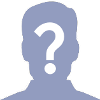
ERROR - Parametrización del constructor fallida
Publicado por Xavi (21 intervenciones) el 06/11/2021 18:36:08
Soy nuevo en Java. Estoy intentando realizar intentando replicar el diagrama UML adjunto en Java.
Como se puede apreciar se trata de una asociación unidireccional de composición, con la clase Team como clase compuesta y la clase StatisticsTeam como componente, de tal forma que si desaparece Team también desaparece el objeto StatisticsTeam vinculado. Los métodos/setters/getters y requerimientos son los siguientes:
- El constructor debe inicializar todos los atributos con el valor por defecto indicado en el diagrama UML.
- Al crear un objeto de tipo Team se tiene que crear un objeto StatisticsTeam que le corresponda.
- Siempre que exista conflicto entre nombres, sobretodo en los métodos setter, se tiene que usar la palabra reservada this.
- Si el nombre que se asigna al equipo tiene más de 50 carácteres, entonces hay que lanzar Exception.
- Si el NIF que se quiere asignar al equipo no cumple con el patrón establecido, entonces setNif no tiene que asignar ese valor al equipo y en su lugar lanzar una Exception. El patrón es: nombre que empiece por A, B o G en mayúsculas seguido de 8 dígitos.
- El mail que se quiere asignar al equipo tiene que contener una @ y acabar en .es o com.
- El método resetStatistics() tiene que instanciar un nuevo objeto StatisticsTeam.
- El valor de la capacidad tiene que ser superior a 0.
- El método getInfo tiene que devolver un texto con el siguiente formato: Name: N - Foundation year: Y - NIF: F. Siendo N el nombre del equipo; Y el año de fundación; F el NIF.
He codificado la clase Team de la siguiente manera:
Para comprobar que se está haciendo bien, se han realizado los siguientes test:
Sin embargo, algo estoy haciendo mal porqué devuelvo Parametrized constructor failed, es decir la Exception del void init() salta.
Como se puede apreciar se trata de una asociación unidireccional de composición, con la clase Team como clase compuesta y la clase StatisticsTeam como componente, de tal forma que si desaparece Team también desaparece el objeto StatisticsTeam vinculado. Los métodos/setters/getters y requerimientos son los siguientes:
- El constructor debe inicializar todos los atributos con el valor por defecto indicado en el diagrama UML.
- Al crear un objeto de tipo Team se tiene que crear un objeto StatisticsTeam que le corresponda.
- Siempre que exista conflicto entre nombres, sobretodo en los métodos setter, se tiene que usar la palabra reservada this.
- Si el nombre que se asigna al equipo tiene más de 50 carácteres, entonces hay que lanzar Exception.
- Si el NIF que se quiere asignar al equipo no cumple con el patrón establecido, entonces setNif no tiene que asignar ese valor al equipo y en su lugar lanzar una Exception. El patrón es: nombre que empiece por A, B o G en mayúsculas seguido de 8 dígitos.
- El mail que se quiere asignar al equipo tiene que contener una @ y acabar en .es o com.
- El método resetStatistics() tiene que instanciar un nuevo objeto StatisticsTeam.
- El valor de la capacidad tiene que ser superior a 0.
- El método getInfo tiene que devolver un texto con el siguiente formato: Name: N - Foundation year: Y - NIF: F. Siendo N el nombre del equipo; Y el año de fundación; F el NIF.
