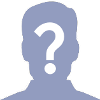
Impresion de tickets C#
Publicado por MARCELO (5 intervenciones) el 19/05/2017 05:44:05
Buenas noches:
Quisiera pedir ayuda con un programa que imprime tickets de venta, tengo hecha toda la impresión de tickets pero necesito agregar una imagen de cabecera y un código de barras al final y no se como hacerlo :(, espero me puedan ayudar dejo el código para que lo revisen y por si a alguien le sirve.
Código clase CrearTicket
Código botón que imprime
Quisiera pedir ayuda con un programa que imprime tickets de venta, tengo hecha toda la impresión de tickets pero necesito agregar una imagen de cabecera y un código de barras al final y no se como hacerlo :(, espero me puedan ayudar dejo el código para que lo revisen y por si a alguien le sirve.
Código clase CrearTicket
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
270
271
272
273
274
275
276
277
278
279
280
281
282
283
284
285
286
287
288
289
290
291
292
293
294
295
296
297
298
299
300
301
302
303
304
305
306
307
308
309
310
311
312
313
314
315
316
317
318
319
320
321
322
323
324
325
326
327
328
329
330
331
332
333
334
335
336
337
338
339
340
341
342
343
344
345
346
347
348
349
350
351
352
353
354
355
356
357
358
359
360
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Drawing; using System.Drawing.Printing; using System.Runtime.InteropServices;
namespace sistema_compu {
class CrearTicket {
StringBuilder linea = new StringBuilder();
public string lineasGuio()
{
string lineasGuion = "";
for (int i = 0; i < maxCar; i++)
{
lineasGuion += "-";
}
return linea.AppendLine(lineasGuion).ToString();
}
public string lineasAsteriscos()
{
string lineasAsterisco = "";
for (int i = 0; i < maxCar; i++)
{
lineasAsterisco += "*";
}
return linea.AppendLine(lineasAsterisco).ToString();
}
public string lineasIgual()
{
string lineasIgual = "";
for (int i = 0; i < maxCar; i++)
{
lineasIgual += "=";
}
return linea.AppendLine(lineasIgual).ToString();
}
public void TextoIzquierda(string texto)
{
if (texto.Length > maxCar)
{
int caracterActual = 0;
for (int longitudTexto = texto.Length; longitudTexto > maxCar; longitudTexto -= maxCar)
{
linea.AppendLine(texto.Substring(caracterActual, maxCar));
caracterActual += maxCar;
}
linea.AppendLine(texto.Substring(caracterActual, texto.Length - caracterActual));
}
else
{
linea.AppendLine(texto);
}
}
public void TextoDerecha(string texto)
{
if (texto.Length > maxCar)
{
int caracterActual = 0;
for (int longitudTexto = texto.Length; longitudTexto > maxCar; longitudTexto -= maxCar)
{
linea.AppendLine(texto.Substring(caracterActual, maxCar));
caracterActual += maxCar;
}
string espacios = "";
for (int i = 0; i < (maxCar - texto.Substring(caracterActual, texto.Length - caracterActual).Length); i++)
{
espacios += " ";
}
linea.AppendLine(espacios + texto.Substring(caracterActual, texto.Length - caracterActual));
}
else
{
string espacios = "";
for (int i = 0; i < (maxCar - texto.Length); i++)
{
espacios += " ";
}
linea.AppendLine(espacios + texto);
}
}
public void TextoCentro(string texto)
{
if (texto.Length > maxCar)
{
int caracterActual = 0;
for (int longitudTexto = texto.Length; longitudTexto > maxCar; longitudTexto -= maxCar)
{
linea.AppendLine(texto.Substring(caracterActual, maxCar));
caracterActual += maxCar;
}
string espacios = "";
int centrar = (maxCar - texto.Substring(caracterActual, texto.Length - caracterActual).Length) / 2;
for (int i = 0; i < centrar; i++)
{
espacios += " ";
}
linea.AppendLine(espacios + texto.Substring(caracterActual, texto.Length - caracterActual));
}
else
{
string espacios = "";
int centrar = (maxCar - texto.Length) / 2;
for (int i = 0; i < centrar; i++)
{
espacios += " ";
}
linea.AppendLine(espacios + texto);
}
}
public void TextoExtremos(string textoIzquierdo, string textoDerecho)
{
string textoIzq, textoDer, textoCompleto = "", espacios = "";
if (textoIzquierdo.Length > 22)
{
cortar = textoIzquierdo.Length - 22;
textoIzq = textoIzquierdo.Remove(22, cortar);
}
else
{ textoIzq = textoIzquierdo; }
textoCompleto = textoIzq;
if (textoDerecho.Length > 24)
{
cortar = textoDerecho.Length - 24;
textoDer = textoDerecho.Remove(24, cortar);
}
else
{ textoDer = textoDerecho; }
int nroEspacios = maxCar - (textoIzq.Length + textoDer.Length);
for (int i = 0; i < nroEspacios; i++)
{
espacios += " ";
}
textoCompleto += espacios + textoDerecho;
linea.AppendLine(textoCompleto);//agregamos la linea al ticket, al objeto en si.
