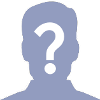
Buzon
Publicado por M2 (5 intervenciones) el 28/02/2013 17:24:04
Buenas tardes necesito ayuda con este ejercicio de programacion concurrente, no se exactamente como hacerlo os pongo lo que yo he pensado, muchas gracias de todas formas.
Buffer con capacidad para 4 mensajes. Un escritor solamente tendría que esperar, cuando el Buffer tenga 4 mensajes sin leer. De la misma forma, un lector solo esperará si el buffer está vacío. Los mensajes deben leerse en el mismo orden en que se han ido enviando al Buffer.
public class Buffer {
private String[] buf = new String[4];
private int numMensajes=0;
public synchronized void enviaMensaje(String msg) throws InterruptedException{
while(numMensajes==4){
wait();
}
buf[numMensajes]=msg;
numMensajes++;
notifyAll();
}
public synchronized String recibeMensaje() throws InterruptedException{
while(numMensajes==0){
wait();
}
numMensajes++;
notifyAll();
return buf[numMensajes];
}
}
import java.util.logging.Level;
import java.util.logging.Logger;
public class Escritor extends Thread {
private String prefijo;
private int numMensajes;
private Buffer miBuzon;
public Escritor(String prefijo, int n, Buffer buzon){
this.prefijo=prefijo;
numMensajes=n;
miBuzon=buzon;
start();
}
public void run(){
for(int i=1; i<=numMensajes; i++){
try{ sleep((int)(500+500*Math.random()));} catch(InterruptedException e){}
try {
miBuzon.enviaMensaje(prefijo+i);
} catch (InterruptedException ex) {
Logger.getLogger(Escritor.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
}
import java.util.logging.Level;
import java.util.logging.Logger;
public class Lector extends Thread {
private int numMensajes;
private Buffer miBuzon;
public Lector(int numMensajes, Buffer miBuzon){
this.numMensajes=numMensajes;
this.miBuzon=miBuzon;
start();
}
@Override
public void run(){
for(int i=0; i<numMensajes; i++){
try{ sleep((int)(500+500*Math.random()));} catch(InterruptedException e){}
try {
System.out.println(miBuzon.recibeMensaje());
} catch (InterruptedException ex) {
Logger.getLogger(Lector.class.getName()).log(Level.SEVERE, null, ex);
}
}
System.out.println("Total mensajes leidos: "+numMensajes);
}
}
public class PruebaBuzon1 {
//Un lector no puede leer un mensaje dos veces
public static void main(String[] s){
Buffer buzonX = new Buffer();
Escritor pedro = new Escritor("Pedro ",5,buzonX);
Escritor juan = new Escritor("Juan ",4,buzonX);
Escritor antonio = new Escritor("Antonio ",6,buzonX);
Escritor luis = new Escritor("Luis ",7,buzonX);
Lector pepe = new Lector(1,buzonX);
Lector jose = new Lector(21,buzonX);
}
}
Buffer con capacidad para 4 mensajes. Un escritor solamente tendría que esperar, cuando el Buffer tenga 4 mensajes sin leer. De la misma forma, un lector solo esperará si el buffer está vacío. Los mensajes deben leerse en el mismo orden en que se han ido enviando al Buffer.
public class Buffer {
private String[] buf = new String[4];
private int numMensajes=0;
public synchronized void enviaMensaje(String msg) throws InterruptedException{
while(numMensajes==4){
wait();
}
buf[numMensajes]=msg;
numMensajes++;
notifyAll();
}
public synchronized String recibeMensaje() throws InterruptedException{
while(numMensajes==0){
wait();
}
numMensajes++;
notifyAll();
return buf[numMensajes];
}
}
import java.util.logging.Level;
import java.util.logging.Logger;
public class Escritor extends Thread {
private String prefijo;
private int numMensajes;
private Buffer miBuzon;
public Escritor(String prefijo, int n, Buffer buzon){
this.prefijo=prefijo;
numMensajes=n;
miBuzon=buzon;
start();
}
public void run(){
for(int i=1; i<=numMensajes; i++){
try{ sleep((int)(500+500*Math.random()));} catch(InterruptedException e){}
try {
miBuzon.enviaMensaje(prefijo+i);
} catch (InterruptedException ex) {
Logger.getLogger(Escritor.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
}
import java.util.logging.Level;
import java.util.logging.Logger;
public class Lector extends Thread {
private int numMensajes;
private Buffer miBuzon;
public Lector(int numMensajes, Buffer miBuzon){
this.numMensajes=numMensajes;
this.miBuzon=miBuzon;
start();
}
@Override
public void run(){
for(int i=0; i<numMensajes; i++){
try{ sleep((int)(500+500*Math.random()));} catch(InterruptedException e){}
try {
System.out.println(miBuzon.recibeMensaje());
} catch (InterruptedException ex) {
Logger.getLogger(Lector.class.getName()).log(Level.SEVERE, null, ex);
}
}
System.out.println("Total mensajes leidos: "+numMensajes);
}
}
public class PruebaBuzon1 {
//Un lector no puede leer un mensaje dos veces
public static void main(String[] s){
Buffer buzonX = new Buffer();
Escritor pedro = new Escritor("Pedro ",5,buzonX);
Escritor juan = new Escritor("Juan ",4,buzonX);
Escritor antonio = new Escritor("Antonio ",6,buzonX);
Escritor luis = new Escritor("Luis ",7,buzonX);
Lector pepe = new Lector(1,buzonX);
Lector jose = new Lector(21,buzonX);
}
}
Valora esta pregunta


0