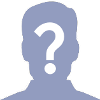
obtener datos de dos tablas y hacer insert en Hibernate
Publicado por kevin (2 intervenciones) el 27/01/2017 15:31:39
hola hace una semana estoy utilizando Hibernate pero el problema es cuando tengo dos tablas relacionadas
pues aqui dejo el codigo. ps cualquier ayuda podria su provechoza para mi
pues aqui dejo el codigo. ps cualquier ayuda podria su provechoza para mi
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
public class Lista implements java.io.Serializable {
private Integer idLista;
private String miLista;
private Cuadro cuadro;
public Lista() {
}
public Lista(Cuadro cuadro, String miLista) {
this.cuadro = cuadro;
this.miLista = miLista;
}
public Integer getIdLista() {
return this.idLista;
}
public void setIdLista(Integer idLista) {
this.idLista = idLista;
}
public Cuadro getCuadro() {
return this.cuadro;
}
public void setCuadro(Cuadro cuadro) {
this.cuadro = cuadro;
}
public String getMiLista() {
return this.miLista;
}
public void setMiLista(String miLista) {
this.miLista = miLista;
}
// cuadro
public class Cuadro implements java.io.Serializable {
private Integer idCuadro;
private String titulo;
private String imagen;
private Set listas = new HashSet(0);
public Cuadro() {
}
public Cuadro(String titulo, String imagen) {
this.titulo = titulo;
this.imagen = imagen;
}
public Cuadro(String titulo, String imagen, Set listas) {
this.titulo = titulo;
this.imagen = imagen;
this.listas = listas;
}
public Integer getIdCuadro() {
return this.idCuadro;
}
public void setIdCuadro(Integer idCuadro) {
this.idCuadro = idCuadro;
}
public String getTitulo() {
return this.titulo;
}
public void setTitulo(String titulo) {
this.titulo = titulo;
}
public String getImagen() {
return this.imagen;
}
public void setImagen(String imagen) {
this.imagen = imagen;
}
public Set getListas() {
return this.listas;
}
public void setListas(Set listas) {
this.listas = listas;
}
//servlet lista
public class Servlet_Lista extends HttpServlet {
/**
* Processes requests for both HTTP <code>GET</code> and <code>POST</code>
* methods.
*
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
protected void processRequest(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
try (PrintWriter out = response.getWriter()) {
Datos dato= new Datos();
Cuadro cuadro= new Cuadro(request.getParameter("titulo"),request.getParameter("imagen"));
Lista lista= new Lista(cuadro,request.getParameter("miLista"));
dato.guardar(lista);
/*Constructor JDBC
dato.ejecutar("Insert into lista (mi_lista, cu_id_fk)"
+ " values ('"+request.getParameter("mi_lista")+"',"
+ " '"+request.getParameter("cu_id_fk")+"')");*/
response.sendRedirect("administrador.jsp");
}
}
//servlet cuadro
public class Servlet_Cuadro extends HttpServlet {
protected void processRequest(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
try (PrintWriter out = response.getWriter()) {
Datos dato= new Datos();
boolean guardar=false;
boolean modificar=false;
if(request.getParameter("guardar")!=null){
guardar=true;
}
if(request.getParameter("modificar")!=null){
modificar=true;
}
if(guardar){
Cuadro cuadro= new Cuadro (request.getParameter("titulo"),request.getParameter("imagen"));
dato.guardar(cuadro);
}
/*metodo de insertar con constructor JDBC
dato.ejecutar("Insert into cuadro (titulo, imagen)"
+ " values ('"+request.getParameter("titulo")+"',"
+ " '"+request.getParameter("imagen")+"')");
}
*/
if(modificar){
dato.ejecutar("UPDATE cuadro SET "
+ "titulo='"+request.getParameter("titulo")+"',"
+ "imagen='"+request.getParameter("imagen")+"'");
}
response.sendRedirect("administrador.jsp");
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
//xml lista
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<!-- Generated 13/01/2017 04:51:32 PM by Hibernate Tools 4.3.1 -->
<hibernate-mapping>
<class name="modelo.Lista" table="lista" catalog="mi_paginadb" optimistic-lock="version">
<id name="idLista" type="java.lang.Integer">
<column name="id_lista" />
<generator class="identity" />
</id>
<many-to-one name="cuadro" class="modelo.Cuadro" fetch="select">
<column name="cu_id_fk" not-null="true" />
</many-to-one>
<property name="miLista" type="string">
<column name="mi_lista" length="45" not-null="true" />
</property>
</class>
</hibernate-mapping>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
//xmlk cuadro
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<!-- Generated 13/01/2017 04:51:32 PM by Hibernate Tools 4.3.1 -->
<hibernate-mapping>
<class name="modelo.Cuadro" table="cuadro" catalog="mi_paginadb" optimistic-lock="version">
<id name="idCuadro" type="java.lang.Integer">
<column name="id_cuadro" />
<generator class="identity" />
</id>
<property name="titulo" type="string">
<column name="titulo" length="45" not-null="true" />
</property>
<property name="imagen" type="string">
<column name="imagen" length="65535" not-null="true" />
</property>
<set name="listas" table="lista" inverse="true" lazy="true" fetch="select">
<key>
<column name="cu_id_fk" not-null="true" />
</key>
<one-to-many class="modelo.Lista" />
</set>
</class>
</hibernate-mapping>
Valora esta pregunta


0