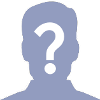
¿como puedo ordenar una lista simple al momento de agregar el dato y como eliminar cualquier dato ?
Publicado por Nancy (1 intervención) el 20/06/2015 17:52:35
necesito que el programa me ordene al momento de ingresar el dato
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
#include<stdio.h>
#include<conio.h>
#include<string.h>
#include<windows.h>
#include<stdlib.h>
#include <time.h>
struct simple
{
int dato;
struct simple *sig;
};
struct simple *primero,*ultimo;
typedef struct simple NODO;//DEFINE TIPO DE DATO A PARTIR DE LA ESTRUCTURA DE simple
typedef NODO *NODOPTR;
int isEmpty(NODOPTR cima)
{
return (cima == NULL);
}
void insert(NODOPTR *cima,int num) //AGREGAR EN LISTA SIMPLE
{
NODOPTR nuevo;
NODOPTR actual;
nuevo=(NODO *)malloc (sizeof(NODO));
if(nuevo==NULL){
printf("\n\n\t\tNo puede ser Insertado\n");
}
if(isEmpty(*cima))
{
nuevo->dato=num;
nuevo->sig=NULL;
*cima=nuevo;
}else{
actual = *cima;
while(actual->sig != NULL)
{
actual = actual->sig;
}
nuevo->dato = num;
nuevo->sig = NULL;
actual->sig = nuevo;
}
}
void mostrar_ls(NODOPTR cima) //MOSTRAR LISTA SIMPLE
{
system("cls");
if(cima == NULL)
{
printf("\n\nNo hay Datos");
}else{
while(cima != NULL)
{
printf("\n\n \t %d ",cima->dato);
cima = cima->sig;
}printf("\n\n\t NULL");
}
getch();
}
void menu_prin() //menu principal de listas
{
system("cls");
int opc;
printf("\n \n\t\tT I P O S D E L I S T A S");
printf("\n \n\t\t 1.- Lista Simple");
printf("\n \n\t\t 2.- Lista Simple Circular");
printf("\n \n\t\t 3.- Lista Doble");
printf("\n \n\t\t 4.- Lista Doble Circular");
printf("\n \n\t\t 5.- Salir ");
printf("\n \n\tSeleccione una opcion: ");
}
void menu_lsim() //menu de opciones
{
NODOPTR cima = NULL;
int op2,num;
do{ system("cls");
printf("\n \n\t\tM E N U D E O P C I O N E S");
printf("\n \n\t\t 1.- Agregar");
printf("\n \n\t\t 2.- Eliminar");
printf("\n \n\t\t 3.- Mostrar Todos");
printf("\n \n\t\t 4.- Mostrar Siguiente");
printf("\n \n\t\t 5.- Regresar a Menu");
printf("\n \n\tSeleccione una opcion: ");
scanf("%d", &op2);
switch(op2)
{
case 1:
system("cls");
printf("\n\n\n\tInserte un Numero: ");scanf("%d",&num);
insert(&cima,num);
break;
case 2:
system("cls");
int numero;
printf("\n\t Ingrese Numero a Eliminar : ");
scanf("%d",&numero);
//eliminar(numero);
break;
case 3: //todos
mostrar_ls(cima);getch();
break;
case 4: //siguiente
break;
}
}while(op2!=5);
}
int main()
{
primero = (struct simple *) NULL;
ultimo = (struct simple *) NULL;
int opc, op2,op3;
system("cls");
menu_prin();
do
{ scanf("%d", &opc);
switch (opc) //LISTAS
{
case 1:
system("cls");
printf("L I S T A S I M P L E");
menu_lsim();
menu_prin();
}
}while(opc!=5);
}
Valora esta pregunta


0