JFrame en el centro de la pantalla – Java Swing
Java
Publicado el 18 de Enero del 2016 por Julio (12 códigos)
9.680 visualizaciones desde el 18 de Enero del 2016
Vamos a ver en este código como centrar un JFrame en Java Swing, en este sencilllo ejemplo con Java Swing simplemente se crea un JFrame con Netbeans para añadir un par de JLabel con el editor gráfico y utilizar el método para centrarlo.
Aquí os dejo el primero de una serie de códigos sobre Java Swing ( http://codigoxules.org/java/java-swing/) que iré publicando próximamente.
El objetivo de este código es explicar como centrar un JFrame o colocarlo en la posición que quieras de forma sencilla. Estos es el método que se puede utilizar en cualquier clase con JFrame:
Así que para colocarlo en el centro de la pantalla simplemente añadimos otro método por comodidad:
En el método main encontrarás el ejemlo de utilización:
Este será el resultado del ejemplo usando:
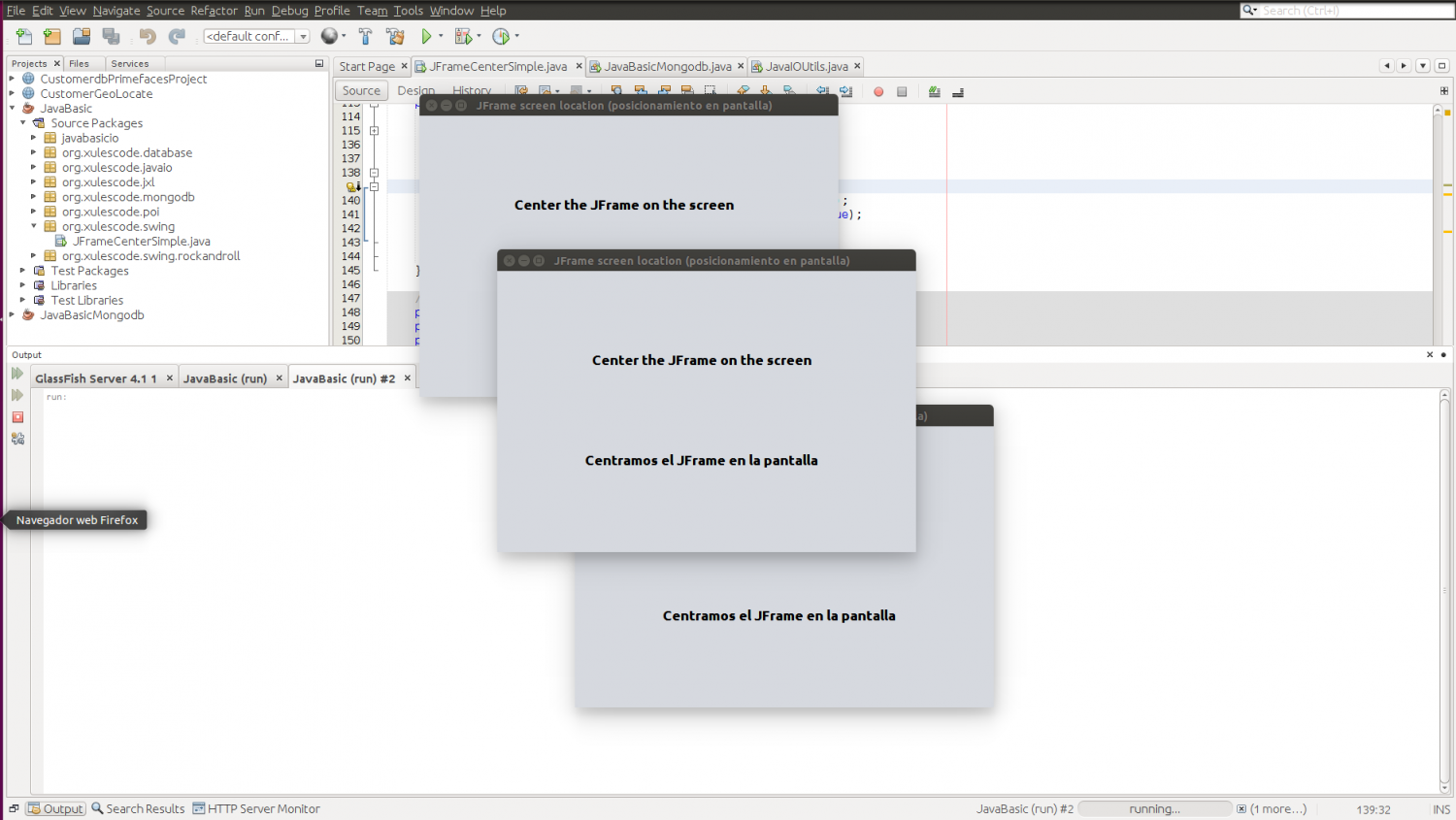
Espero que te sea útil.
Aquí os dejo el primero de una serie de códigos sobre Java Swing ( http://codigoxules.org/java/java-swing/) que iré publicando próximamente.
El objetivo de este código es explicar como centrar un JFrame o colocarlo en la posición que quieras de forma sencilla. Estos es el método que se puede utilizar en cualquier clase con JFrame:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
/**
* Place the JFrame with the parameters by moving the component relative to the center of the screen.
* Colocamos el JFrame con los parámetros desplazando el componente respecto al centro de la pantalla.
* @param moveWidth int positive or negative offset width (desplazamiente de width positivo o negativo).
* @param moveHeight int Positive or negative offset height (desplazamiento de height positivo o negativo).
