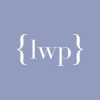
Juego de la serpiente con PyGame
Python
Publicado el 9 de Abril del 2020 por Administrador (718 códigos)
66.423 visualizaciones desde el 9 de Abril del 2020
Juego de la serpente con pyGame
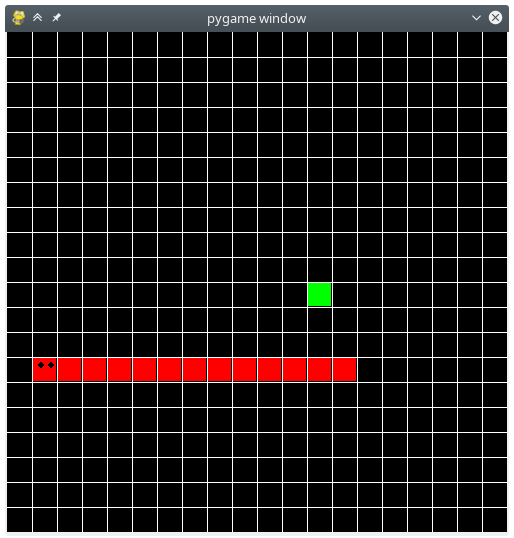
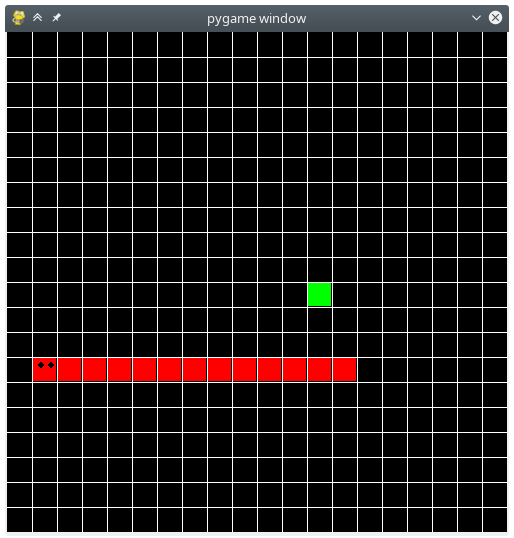
#Snake Tutorial Python
import math
import random
import pygame
import tkinter as tk
from tkinter import messagebox
class cube(object):
rows = 20
w = 500
def __init__(self,start,dirnx=1,dirny=0,color=(255,0,0)):
self.pos = start
self.dirnx = 1
self.dirny = 0
self.color = color
def move(self, dirnx, dirny):
self.dirnx = dirnx
self.dirny = dirny
self.pos = (self.pos[0] + self.dirnx, self.pos[1] + self.dirny)
def draw(self, surface, eyes=False):
dis = self.w // self.rows
i = self.pos[0]
j = self.pos[1]
pygame.draw.rect(surface, self.color, (i*dis+1,j*dis+1, dis-2, dis-2))
if eyes:
centre = dis//2
radius = 3
circleMiddle = (i*dis+centre-radius,j*dis+8)
circleMiddle2 = (i*dis + dis -radius*2, j*dis+8)
pygame.draw.circle(surface, (0,0,0), circleMiddle, radius)
pygame.draw.circle(surface, (0,0,0), circleMiddle2, radius)
class snake(object):
body = []
turns = {}
def __init__(self, color, pos):
self.color = color
self.head = cube(pos)
self.body.append(self.head)
self.dirnx = 0
self.dirny = 1
def move(self):
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
keys = pygame.key.get_pressed()
for key in keys:
if keys[pygame.K_LEFT]:
self.dirnx = -1
self.dirny = 0
self.turns[self.head.pos[:]] = [self.dirnx, self.dirny]
elif keys[pygame.K_RIGHT]:
self.dirnx = 1
self.dirny = 0
self.turns[self.head.pos[:]] = [self.dirnx, self.dirny]
elif keys[pygame.K_UP]:
self.dirnx = 0
self.dirny = -1
self.turns[self.head.pos[:]] = [self.dirnx, self.dirny]
elif keys[pygame.K_DOWN]:
self.dirnx = 0
self.dirny = 1
self.turns[self.head.pos[:]] = [self.dirnx, self.dirny]
for i, c in enumerate(self.body):
p = c.pos[:]
if p in self.turns:
turn = self.turns[p]
c.move(turn[0],turn[1])
if i == len(self.body)-1:
self.turns.pop(p)
else:
if c.dirnx == -1 and c.pos[0] <= 0: c.pos = (c.rows-1, c.pos[1])
elif c.dirnx == 1 and c.pos[0] >= c.rows-1: c.pos = (0,c.pos[1])