He codificado la clase Team de la siguiente manera:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
package edu.uoc.pac3;
public class Team {
private int id;
private static int nextId = 0;
private String name;
private String foundationYear;
private String nif;
private String email;
private int capacity;
private StatisticsTeam statistics;
public Team() throws Exception {
this.setId();
this.setName("Lorem club");
this.setFoundationYear("2000");
this.setNif("G12345678");
this.setEmail("info@yourmail.com");
this.setCapacity(21);
this.getStatisticsTeam();
}
public Team(String name, String foundationYear, String nif, String email, int capacity) throws Exception {
this.setId();
this.setName(name);
this.setFoundationYear(foundationYear);
this.setNif(nif);
this.setEmail(email);
this.setCapacity(capacity);
this.getStatisticsTeam();
}
public int getId() {
return this.id;
}
private void setId() {
this.id = getNextId();
this.incNextId();
}
public static int getNextId() {
return nextId;
}
private void incNextId() {
++nextId;
}
public String getName() {
return this.name;
}
public void setName(String name) throws Exception {
if (name.length() > 50) {
throw new Exception("[ERROR] Team's name cannot be longer than 50 characters");
} else {
this.name = name;
}
}
public String getNif() {
return this.nif;
}
public void setNif(String nif) throws Exception {
String pattern;
pattern = "^[ABC]\\d{8}";
if (nif.matches(pattern)){
this.nif = nif;
} else {
throw new Exception("[ERROR] Team's NIF pattern is incorrect");
}
}
public String getEmail() {
return this.email;
}
public void setEmail(String email) throws Exception {
String pattern1 = "^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.+-]+\\.es$";
String pattern2 = "^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.+-]+\\.com$";
if (email.matches(pattern1) || email.matches(pattern2)) {
this.email = email;
} else {
throw new Exception("[ERROR] Team's email pattern is incorrect");
}
}
public int getCapacity() {
return this.capacity;
}
public void setCapacity(int capacity) throws Exception{
if (capacity <= 0) {
throw new Exception("[ERROR Team's capacity must be greater than 0]");
} else {
this.capacity = capacity;
}
}
public String getFoundationYear() {
return this.foundationYear;
}
public void setFoundationYear(String foundationYear) {
this.foundationYear = foundationYear;
}
public String getInfo() {
String text;
text = "Name:" + this.name + "- Foundation year:" + this.foundationYear + "- NIF:" + this.nif ;
return text;
}
public StatisticsTeam getStatisticsTeam() {
this.statistics = resetStatistics();
return this.statistics;
}
public StatisticsTeam resetStatistics() {
try {
this.statistics = new StatisticsTeam();
} catch (Exception e) {
e.printStackTrace();
}
return this.statistics;
}
}
Para comprobar que se está haciendo bien, se han realizado los siguientes test:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
package edu.uoc.pac3;
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.*;
import org.junit.jupiter.api.MethodOrderer.OrderAnnotation;
import org.junit.jupiter.api.TestInstance.Lifecycle;
import java.lang.reflect.Field;
@TestInstance(Lifecycle.PER_CLASS)
@TestMethodOrder(OrderAnnotation.class)
class IntegrationTest {
Team team;
@BeforeAll
void init() {
try{
Field field = Team.class.getDeclaredField("nextId");
field.setAccessible(true);
field.set(null, 0);
team = new Team("Futbol Club Barcelona", "1899", "G08266298", "sac@fcbarcelona.com", 25);
}catch(Exception e) {
fail("Parameterized constructor failed");
}
}
@Test
@Order(1)
void testTeamDefault() {
try{
Team t = new Team();
assertEquals(1, t.getId());
assertEquals("Lorem club", t.getName());
assertEquals("2000", t.getFoundationYear());
assertEquals("G12345678", t.getNif());
assertEquals("info@yourmail.com", t.getEmail());
assertEquals(21, t.getCapacity());
assertNotNull(t.getStatisticsTeam());
assertEquals(0, team.getStatisticsTeam().getWon());
assertEquals(0, team.getStatisticsTeam().getDrawn());
assertEquals(0, team.getStatisticsTeam().getLost());
assertEquals(0, team.getStatisticsTeam().getGoalsFor());
assertEquals(0, team.getStatisticsTeam().getGoalsAgainst());
assertEquals(0, team.getStatisticsTeam().getGamesPlayed());
assertEquals(0, team.getStatisticsTeam().getGoalsDifference());
assertEquals(0, team.getStatisticsTeam().getPoints());
}catch(Exception e) {
fail("Default constructor failed");
}
}}
Sin embargo, algo estoy haciendo mal porqué devuelvo Parametrized constructor failed, es decir la Exception del void init() salta.
- UML.zip(66,9 KB)
Valora esta pregunta


0