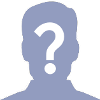
Cliente-Servidor TCP duda
Publicado por damian (3 intervenciones) el 08/04/2017 04:52:34
Hola a todos tengo estos codigos de cliente servidor protocolo TCP en el cual el cliente manda un mensaje al servidor y el servidor lo retorna transformado en mayusculas, el problema empezo cuando quise hacer que todo lo que obtenga el servidor lo anote en un documento de texto, solo lo hace una vez con un solo mensaje cuando le mando otro mensaje me sale error Stream Closed, quiza me este faltando o no vea el problema agradezco ayuda muchas gracias.
Server :
Cliente :
Server :
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
package conectar1;
import java.io.BufferedReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintStream;
import java.io.PrintWriter;
import java.net.ServerSocket;
import java.net.Socket;
public class server {
public static void main(String[] args) throws IOException {
ServerSocket s; //Socket servidor
Socket sc; //Socket cliente
PrintStream p; //Canal de escritura
BufferedReader b; //Canal de Lectura
String mensaje;
try {
//Creo el socket server
s = new ServerSocket(12000);
//Invoco el metodo accept del socket servidor, me devuelve una referencia al socket cliente
sc = s.accept();
//Obtengo una referencia a los canales de escritura y lectura del socket cliente
b = new BufferedReader( new InputStreamReader ( sc.getInputStream() ) );
p = new PrintStream ( sc.getOutputStream() );
while ( true ) {
//Leo lo que escribio el socket cliente en el canal de lectura
mensaje = b.readLine();
System.out.println(mensaje);
//Escribo en el texto el mensaje enviado por cliente
FileWriter fichero = null;
PrintWriter pw = null;
try
{
fichero = new FileWriter("C:\\/holanda.txt"); //Abro el fichero
pw = new PrintWriter(fichero);
pw.println(mensaje); //Escribo en el fichero
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
// Nuevamente aprovechamos el finally para
// asegurarnos que se cierra el fichero.
if (null != fichero)
fichero.close();
} catch (Exception e2) {
e2.printStackTrace();
}
p.println(mensaje.toUpperCase());
}
if ( mensaje.equals("“by”")) {
break;
}
p.close();
b.close();
sc.close();
s.close();
}
} catch (IOException e) {
System.out.println( e.getMessage());
}
}
}
Cliente :
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
package conectar1;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintStream;
import java.net.Socket;
import java.net.UnknownHostException;
public class cliente {
public static void main(String[] args) {
Socket s;
PrintStream p;
BufferedReader b;
String host = "localhost";
int port = 12000;
String respuesta;
//Referencia a la entrada por consola (System.in)
BufferedReader in = new BufferedReader(new InputStreamReader(System.in));
try {
//Creo una conexion al socket servidor
s = new Socket(host,port);
//Creo las referencias al canal de escritura y lectura del socket
p = new PrintStream(s.getOutputStream());
b = new BufferedReader ( new InputStreamReader ( s.getInputStream() ) );
while ( true ) {
//Ingreso un String por consola
System.out.print("Mensaje a enviar: ");
//Escribo en el canal de escritura del socket
p.println( in.readLine() );
//Espero la respuesta por el canal de lectura
respuesta = b.readLine();
System.out.println(respuesta);
if ( respuesta.equals("by")) {
break;
}
}
p.close();
b.close();
s.close();
} catch (UnknownHostException e) {
System.out.println("No puedo conectarme a " + host + ":" + port);
} catch (IOException e) {
System.out.println("Error de E/S en " + host + ":" + port);
}
}
}
Valora esta pregunta


0