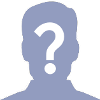
este programa es una cola o una lista?
Publicado por paola (10 intervenciones) el 01/05/2013 20:16:43
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
#include <iostream.h>
#include <stdlib.h>
using namespace std;
struct nodo{
int num;
struct nodo *sig;
};
typedef struct nodo *Lista;
void insertarInicio(Lista &lista, int valor)
{
Lista q;
q = new(struct nodo);
q->num = valor;
q->sig = lista;
lista = q;
}
void buscarElemento(Lista lista, int valor)
{
Lista q = lista;
int i = 1, band = 0;
while(q!=NULL)
{
if(q->num==valor)
{
cout<<endl<<" Encontrada en posicion "<< i <<endl;
band = 1;
}
q = q->sig;
i++;
}
if(band==0)
cout<<"\n\n Numero no encontrado..!"<< endl;
}
void reportarLista(Lista lista)
{
int i = 0;
while(lista != NULL)
{
cout <<' '<< i+1 <<") " << lista->num << endl;
lista = lista->sig;
i++;
}
}
int contarNodos(nodo *Lista){
int p;
int cont = 0;
while(Lista!=NULL){
Lista = Lista->sig;
cont++;
}
return cont;
}
int main()
{
Lista lista = NULL;
int op; // opcion del menu
int _dato; // elemenento a ingresar
int pos; // posicion a insertar
do{
do{
cout<<"\n\t\tLISTA ENLAZADA SIMPLE\n\n";
cout<<" 1. INSERTAR AL INICIO "<<endl;
cout<<" 2. REPORTAR LISTA "<<endl;
cout<<" 3. BUSCAR ELEMENTO "<<endl;
cout<<" 4. CONTAR NODOS "<<endl;
cout<<" 5. SALIR "<<endl;
cout<<"\n INGRESE OPCION: ";
cin>>op;
}while(op<1 || op>5);
switch(op)
{
case 1:
cout<< "\n NUMERO A INSERTAR: ";
cin>> _dato;
insertarInicio(lista, _dato);
break;
case 2:
cout << "\n\n MOSTRANDO LISTA\n\n";
reportarLista(lista);
break;
case 3:
cout<<"\n Valor a buscar: ";
cin>> _dato;
buscarElemento(lista, _dato);
break;
case 4:
int nNodos = contarNodos(lista);
cout<<nNodos<<" nodos en la lista"<<endl;
contarNodos(lista);
break;
}
cout<<endl<<endl;
system("pause");
system("cls");
}while(op!=5);
return 0;
}
Valora esta pregunta


0