elif c.dirny == 1 and c.pos[1] >= c.rows-1: c.pos = (c.pos[0], 0)
elif c.dirny == -1 and c.pos[1] <= 0: c.pos = (c.pos[0],c.rows-1)
else: c.move(c.dirnx,c.dirny)
def reset(self, pos):
self.head = cube(pos)
self.body = []
self.body.append(self.head)
self.turns = {}
self.dirnx = 0
self.dirny = 1
def addCube(self):
tail = self.body[-1]
dx, dy = tail.dirnx, tail.dirny
if dx == 1 and dy == 0:
self.body.append(cube((tail.pos[0]-1,tail.pos[1])))
elif dx == -1 and dy == 0:
self.body.append(cube((tail.pos[0]+1,tail.pos[1])))
elif dx == 0 and dy == 1:
self.body.append(cube((tail.pos[0],tail.pos[1]-1)))
elif dx == 0 and dy == -1:
self.body.append(cube((tail.pos[0],tail.pos[1]+1)))
self.body[-1].dirnx = dx
self.body[-1].dirny = dy
def draw(self, surface):
for i, c in enumerate(self.body):
if i ==0:
c.draw(surface, True)
else:
c.draw(surface)
def drawGrid(w, rows, surface):
sizeBtwn = w // rows
x = 0
y = 0
for l in range(rows):
x = x + sizeBtwn
y = y + sizeBtwn
pygame.draw.line(surface, (255,255,255), (x,0),(x,w))
pygame.draw.line(surface, (255,255,255), (0,y),(w,y))
def redrawWindow(surface):
global rows, width, s, snack
surface.fill((0,0,0))
s.draw(surface)
snack.draw(surface)
drawGrid(width,rows, surface)
pygame.display.update()
def randomSnack(rows, item):
positions = item.body
while True:
x = random.randrange(rows)
y = random.randrange(rows)
if len(list(filter(lambda z:z.pos == (x,y), positions))) > 0:
continue
else:
break
return (x,y)
def message_box(subject, content):
root = tk.Tk()
root.attributes("-topmost", True)
root.withdraw()
messagebox.showinfo(subject, content)
try:
root.destroy()
except:
pass
def main():
global width, rows, s, snack
width = 500
rows = 20
win = pygame.display.set_mode((width, width))
s = snake((255,0,0), (10,10))
snack = cube(randomSnack(rows, s), color=(0,255,0))
flag = True
clock = pygame.time.Clock()
while flag:
pygame.time.delay(50)
clock.tick(10)
s.move()
if s.body[0].pos == snack.pos:
s.addCube()
snack = cube(randomSnack(rows, s), color=(0,255,0))
for x in range(len(s.body)):
if s.body[x].pos in list(map(lambda z:z.pos,s.body[x+1:])):
print('Score: ', len(s.body))
message_box('You Lost!', 'Play again...')