}
public void EncabezadoVenta()
{
linea.AppendLine("ITEM |CANT |PRECIO ");
}
public void AgregarTotales(string texto, decimal total)
{
string resumen, valor, textoCompleto, espacios = "";
if (texto.Length > 29)
{
cortar = texto.Length - 29;
resumen = texto.Remove(29, cortar);
}
else
{ resumen = texto; }
textoCompleto = resumen;
valor = total.ToString("#,#.00");
int nroEspacios = maxCar - (resumen.Length + valor.Length);
for (int i = 0; i < nroEspacios; i++)
{
espacios += " ";
}
textoCompleto += espacios + valor;
linea.AppendLine(textoCompleto);
}
public void AgregaArticulo(string articulo, int cant, decimal precio)
{
if (cant.ToString().Length <= 7 && precio.ToString().Length <= 11)
{
string elemento = "", espacios = "";
bool bandera = false;
int nroEspacios = 0;
if (articulo.Length > 24)
{
nroEspacios = (7 - cant.ToString().Length);
espacios = "";
for (int i = 0; i < nroEspacios; i++)
{
espacios += " ";
}
elemento += espacios + cant.ToString();
nroEspacios = (11 - precio.ToString().Length);
espacios = "";
for (int i = 0; i < nroEspacios; i++)
{
espacios += " ";
}
elemento += espacios + precio.ToString();//Agregamos el precio a la variable elemento
int caracterActual = 0;
for (int longitudTexto = articulo.Length; longitudTexto > 24; longitudTexto -= 24)
{
if (bandera == false)
{
linea.AppendLine(articulo.Substring(caracterActual, 24) + elemento);
bandera = true;
}
else
linea.AppendLine(articulo.Substring(caracterActual, 24));
caracterActual += 24;
}
linea.AppendLine(articulo.Substring(caracterActual, articulo.Length - caracterActual));
}
else //Si no es mayor solo agregarlo, sin dar saltos de lineas
{
for (int i = 0; i < (24 - articulo.Length); i++)
{
espacios += " "; //Agrega espacios para completar los 20 caracteres
}
elemento = articulo + espacios;
nroEspacios = (7 - cant.ToString().Length);// +(20 - elemento.Length);
espacios = "";
for (int i = 0; i < nroEspacios; i++)
{
espacios += " ";
}
elemento += espacios + cant.ToString();
//Colocar el precio a la derecha.
nroEspacios = (11 - precio.ToString().Length);
espacios = "";
for (int i = 0; i < nroEspacios; i++)
{
espacios += " ";
}
elemento += espacios + precio.ToString();
linea.AppendLine(elemento);//Agregamos todo el elemento: nombre del articulo, cant, precio, importe.
}
}
else
{
linea.AppendLine("Los valores ingresados para esta fila");
linea.AppendLine("superan las columnas soportdas por éste.");
throw new Exception("Los valores ingresados para algunas filas del ticket\nsuperan las columnas soportdas por éste.");
}
}
public void CortaTicket()
{
linea.AppendLine("\x1B" + "m"); //Caracteres de corte. Estos comando varian segun el tipo de impresora
linea.AppendLine("\x1B" + "d" + "\x00"); //Avanza 9 renglones, Tambien varian
}
public void AbreCajon()
{
linea.AppendLine("\x1B" + "p" + "\x00" + "\x0F" + "\x96"); //Caracteres de apertura cajon 0
}
public void ImprimirTicket(string impresora)
{
RawPrinterHelper.SendStringToPrinter(impresora, linea.ToString()); //Imprime texto.
