Imprimir JTable directamente en Java
Java
Publicado el 18 de Enero del 2016 por Julio (12 códigos)
17.823 visualizaciones desde el 18 de Enero del 2016
Vamos con otro ejemplo sencillo con Java Swing en este caso vamos a ver como podemos imprimir automáticamente y de forma sencilla el contenido de cualquier JTable directamente por la impresora del sistema utilizando el método de JTable print.
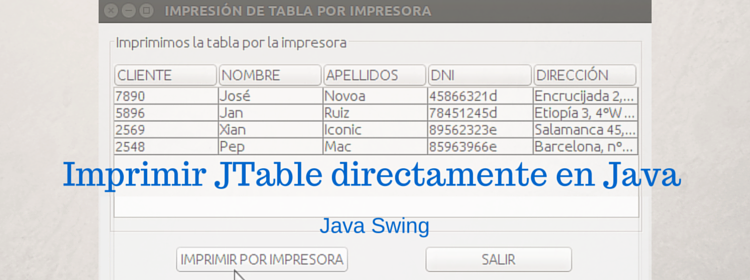
Para poder utilizar este método con cualquier JTable voy a crear un método donde pasaré por parámetros los elementos principales que me interesan: public void utilJTablePrint(JTable jTable, String header, String footer, boolean showPrintDialog).
Espero que te sea útil.
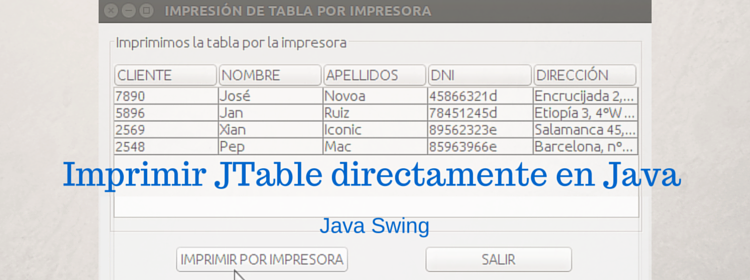
Para poder utilizar este método con cualquier JTable voy a crear un método donde pasaré por parámetros los elementos principales que me interesan: public void utilJTablePrint(JTable jTable, String header, String footer, boolean showPrintDialog).
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
/**
* Standard method to print a JTable to the printer directly..
* Método estándar para imprimir un JTable por la impresora directamente.
* <h3>Example (Ejemplo)</h3>
* <pre>
* utilJTablePrint(jTable2, getTitle(), "Código Xules", true);
* </pre>
*
* @param jTable <code>JTable</code>
* the JTable we are going to extract to excel
* El Jtable que vamos a extraer a excel.
* @param header <code>String</code>
* Header to print in the document.
* Cabecera que imprimiremos en el documento.
* @param footer <code>String</code>
* Footer to print in the document.
* Pie de página que imprimiremos en el documento.
* @param showPrintDialog <code>boolean</code>
* To show or not the print dialog.
* Mostramos o no el diálogo de impresión.
*/
public void utilJTablePrint(JTable jTable, String header, String footer, boolean showPrintDialog){
boolean fitWidth = true;
boolean interactive = true;
// We define the print mode (Definimos el modo de impresión)
JTable.PrintMode mode = fitWidth ? JTable.PrintMode.FIT_WIDTH : JTable.PrintMode.NORMAL;
try {
// Print the table (Imprimo la tabla)
boolean complete = jTable.print(mode,
new MessageFormat(header),
new MessageFormat(footer),
showPrintDialog,
null,
interactive);
if (complete) {
// Mostramos el mensaje de impresión existosa
JOptionPane.showMessageDialog(jTable,
"Print complete (Impresión completa)",
"Print result (Resultado de la impresión)",
JOptionPane.INFORMATION_MESSAGE);
} else {
// Mostramos un mensaje indicando que la impresión fue cancelada
JOptionPane.showMessageDialog(jTable,
"Print canceled (Impresión cancelada)",
"Print result (Resultado de la impresión)",
JOptionPane.WARNING_MESSAGE);
}
} catch (PrinterException pe) {
JOptionPane.showMessageDialog(jTable,
"Print fail (Fallo de impresión): " + pe.getMessage(),
"Print result (Resultado de la impresión)",
JOptionPane.ERROR_MESSAGE);
}
}
Espero que te sea útil.
Comentarios sobre la versión: 1.0 (7)
muchas gracias
He realizado lo que indicas y funciona perfectamente, el problema que me da es que al sacar la jtable en formato PDF, las columnas tienen la misma longitud y no se ven los datos correctamente.
¿Cómo se puede hacer para que se respete la anchura de la columna?
Gracias.