s.reset((10,10))
break
redrawWindow(win)
pass
main()
Comentarios sobre la versión: 20181031 (9)
TypeError: Iterating over key states is not supported
Alguna ayuda porfa. Gracias
import aleatorio
import tiempo
puntuaci贸n_jugador = 0
puntuaci贸n_m谩s_alta = 0
tiempo_retraso = 0.1
# pantalla creada
wind = turtle.Screen()
wind.title("Laberinto de serpientes馃悕")
wind.bgcolor("rojo")
# el tama帽o de la pantalla
wind.setup(width=600, height=600)
# crear la serpiente
snake = turtle.Turtle()
snake.shape("cuadrado")
snake.color("negro")
snake.penup()
snake.goto(0, 0)
snake.direction = "Stop"
# crear la comida
snake_food = turtle.Turtle()
shapes = random.choice('tri谩ngulo','c铆rculo')
snake_food.shape(shapes)
snake_food.color("azul")
snake_food.speed(0)
snake_food.penup()
snake_food.goto(0, 100)
pen = tortuga.Turtle()
pen.speed(0)
pen.shape('cuadrado')
pen.color('blanco')
pen.penup()
pen.hideturtle()
pen.goto(0, 250)
pen.write("Your_score: 0 Highest_Score : 0", align="center",
font=("Arial", 24, "normal"))
turtle.mainloop()
# Asignaci贸n de direcciones
def moveleft():
if snake.direction != "right":
snake.direction = "left"
def moveright():
if snake.direction != "left":
snake.direction = "right"
def moveup():
if snake.direction != "down":
snake.direction = "up"
def movedonw():
if snake.direction != "up":
snake.direction = "down"
def move():
if snake.direction == "up":
coord_y = snake.ycor()
snake.sety(coord_y+20)
if snake.direction == "down":
coord_y = snake.ycor()
snake.sety(coord_y-20)
if snake.direction == "right":
coord_x = snake.xcor()
snake.setx(coord_x+20)
if snake.direction == "left":
coord_x = snake.xcor()
snake.setx(coord_x-20)
wind.listen()
wind.onkeypress(moveeft, 'L')
wind.onkeypress(moveright, 'R')
wind.onkeypress(moveup, 'U')
wind.onkeypress(movedown, 'D')
segmentos = []
#Implementacion de la jugabilidad
while True:
wind.update()
if snake.xcor() > 290 or snake.xcor() < -290 or snake.ycor() > 290 or snake.ycor() < -290:
time.sleep(1)
snake.goto(0, 0)
snake.direction = "Stop"
snake.shape("square")
snake.color("green")
for segment in segments:
segment.goto(1000, 1000)
segments.clear()
player_score = 0
delay_time = 0.1
pen.clear()
pen.write("Puntuacion_jugador: {} Puntuacion_mas_alta: {}".format(puntuacion_jugador, puntuaci贸n_mas_alta), align="center", font=("Arial", 24, "normal"))
if distancia_serpiente(comida_serpiente) < 20:
coord_x = random.randint(-270, 270)
coord_y = random.randint(-270, 270)
snake_food.goto(coord_x, coord_y)
# Añadir segmento
added_segment = turtle.Turtle()
added_segment.speed(0)
added_segment.shape("square")
added_segment.color("white")
added_segment.penup()
segments.append(added_segment)
delay_time -= 0.001
player_score = 5
if player_score > highest_score:
highest_score = player_score
pen.clear()
pen.write("Puntuacion_del_jugador: {} Puntuacion_mas_alta: {}".format(puntuaci0n_jugador, puntuacion_mas_alta), align="center", font=("Arial", 24, "normal"))
# comprobaci贸n de colisiones
for i in range(len(segments)-1, 0, -1):
coord_x = segments[i-1].xcor()
coord_y = segments[i-1].ycor()
segments[i].goto(coord_x, coord_y)
if len(segments) > 0:
coord_x = snake.xcor()
coord_y = snake.ycor()
segments[0].goto(coord_x, coord_y)
move()
for segment in segments:
if segment.distance(snake) < 20:
time.sleep(1)
snake.goto(0, 0)
snake.direction = "stop"
snake.color('white')
snake.shape('square')
for segment in segments:
segment.goto(1000, 1000)
segment.clear()
player_score = 0
delay_time = 0.1
pen.clear()
pen.write("Puntuacion_jugador: {} Puntuacion_mas_alta: {}".format(puntuacion_jugador, puntuacion_mas_alta), align="center", font=("Arial", 24, "normal"))
time.sleep(delay_time)
turtle.mainloop()