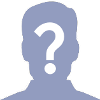
Random Access File
Publicado por Wrath (1 intervención) el 02/04/2014 07:52:15
Saludos. Estoy iniciando en los archivos de acceso aleatorio y tengo un pequeño problema en un ejercicio, lo que pasa es que al imprimir la información del archivo.dat en un jTextArea me presenta algo como !@#$$# 0 y 0.0. Si alguien puede ayudarme de antemano gracias.
INGRESO
LECTURA por codigo del registros
INGRESO
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
int cantidad = 1;
cantidad += cb_cant.getSelectedIndex();
double precio = Double.parseDouble(cb_precio.getText());
String cod = tf_cod.getText();
String des = tf_des.getText();
String prov = tf_pro.getText();
if(cod.length() < 10)
for(int i = cod.length(); i < 10; i++)
cod += "."; // completar los 10 caracteres
if(des.length() < 15)
for(int i = des.length(); i < 15; i++)
des += "."; // completar los 15 caracteres
if(prov.length() < 10)
for(int i = prov.length(); i < 10; i++)
prov += "."; // completar los 10 caracteres
try{
RandomAccessFile ventas = new RandomAccessFile("productos.dat","rw");
ventas.writeChars(cod + des + prov);
ventas.writeInt(cantidad);
ventas.writeDouble(precio);
ventas.close();
}
catch(FileNotFoundException e){
System.out.println(e.getMessage());
}
catch(IOException e){
System.out.println(e.getMessage());
}
LECTURA por codigo del registros
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
String codigo = tf_cod.getText();
char cod[] = new char [10];
char des[] = new char [15];
char prov[] = new char [10];
int cant = 0;
double precio = 0;
try {
RandomAccessFile archivo = new RandomAccessFile("productos.dat","r");
archivo.seek(0); //nos situamos al principio
for(int i = 0; i < archivo.length(); i += 82){
for(int j = 0; j < 10; j ++)
cod[j] = archivo.readChar();
if(tfcod.getText().equals(cod)){
archivo.seek(i);
for(int k = 0; k < 10; k ++)
cod[k] = archivo.readChar();
archivo.skipBytes(20);
for(int l = 0; l < 15; l ++)
des[l] = archivo.readChar();
archivo.skipBytes(50);
for(int m = 0; m < 10; m ++)
prov[m] = archivo.readChar();
archivo.skipBytes(70);
cant = archivo.readInt();
archivo.skipBytes(74);
precio = archivo.readDouble();
}
archivo.close();
ta_ consulta.append(cod + "\n" + des + "\n" + prov + "\n" + cant + "\n" + precio);
}
} catch (FileNotFoundException e) {
System.out.println("Fin de fichero");
} catch (IOException ex) {
System.out.println(ex.getMessage());
}
Valora esta pregunta


0