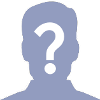
duda acceso a ficheros
Publicado por Fulgencio (2 intervenciones) el 25/10/2020 21:46:56
Hola, tengo el siguiente código y no sé si es correcto, me ayudais?
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
/*
1. Crear un fichero
2. Crear un directorio
3. Pedir fichero (si no existe, crearlo) y escribir algo.
4. Pedir fichero (indicar si no existe) y leer su contenido
5. Pedir dos ficheros y copiar el contenido del primero en el segundo.
6. Contar el número de vocales de un fichero dado.
7. Salir
*/
package accesodatos_tarea2_1;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Scanner;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
*
* @author Usuario
*/
public class AccesoDatos_Tarea2_1 {
/**
* @param args the command line arguments
*/
public static void main(String[] args) throws IOException {
// TODO code application logic here
Scanner sn=new Scanner(System.in);
boolean salir=false;
int opcion;
while(!salir){
System.out.println("1.- Crear fichero.");
System.out.println("2.- Crear directorio.");
System.out.println("3.- Pedir fichero (si no existe, crearlo) y escribir algo.");
System.out.println("4.- Pedir fichero (indicar si no existe) y leer su contenido.");
System.out.println("5.- Pedir dos ficheros y copiar el contenido del primero en el segundo.");
System.out.println("6.- Contar el número de vocales de un fichero dado.");
System.out.println("7.- Salir.");
System.out.println("Escribe una opcion: ");
opcion=sn.nextInt();
switch(opcion){
case 1:
System.out.println("Has elegido la opcion 1.- Crear fichero.");
{
try {
FileWriter fw=new FileWriter("C:/Users/Usuario/Desktop/mifichero2.txt",true);
} catch (IOException ex) {
Logger.getLogger(AccesoDatos_Tarea2_1.class.getName()).log(Level.SEVERE, null, ex);
}
}
break;
case 2:
System.out.println("Has elegido la opcion 2.- Crear directorio.");
File carpeta=new File("C:/Users/Usuario/Desktop/yo2");
carpeta.mkdir();
break;
case 3:
System.out.println("Has elegido la opcion 3.- Pedir fichero (si no existe, crearlo) y escribir algo.");
String fichero3pedir;
fichero3pedir=sn.next();
File archivo = new File("C:\\Users\\Usuario\\Desktop\\"+fichero3pedir+".txt");
if (!archivo.exists()) {
System.out.println("OJO: ¡¡No existe el archivo. Lo creo y escribo mi nombre");
FileWriter fw=new FileWriter(archivo,true);
fw.write("Fulgencio");
fw.flush();
fw.close();
}
else{
System.out.println("SI que existe el fichero y escribo mi nombre.");
FileWriter fw=new FileWriter(archivo,true);
fw.write("Fulgencio");
fw.flush();
fw.close();
}
break;
case 4:
System.out.println("Has elegido la opcion 4.- Pedir fichero (indicar si no existe) y leer su contenido.");
String fichero4pedir;
fichero4pedir=sn.next();
File archivo4 = new File("C:\\Users\\Usuario\\Desktop\\"+fichero4pedir+".txt");
if (!archivo4.exists()) {
System.out.println("OJO: ¡¡No existe el archivo.");
}else{
String cadena;
FileReader f = new FileReader(archivo4);
BufferedReader b = new BufferedReader(f);
while((cadena = b.readLine())!=null) {
System.out.println(cadena);
}
b.close();
}
break;
case 5:
System.out.println("Has elegido la opcion 5.- Pedir dos ficheros y copiar el contenido del primero en el segundo.");
String ficheroOrigen;
String ficheroDestino;
ficheroOrigen=sn.next();
ficheroDestino=sn.next();
File origen = new File("C:\\Users\\Usuario\\Desktop\\"+ficheroOrigen+".txt");
File destino = new File("C:\\Users\\Usuario\\Desktop\\"+ficheroDestino+".txt");
FileInputStream in = new FileInputStream(origen);
FileOutputStream out = new FileOutputStream(destino);
int c;
while( (c = in.read() ) != -1)
out.write(c);
break;
case 6:
System.out.println("Has elegido la opcion 6.- Contar el número de vocales de un fichero dado.");
String ficheroContar;
int contador=0;
ficheroContar=sn.next();
File contar=new File("C:\\Users\\Usuario\\Desktop\\"+ficheroContar+".txt");
FileReader fr = new FileReader("C:\\Users\\Usuario\\Desktop\\"+ficheroContar+".txt");
int caract=fr.read();
while(caract!=-1){
contador++;
caract=fr.read();
}
System.out.println("El fichero "+ficheroContar+".txt tiene "+contador+" caracteres.");
case 7:
salir=true;
break;
default:
System.out.println("Solo opciones del 1 al 7");
}//fin switch
}//fin while
}
}
Valora esta pregunta


0