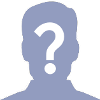
Volver a iterar
Publicado por Pedro (7 intervenciones) el 22/09/2022 09:39:30
Buenas, tengo el siguiente codigo, y quiero que, cada vez que insertas dos numeros y realizas una opercion, la consola te pregunte si quieres seguir operando o no. En caso de que si, te vuelve a pedir dos numeros, y en casod e que no pues nada se para la ejecución. He pensado que se tiene que hacer con un while o un if pero no se donde insertarlo para que funcione, ya que he probado varias cosas y he dejado la función en un bucle infinito
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
package ejercicios;
import java.util.InputMismatchException;
import java.util.Scanner;
/**
*
* @author Pedro Ruiz
*
*/
public class Input {
public static int promptInt( Scanner sc, String prompt )
{
System.out.print( prompt );
while( ! sc.hasNextInt() )
{
System.out.println( "Invalid input, must be an integer" );
System.out.print( prompt );
sc.next(); //if the input is not a number throw away invalid input
}
return sc.nextInt();
}
public static void operations(){
try {
Integer number1;
Integer number2;
char operator;
Scanner sc = new Scanner(System.in);
number1 = promptInt( sc, "Give me number 1: " );//Here we ask for the numbers
number2 = promptInt( sc, "Give me number 2: " );
System.out.println("Choose between +, /, - or *");
operator = sc.next().charAt(0);
int result = 0;
switch(operator) {//With this switch we go through the operations
case '+':
result = number1 + number2;
break;
case '-':
result = number1 - number2;
break;
case '*':
result = number1 * number2;
break;
case '/':
result = number1 / number2;
if(number1 == 0 || number2 == 0 ) {
System.out.println("Don't divide by 0, please");//If we try to divide by 0 we get an error
}
break;
default:
System.out.println("We don't contemplate that operation");//If we pass something different from +,* or / it gives an error
}
System.out.println("The result of the " + " operation is " + result );
if(operator == '+' || operator == '/' || operator == '*' ) { //This would be the way to ask if you want to try again
System.out.println("Do you want to try another operation?");
}
}catch (InputMismatchException e) {
System.out.println("You are not inserting a number, try again");}
}
public static void main(String[] args) {
operations();
}
}
Valora esta pregunta


0