}
}
public class RawPrinterHelper
{
[StructLayout(LayoutKind.Sequential, CharSet = CharSet.Ansi)]
public class DOCINFOA
{
[MarshalAs(UnmanagedType.LPStr)]
public string pDocName;
[MarshalAs(UnmanagedType.LPStr)]
public string pOutputFile;
[MarshalAs(UnmanagedType.LPStr)]
public string pDataType;
}
[DllImport("winspool.Drv", EntryPoint = "OpenPrinterA", SetLastError = true, CharSet = CharSet.Ansi, ExactSpelling = true, CallingConvention = CallingConvention.StdCall)]
public static extern bool OpenPrinter([MarshalAs(UnmanagedType.LPStr)] string szPrinter, out IntPtr hPrinter, IntPtr pd);
[DllImport("winspool.Drv", EntryPoint = "ClosePrinter", SetLastError = true, ExactSpelling = true, CallingConvention = CallingConvention.StdCall)]
public static extern bool ClosePrinter(IntPtr hPrinter);
[DllImport("winspool.Drv", EntryPoint = "StartDocPrinterA", SetLastError = true, CharSet = CharSet.Ansi, ExactSpelling = true, CallingConvention = CallingConvention.StdCall)]
public static extern bool StartDocPrinter(IntPtr hPrinter, Int32 level, [In, MarshalAs(UnmanagedType.LPStruct)] DOCINFOA di);
[DllImport("winspool.Drv", EntryPoint = "EndDocPrinter", SetLastError = true, ExactSpelling = true, CallingConvention = CallingConvention.StdCall)]
public static extern bool EndDocPrinter(IntPtr hPrinter);
[DllImport("winspool.Drv", EntryPoint = "StartPagePrinter", SetLastError = true, ExactSpelling = true, CallingConvention = CallingConvention.StdCall)]
public static extern bool StartPagePrinter(IntPtr hPrinter);
[DllImport("winspool.Drv", EntryPoint = "EndPagePrinter", SetLastError = true, ExactSpelling = true, CallingConvention = CallingConvention.StdCall)]
public static extern bool EndPagePrinter(IntPtr hPrinter);
[DllImport("winspool.Drv", EntryPoint = "WritePrinter", SetLastError = true, ExactSpelling = true, CallingConvention = CallingConvention.StdCall)]
public static extern bool WritePrinter(IntPtr hPrinter, IntPtr pBytes, Int32 dwCount, out Int32 dwWritten);
public static bool SendBytesToPrinter(string szPrinterName, IntPtr pBytes, Int32 dwCount)
{
Int32 dwError = 0, dwWritten = 0;
IntPtr hPrinter = new IntPtr(0);
DOCINFOA di = new DOCINFOA();
bool bSuccess = false; // Assume failure unless you specifically succeed.
di.pDocName = "Ticket de Venta";
di.pDataType = "RAW";
if (OpenPrinter(szPrinterName.Normalize(), out hPrinter, IntPtr.Zero))
{
if (StartDocPrinter(hPrinter, 1, di))
{
if (StartPagePrinter(hPrinter))
{
bSuccess = WritePrinter(hPrinter, pBytes, dwCount, out dwWritten);
EndPagePrinter(hPrinter);
}
EndDocPrinter(hPrinter);
}
ClosePrinter(hPrinter);
}
if (bSuccess == false)
{
dwError = Marshal.GetLastWin32Error();
}
return bSuccess;
}
public static bool SendStringToPrinter(string szPrinterName, string szString)
{
IntPtr pBytes;
Int32 dwCount;
// How many characters are in the string?
dwCount = szString.Length;
// Assume that the printer is expecting ANSI text, and then convert
// the string to ANSI text.
pBytes = Marshal.StringToCoTaskMemAnsi(szString);
// Send the converted ANSI string to the printer.
SendBytesToPrinter(szPrinterName, pBytes, dwCount);
Marshal.FreeCoTaskMem(pBytes);
return true;
}
}
}
Código botón que imprime
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
private void btnImprimir_Click(object sender, EventArgs e) { try { CrearTicket ticket = new CrearTicket();
ticket.TextoIzquierda(" ");
ticket.TextoCentro("TICKET CIERRE DE CAJA");
ticket.TextoIzquierda(" ");
ticket.TextoExtremos("FECHA : " + txtFecha.Text, "HORA : " + txtHora.Text);
ticket.TextoIzquierda(" ");
ticket.EncabezadoVenta();
ticket.lineasGuio();
foreach (DataGridViewRow fila in dataGridView1.Rows)
{
ticket.AgregaArticulo(fila.Cells[1].Value.ToString(), int.Parse(fila.Cells[0].Value.ToString()) , decimal.Parse(fila.Cells[3].Value.ToString()));
}
ticket.lineasIgual();
ticket.AgregarTotales(" TOTAL COMPRADO : $ ", decimal.Parse(txtCompra.Text));
ticket.AgregarTotales(" TOTAL VENDIDO : $ ", decimal.Parse(txtVenta.Text));
ticket.TextoIzquierda(" ");
ticket.AgregarTotales(" GANANCIA : $ ", decimal.Parse(txtResultado.Text));
ticket.TextoIzquierda(" ");
ticket.TextoIzquierda(" ");
ticket.TextoIzquierda(" ");
ticket.TextoIzquierda(" ");
ticket.TextoIzquierda(" ");
ticket.TextoIzquierda(" ");
ticket.CortaTicket();
ticket.ImprimirTicket("EPSON TM-T20II Receipt");
}
catch (Exception eeee) { }
}
Valora esta pregunta


0