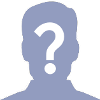
Ayuda con código *java.lang.ArrayIndexOutOfBoundsException*
Publicado por Alejandro (14 intervenciones) el 13/11/2016 22:55:05
Hola buenas tardes, necesito de su ayuda con mi siguiente código, agradecería su ayuda
Incluyo el código en este mismo recuadro.
Incluyo el código en este mismo recuadro.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.LinkedList;
import java.util.Queue;
public class FordFulkerson1 {
private int[] padre;
private Queue<Integer> cola;
private int numeroVertices;
private boolean[] visitado;
public FordFulkerson1(int numeroVertices){
this.numeroVertices = numeroVertices;
this.cola = new LinkedList<Integer>();
padre = new int[numeroVertices + 1];
visitado = new boolean[numeroVertices + 1];
}
public boolean Busqueda(int fuente, int des, int grafo[][]){
boolean Encontrado = false;
int destino, elemento;
for(int vertice = 1; vertice <= numeroVertices; vertice++)
{
padre[vertice] = -1;
visitado[vertice] = false;
}
cola.add(fuente);
padre[fuente] = -1;
visitado[fuente] = true;
while (!cola.isEmpty())
{
elemento = cola.remove();
destino = 1;
while (destino <= numeroVertices)
{
if (grafo[elemento][destino] > 0 && !visitado[destino])
{
padre[destino] = elemento;
cola.add(destino);
visitado[destino] = true;
}
destino++;
}
}
if(visitado[des])
{
Encontrado = true;
}
return Encontrado;
}
public int fordFulkerson(int grafo[][], int fuente, int destino){
int u, v;
int maximoFlujo = 0;
int pathFlujo;
int[][] grafoResidual = new int[numeroVertices + 1][numeroVertices + 1];
for (int fuenteVertice = 1; fuenteVertice <= numeroVertices; fuenteVertice++)
{
for (int destinoVertice = 1; destinoVertice <= numeroVertices; destinoVertice++)
{
grafoResidual[fuenteVertice][destinoVertice] = grafo[fuenteVertice][destinoVertice];
}
}
while (Busqueda(fuente ,destino, grafoResidual))
{
pathFlujo = Integer.MAX_VALUE;
for (v = destino; v != fuente; v = padre[v])
{
u = padre[v];
pathFlujo = Math.min(pathFlujo, grafoResidual[u][v]);
}
for (v = destino; v != fuente; v = padre[v])
{
u = padre[v];
grafoResidual[u][v] -= pathFlujo;
grafoResidual[v][u] += pathFlujo;
}
maximoFlujo += pathFlujo;
}
return maximoFlujo;
}
public static void main(String args[]){
int[][] grafo;
int numberOfNodes = 4;
int fuente = 0;
int sink = 3;
int maximoFlujo;
String lineas[] = null;
File archivo = new File("matriz.txt");
try{
if(archivo.exists()){
grafo = new int[4][4];
BufferedReader bf = new BufferedReader(new FileReader(archivo));
int Dimencion = 0;
String Linea = bf.readLine();
while(Linea != null && Dimencion<4){
String[] tmp = Linea.split(" ");
for(int x=0;x<4;x++)
grafo[Dimencion][x] = Integer.valueOf(tmp[x]);
Dimencion++;
Linea = bf.readLine();
}
FordFulkerson1 fordFulkerson = new FordFulkerson1(numberOfNodes);
maximoFlujo = fordFulkerson.fordFulkerson(grafo, fuente, sink);
System.out.println("El flujo maximo es: " + maximoFlujo);
}
} catch (IOException ex) {
System.err.println(ex);
}
}
}
- codigo.rar(1,3 KB)
Valora esta pregunta


0