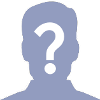
Validar contraseñas iguales
Publicado por Sebastian (35 intervenciones) el 20/05/2015 06:40:43
Saludos, necesito de su apoyo y conocimiento
Mi problema es el siguiente. En un formulario tengo dos campos uno de contraseña y otro confirmar contraseña, necesito que me compare esos campos y si son diferentes me muestre un mensaje de alerta, si son iguales simplemente no hace nada.
Este es todo el código que tengo para hacer el registro, el problema es que no se en que parte de mi código insertar la validación..
De aqui tomo las contrasenias ingresadas
PD. Para hacer el registro utilice dreamweaver
Ojala me puedan ayudar muchas gracias...
Mi problema es el siguiente. En un formulario tengo dos campos uno de contraseña y otro confirmar contraseña, necesito que me compare esos campos y si son diferentes me muestre un mensaje de alerta, si son iguales simplemente no hace nada.
Este es todo el código que tengo para hacer el registro, el problema es que no se en que parte de mi código insertar la validación..
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
<?php
if (!function_exists("GetSQLValueString")) {
function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "")
{
if (PHP_VERSION < 6) {
$theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue;
}
$theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue);
switch ($theType) {
case "text":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "long":
case "int":
$theValue = ($theValue != "") ? intval($theValue) : "NULL";
break;
case "double":
$theValue = ($theValue != "") ? doubleval($theValue) : "NULL";
break;
case "date":
$theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL";
break;
case "defined":
$theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue;
break;
}
return $theValue;
}
}
$editFormAction = $_SERVER['PHP_SELF'];
if (isset($_SERVER['QUERY_STRING'])) {
$editFormAction .= "?" . htmlentities($_SERVER['QUERY_STRING']);
}
if ((isset($_POST["MM_insert"])) && ($_POST["MM_insert"] == "form1")) {
$insertSQL = sprintf("INSERT INTO usuario (usu_usuario, usu_password, usu_nombres, usu_apellidos, usu_telefono, usu_nac, usu_direccion, usu_email, tipousu_id) VALUES (%s, %s, %s, %s, %s, %s, %s, %s, %s)",
GetSQLValueString($_POST['usu_usuario'], "text"),
GetSQLValueString($_POST['usu_password'], "text"),
GetSQLValueString($_POST['usu_nombres'], "text"),
GetSQLValueString($_POST['usu_apellidos'], "text"),
GetSQLValueString($_POST['usu_telefono'], "text"),
GetSQLValueString($_POST['usu_nac'], "date"),
GetSQLValueString($_POST['usu_direccion'], "text"),
GetSQLValueString($_POST['usu_email'], "text"),
GetSQLValueString($_POST['usu_tipo'], "int"));
mysql_select_db($database_prueba, $prueba);
$Result1 = mysql_query($insertSQL, $prueba) or die(mysql_error());
$insertGoTo = "registrarusuarios.php";
if (isset($_SERVER['QUERY_STRING'])) {
$insertGoTo .= (strpos($insertGoTo, '?')) ? "&" : "?";
$insertGoTo .= $_SERVER['QUERY_STRING'];
echo ' <script language="javascript"> alert("Usuario registrado con éxito");</script> ';
}
header(sprintf("Location: %s", $insertGoTo));
}
?>
De aqui tomo las contrasenias ingresadas
1
2
3
4
5
6
7
8
9
10
11
12
<div class="form-group">
<label class="control-label col-xs-3" for="inputPassword">Contraseña:</label>
<div class="col-xs-9">
<input type="password" class="form-control" id="usu_password" name="usu_password" value="" placeholder="Contraseña" required="required">
</div>
</div>
<div class="form-group">
<label class="control-label col-xs-3" for="confirmPassword">Confirmar Contraseña:</label>
<div class="col-xs-9">
<input type="password" class="form-control" id="usu_confirm" name="usu_confirm" placeholder="Confirmar Contraseña" required="required">
</div>
</div>
PD. Para hacer el registro utilice dreamweaver
Ojala me puedan ayudar muchas gracias...
Valora esta pregunta


0