ª etapa se lean los últimos. Eso nos obliga a que nosotros
// llevemos la cuenta. Recordemos que no tenemos una BBDD que nos
// facilita la tarea.
System.out.println("Empezamos la lectura.");
// Etapa 1: Vamos a leer sólo 1 registro:
leidos = Lee_Registros (fichero, 1, 0); // De momento no hemos leído ninguno
registros_leidos += leidos;
// Etapa 2: Vamos a leer 2 registros:
leidos = Lee_Registros (fichero, 2, registros_leidos);
registros_leidos += leidos;
// Etapa 3: Vamos a leer 2 registros:
leidos = Lee_Registros (fichero, 2, registros_leidos);
registros_leidos += leidos;
System.out.println("Se han leído: " + registros_leidos + " registros.");
}//fin del main
private static void Guarda_Info (RandomAccessFile file, String lenguaje, double porcentaje) throws Exception
{
// F.writeBytes(L);
// F.writeDouble(P);
file.writeBytes(lenguaje);
file.writeDouble(porcentaje);
}
private static int Lee_Registros (String Nombre_Fichero, int registros_a_leer, int ya_leidos) throws Exception
{
//int numero_telefono, cuantos;
//double importe_factura;
Byte leng;
Double porc;
int cuantos;
// Abrimos el archivo en modo lectura, Sabemos que SÍ existe:
try (RandomAccessFile fichero = new RandomAccessFile(Nombre_Fichero, "r"))
{
// ATENCIÓN: Con el argumento "ya_leidos" nos están proporcionando cuantos registros se han
// leido hasta ahora en las anteriores invocaciones a esta función.
// Vamos a intentar leer el número de registros indicado en la variable registros_a_leer.
// Primero deberemos situar el puntero de lectura en el sitio preciso para evitar leer
// los registros desde el principio. Recordemos que "ya hemos leido" ciertos registros.
// Si no hiciéramos esto siempre empezaríamos a leer desde el principio:
fichero.seek (ya_leidos * 12); // Cada registro tiene 12 bytes: 4 + 8
cuantos=0;
for (int n=0; n<registros_a_leer; n++)
{
leng=fichero.readByte();
porc=fichero.readDouble();
// numero_telefono = fichero.readChar();
// importe_factura = fichero.readDouble();
cuantos++;
System.out.println("Lenguaje: " +leng + " Porcentaje: " + porc);
}
// Lo cerramos:
fichero.close();
}
// Devolvemos cuántos registros hemos leído:
return (cuantos);
}
}
Valora esta pregunta


0