*/
public void setLocationMove(int moveWidth, int moveHeight) {
// Obtenemos el tamaño de la pantalla.
Dimension screenSize = Toolkit.getDefaultToolkit().getScreenSize();
// Obtenemos el tamaño de nuestro frame.
Dimension frameSize = this.getSize();
frameSize.width = frameSize.width > screenSize.width?screenSize.width:frameSize.width;
frameSize.height = frameSize.height > screenSize.height?screenSize.height:frameSize.height;
// We define the location. Definimos la localización.
setLocation((screenSize.width - frameSize.width) / 2 + moveWidth, (screenSize.height - frameSize.height) / 2 + moveHeight);
}
1
2
3
4
5
6
7
/**
* Set the JFrame in the center of the screen.
* Colocamos nuestro JFrame en el centro de la pantalla.
*/
public void setLocationCenter(){
setLocationMove(0, 0);
}
En el método main encontrarás el ejemlo de utilización:
1
2
3
new JFrameCenterSimple(100, 200).setVisible(true);
new JFrameCenterSimple(-100, -200).setVisible(true);
new JFrameCenterSimple().setVisible(true);
Este será el resultado del ejemplo usando:
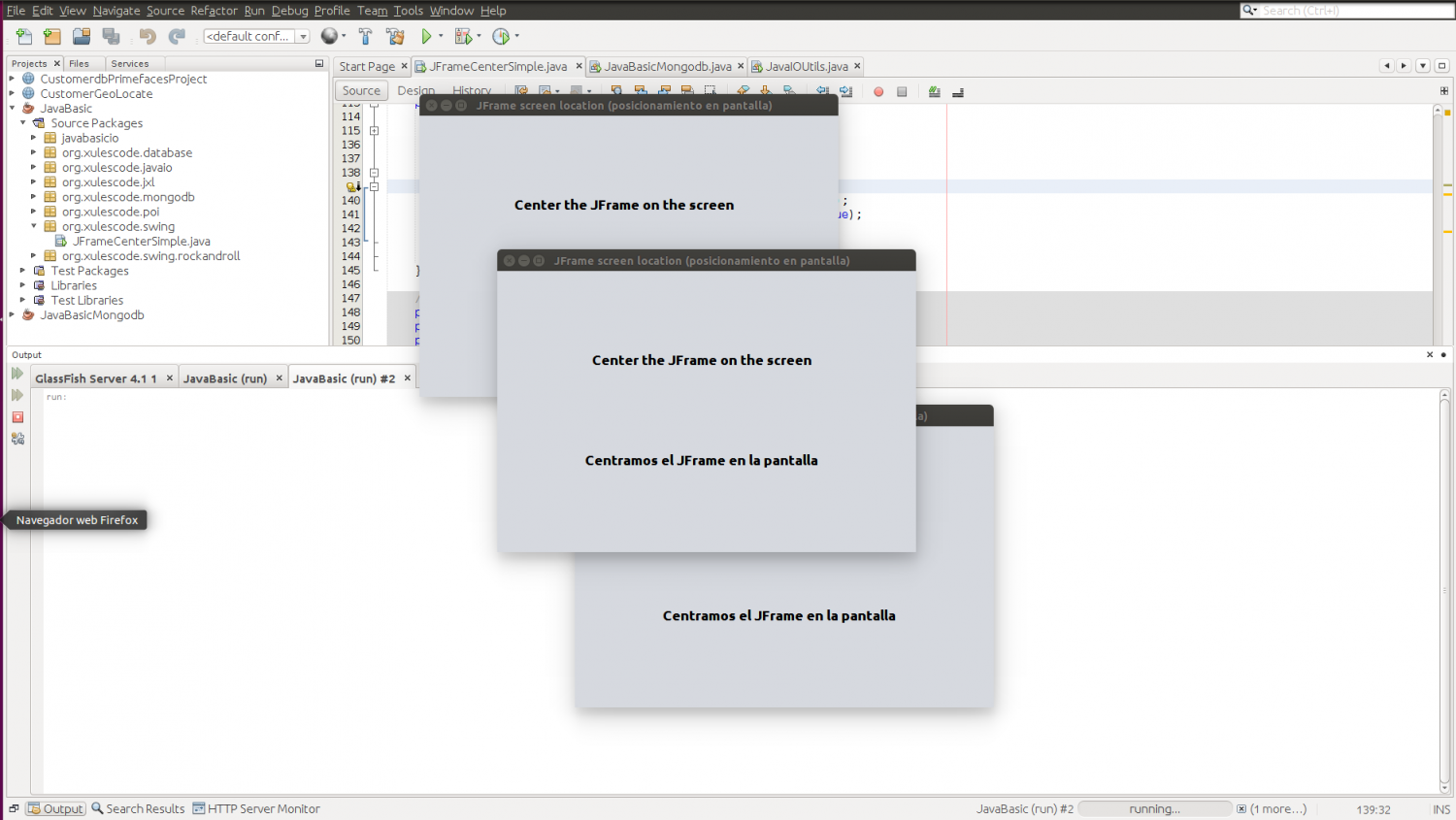
Espero que te sea útil.
Comentarios sobre la versión: 1.0 (2)
En este ejemplo... Mi jFrame se llama jFRM_Menu, en la parte del public simplemente se pone "setLocationRelativeTo(null);" sin las comillas y debajo de initComponents(); como en el ejemplo de abajo:
public jFRM_Menu() {
initComponents();
setLocationRelativeTo(null);
}
Espero que le sea de utilidad a alguien más ya que me ha servido bastante ésta pequeña sección de código.
Saludos.