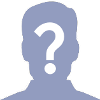
Programa Java
Publicado por Sergio (2 intervenciones) el 10/01/2024 19:59:37
Necesito un programa que transforme un archivo .docx a .txt con un limite de 70 caracteres, que reemplace los saltos de linea por este simbolo "¬" y los tabuladores por 4 espacios, tambien debe reconocer y escribir viñetas (1. 2. 3. , A. B. C. ....), solo he conseguido que transofrme a .txt con un limite de 70 caracteres pero no reemplaza nada ni escribe viñetas, si alguien puede ayudarme se lo agradeceria mucho, voy a dejar el codigo aqui abajo
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
package snippet;
import java.awt.HeadlessException;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.PrintWriter;
import javax.swing.JFileChooser;
import javax.swing.filechooser.FileNameExtensionFilter;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
public class DocxToTxt {
public static void main(String[] args) throws IOException {
try {
// Solicita al usuario seleccionar un archivo .docx
File docxFile = selectDocxFile();
if (docxFile == null) {
System.out.println("No .docx file selected.");
return;
}
// Define la ruta para guardar el archivo .txt en el escritorio
String txtFilePath = System.getProperty("user.home") + "/Desktop/output.txt";
// Convierte el archivo .docx a .txt
convertDocxToTxt(docxFile, txtFilePath);
System.out.println("Conversion completed. Check the " + txtFilePath + " file.");
} catch (HeadlessException e) {
e.printStackTrace();
}
}
// Método para permitir al usuario seleccionar un archivo .docx mediante un cuadro de diálogo
private static File selectDocxFile() {
JFileChooser fileChooser = new JFileChooser();
fileChooser.setFileFilter(new FileNameExtensionFilter("Microsoft Word Document", "docx"));
int result = fileChooser.showOpenDialog(null);
if (result == JFileChooser.APPROVE_OPTION) {
return fileChooser.getSelectedFile();
}
return null;
}
// Método para convertir el contenido de un archivo .docx en un archivo .txt
private static void convertDocxToTxt(File docxFile, String txtFilePath) throws IOException {
try (XWPFDocument document = new XWPFDocument(new FileInputStream(docxFile));
FileOutputStream fos = new FileOutputStream(txtFilePath);
PrintWriter writer = new PrintWriter(fos)) {
for (XWPFParagraph paragraph : document.getParagraphs()) {
String text = paragraph.getText();
if (text != null && !text.trim().isEmpty()) {
String[] lines = text.split("[\\r\\n]+"); // Divide el texto en líneas para no cortar las palabras
for (String line : lines) {
line = line.replace("\n", "¬"); // Reemplaza saltos de línea con "¬" (NO FUNCIONA)
writeTextWithLimit(line, writer);
}
}
}
}
}
// Método para escribir el texto con un límite de 70 caracteres por línea
private static void writeTextWithLimit(String text, PrintWriter writer) {
StringBuilder lineBuilder = new StringBuilder();
String[] words = text.split("\\s+");
for (String word : words) {
if (lineBuilder.length() + word.length() + 1 <= 70) {
if (lineBuilder.length() > 0) {
lineBuilder.append(" ");
}
lineBuilder.append(word);
} else {
writer.println(lineBuilder.toString());
lineBuilder.setLength(0);
lineBuilder.append(word);
}
}
if (lineBuilder.length() > 0) {
writer.println(lineBuilder.toString());
}
}
}
Valora esta pregunta


0