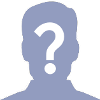
proyecto señor de los anillos
Publicado por Gianluca (1 intervención) el 25/04/2023 18:39:12
buenas !! estoy un proyecto para un master en java, es un juego del señor de los anillos, estoy teniendo problemas con implementarle las imagenes de los jugadores en un jFrame con un fondo, si me dan una mano estare agradecido, aqui dejo parte del codigo
yo lo que quisiera es pintar las imagenes de la clase panelcrearpersonaje en el fondo que esta en campodebatalla asi a medida que se crea un personaje se pinta en un jframe con el fondo y simula la batalla
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
package Game.tokio.Grafic;
import Game.tokio.Ejercito;
import Game.tokio.Personajes.*;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
public class PanelCrearPersonaje extends JPanel {
private JTextField jtNombre;
private JComboBox<String> jcTipo;
private JTextField jtVida;
private JTextField jtArmadura;
private JButton btaniadir;
public static ImageIcon imageIcon;
public static JLabel label;
// private ImagenPersonajes imagenPersonajes;
private ArrayList<ImageIcon> imagenHeroes;
private ArrayList<ImageIcon> imagenBestias;
public ArrayList<ImageIcon> getImagenHeroes() {
return imagenHeroes;
}
public ArrayList<ImageIcon> getImagenBestias() {
return imagenBestias;
}
//Atributo para referenciar alguno de los ejercitos de la clase main
private Ejercito ejercito;
//Panel con la lista de personajes
private PanelLista lista;
public PanelCrearPersonaje(String titulo, String[] tipos, Ejercito ejercito) {
// inicializamos componentes
jtNombre = new JTextField();
jcTipo = new JComboBox<String>(tipos);
jtVida = new JTextField();
jtArmadura = new JTextField();
btaniadir = new JButton("añadir");
btaniadir.addActionListener(new AccionCrearPersonaje());
imagenBestias = new ArrayList<>();
imagenHeroes = new ArrayList<>();
this.ejercito = ejercito; // Ejercito referenciado
lista = new PanelLista(titulo, ejercito);//pasamos titulo de borde y referencia a ejercito
// layout de "cajas" verticales
setLayout(new BoxLayout(this, BoxLayout.Y_AXIS));
// cada "caja" apilada verticalmente sera un panel de la clase panleConLabel
add(new PanelConLabel("Nombre: ", jtNombre));
add(new PanelConLabel("Tipo: ", jcTipo));
add(new PanelConLabel("Vida: ", jtVida));
add(new PanelConLabel("Armadura: ", jtArmadura));
// Boton añadir
JPanel pnAniadir = new JPanel();
pnAniadir.add(btaniadir);
add(pnAniadir);
//colocamos el panel lista debajo de todo
add(lista);
// combinamos dos bordes, uno titulador y otro vacio para crear algo de relleno
setBorder(BorderFactory.createCompoundBorder(
BorderFactory.createEmptyBorder(15, 15, 15, 15),
BorderFactory.createTitledBorder(titulo)));
}
private class PanelConLabel extends JPanel {
public PanelConLabel(String texto, JComponent componente) {
//Layout tipo grilla, sera una fila con dos columnas
setLayout(new GridLayout(1, 2));
// En la primera columna, la etiqueta
add(new JLabel(texto));
// En la segunda columna, el componente que acompaña la etiqueta
add(componente);
// inflamos el panel con aldo de borde vacio
setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
}
}
public class AccionCrearPersonaje implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
// Recogemos datos
String nombre = jtNombre.getText();
String tipo = (String) jcTipo.getSelectedItem();
int vida = Integer.parseInt(jtVida.getText());
int armadura = Integer.parseInt(jtArmadura.getText());
ArrayList<ImageIcon> iconos = new ArrayList<>();
imageIcon = new ImageIcon();
label = new JLabel(imageIcon);
// ImagenPersonajes imagenPersonajes = new ImagenPersonajes();
// Creamos nuevo personaje segun tipo
switch (tipo) {
case "Elfo":
ejercito.addPersonaje(new Elfos(nombre, vida, armadura));
ImageIcon imagenElfo = new ImageIcon(getClass()
.getResource("/Game/tokio/Multimedia/elfo.PNG"));
imageIcon = imagenElfo;
break;
case "Humano":
ejercito.addPersonaje(new Humanos(nombre, vida, armadura));
ImageIcon imagenHumano = new ImageIcon(getClass()
