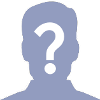
Ayuda con SOAP y recogida de ARRAY de WEBSERVER
Publicado por Javier (9 intervenciones) el 11/05/2017 20:12:55
Buenas haber si me podéis ayudar, el tema es que tengo un problema con la app que estoy desarrollando, ago la peticion y todo correcto y me trae el array pero no me rellena la lista, os voy a adjuntar mis codigos:
Cliente.java
activity_main.xml
aver si podeis ayudarme, Gracias
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
MainActivity.java
package com.example.ki11z0n3.clientes;
import android.app.Activity;
import android.os.AsyncTask;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.ListView;
import android.widget.TextView;
import net.sgoliver.android.soap.R;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
public class MainActivity extends Activity {
private TextView txtResultado;
private Button btnConsultar;
private ListView lstClientes;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
txtResultado = (TextView)findViewById(R.id.txtResultado);
btnConsultar = (Button)findViewById(R.id.btnConsultar);
lstClientes = (ListView)findViewById(R.id.lstClientes);
btnConsultar.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
TareaWSConsulta tarea = new TareaWSConsulta();
tarea.execute();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
//Tarea Asíncrona para llamar al WS de consulta en segundo plano
private class TareaWSConsulta extends AsyncTask<String,Integer,Boolean> {
private Cliente[] listaClientes;
protected Boolean doInBackground(String... params) {
boolean resul = true;
String NAMESPACE = "http://datagram.es/";
String URL="http://develop.datagram.es/wsDTG/Service.asmx";
String METHOD_NAME = "getClientes";
String SOAP_ACTION = "http://datagram.es/getClientes";
String token = "VqE9OGko-l-9IKS7oToJxR<>";
SoapObject request = new SoapObject(NAMESPACE, METHOD_NAME);
request.addProperty("_token", token);
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(SoapEnvelope.VER11);
envelope.dotNet = true;
envelope.setOutputSoapObject(request);
HttpTransportSE transporte = new HttpTransportSE(URL);
try
{
transporte.call(SOAP_ACTION, envelope);
SoapObject resSoap =(SoapObject)envelope.bodyIn;
Cliente[] listaClientes = new Cliente[resSoap.getPropertyCount()];
for (int i = 0; i < listaClientes.length; i++)
{
SoapObject ic = (SoapObject)resSoap.getProperty(i);
Cliente cli = new Cliente();
cli.id = Integer.parseInt(ic.getProperty(0).toString());
cli.nombre = ic.getProperty(1).toString();
listaClientes[i] = cli;
}
}
catch (Exception e)
{
resul = false;
}
return resul;
}
protected void onPostExecute(Boolean result) {
if (result)
{
//Rellenamos la lista con los nombres de los clientes
final String[] datos = new String[listaClientes.length];
for(int i=0; i<listaClientes.length; i++)
datos[i] = listaClientes[i].nombre;
ArrayAdapter<String> adaptador =
new ArrayAdapter<String>(MainActivity.this,
android.R.layout.simple_list_item_1, datos);
lstClientes.setAdapter(adaptador);
}
else
{
txtResultado.setText("Error!");
}
}
}
}
Cliente.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
package com.example.ki11z0n3.clientes;
import org.ksoap2.serialization.KvmSerializable;
import org.ksoap2.serialization.PropertyInfo;
import java.util.Hashtable;
public class Cliente implements KvmSerializable {
public int id;
public String nombre;
public Cliente()
{
id = 0;
nombre = "";
}
public Cliente(int id, String nombre)
{
this.id = id;
this.nombre = nombre;
}
@Override
public Object getProperty(int arg0) {
switch(arg0)
{
case 0:
return id;
case 1:
return nombre;
}
return null;
}
@Override
public int getPropertyCount() {
return 2;
}
@Override
public void getPropertyInfo(int ind, Hashtable ht, PropertyInfo info) {
switch(ind)
{
case 0:
info.type = PropertyInfo.INTEGER_CLASS;
info.name = "ID";
break;
case 1:
info.type = PropertyInfo.STRING_CLASS;
info.name = "ENTIDAD";
break;
default:break;
}
}
@Override
public void setProperty(int ind, Object val) {
switch(ind)
{
case 0:
id = Integer.parseInt(val.toString());
break;
case 1:
nombre = val.toString();
break;
default:
break;
}
}
}
activity_main.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:orientation="vertical"
tools:context=".MainActivity" >
<LinearLayout
android:id="@+id/linearLayout1"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout2"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout3"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<Button
android:id="@+id/btnConsultar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/consultar" />
<TextView
android:id="@+id/txtResultado"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="" />
</LinearLayout>
<ListView
android:id="@+id/lstClientes"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
</ListView>
</LinearLayout>
aver si podeis ayudarme, Gracias
Valora esta pregunta


0