.getResource("/Game/tokio/Multimedia/humano1.PNG"));
imageIcon = imagenHumano;
break;
case "Hobbit":
ejercito.addPersonaje(new Hobbits(nombre, vida, armadura));
ImageIcon imagenHobbit = new ImageIcon(getClass()
.getResource("/Game/tokio/Multimedia/hobbit.PNG"));
imageIcon = imagenHobbit;
break;
case "Trasgo":
ejercito.addPersonaje(new Trasgos(nombre, vida, armadura));
ImageIcon imagenTrasgo = new ImageIcon(getClass()
.getResource("/Game/tokio/Multimedia/trasgo.PNG"));
imageIcon = imagenTrasgo;
break;
case "Orco":
ejercito.addPersonaje(new Orcos(nombre, vida, armadura));
ImageIcon imagenOrco = new ImageIcon(getClass()
.getResource("/Game/tokio/Multimedia/orco1.PNG"));
imageIcon = imagenOrco;
break;
}
JLabel label;
label= new JLabel(imageIcon);
// Limpiamos campos
jtNombre.setText(null);
jcTipo.setSelectedIndex(0);
jtVida.setText(null);
jtArmadura.setText(null);
add(label);
revalidate();
repaint();
//Actualiza lista
lista.actualizarLista();
}
}
public void Paint(Graphics2D g){
g.drawImage(imageIcon.getImage(),50,10,15,15,null);
setOpaque(false);
super.paint(g);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
package Game.tokio.Grafic;
import Game.tokio.Batalla;
import Game.tokio.Ejercito;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class BatallaGr extends JFrame{
//Modelo
private Ejercito bestias;
private Ejercito heroes;
// Vistas
private PanelCrearPersonaje crearHeroes;
private PanelCrearPersonaje crearBestias;
private PanelTexto textArea;
private Fondo fondo ;
private PanelCrearPersonaje image;
private CampoDeBatalla campoDeBatalla;
public BatallaGr() {
bestias = new Ejercito();
heroes = new Ejercito();
crearHeroes = new PanelCrearPersonaje("Heroes", new String[]{"Elfo", "Humano", "Hobbit"}, heroes);
crearBestias = new PanelCrearPersonaje("Bestias", new String[]{"Orco", "Trasgo"}, bestias);
textArea = new PanelTexto();
textArea.setAccionBotonLuchar(new AccionBotonLuchar());
campoDeBatalla = new CampoDeBatalla(crearHeroes,crearBestias);
fondo = new Fondo(this);
JPanel pnSuperior = new JPanel();
pnSuperior.add(crearHeroes);
pnSuperior.add(crearBestias);
JFrame frameBatalla = new JFrame();
campoDeBatalla.setBorder(BorderFactory.createCompoundBorder(
BorderFactory.createEmptyBorder(15, 15, 15, 15),
BorderFactory.createTitledBorder("titulo")));
frameBatalla.setContentPane(campoDeBatalla);
frameBatalla.setSize(700,500);
frameBatalla.setVisible(true);
setLayout(new BoxLayout(getContentPane(),BoxLayout.Y_AXIS));
add(textArea);
add(pnSuperior);
setTitle("Batalla señor de los anillos ");
setSize(2000,1832);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setLocationRelativeTo(null);
setVisible(true);
}
public void getGraphics(Graphics2D g) {
super.paint(g);
fondo.paint(g);
crearBestias.paint(g);
crearHeroes.paint(g);
}
private class AccionBotonLuchar implements ActionListener{
@Override
public void actionPerformed(ActionEvent e){
textArea.BorrarText();
BatallaHilo batallaHilo = new BatallaHilo(heroes,bestias,textArea);
batallaHilo.start();
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new BatallaGr();
}
});
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
package Game.tokio.Grafic;
import Game.tokio.Ejercito;
import javax.imageio.ImageIO;
import javax.swing.*;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
public class CampoDeBatalla extends JPanel {
private BufferedImage backgroundImage;
private Ejercito heroes;
private Ejercito bestias;
PanelCrearPersonaje crearheroes;
PanelCrearPersonaje crearbestias;
public CampoDeBatalla(PanelCrearPersonaje crearheroes, PanelCrearPersonaje crearbestias) {
this.heroes = heroes;
this.bestias = bestias;
this.crearheroes = crearheroes;
this.crearbestias = crearbestias;
try {
backgroundImage = ImageIO.read(new File("/Users/gian/IdeaProjects/ProyectoFinal_GianlucaMaida/src/Game/tokio/Multimedia/FondoJuego.png"));
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// Dibuja el fondo
g.drawImage(backgroundImage, 15, 15, 600, 400, null);
revalidate();
repaint();
//
Valora esta pregunta


0