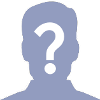
Metodo burbuja
C/Visual C
52.234 visualizaciones desde el 16 de Abril del 2005
Código que solicita 10 números y los ordena ascendentemente y descendentemente utilizando el método de la burbuja.
Probado con GNU g++.
Probado con GNU g++.
#include <iostream>
using namespace std;
void mostrar_datos(int valores[]);
int main()
{
int valores[10];
int valor_temporal;
int i, ii;
int valor_suma=0;
int valor_mayor=0;
int valor_menor=9999999;
//lee diez valores enteror del teclado y los coloca en un array
for(i=0;i<10;i++)
{
cout << "Introduce el valor " << i << " : " ;
cin >> valores[i];
valor_suma+=valores[i];
if(valor_mayor<valores[i])
valor_mayor=valores[i];
if(valor_menor>valores[i])
valor_menor=valores[i];
}
//mostramos los valores introducidos
cout << "\nLos valores introducidos son: ";
mostrar_datos(valores);
//ordenamos los valores ascendente
for(i=0;i<10;i++)
{
for(ii=i+1;ii<10;ii++)
{
if(valores[i]>valores[ii])
{
valor_temporal=valores[ii];
valores[ii]=valores[i];
valores[i]=valor_temporal;
}
}
}
//mostramos los valores ordenador ascendentes
cout << "\nLos valores ordenados ascendentemente son: ";
mostrar_datos(valores);
//ordenamos los valores descendente
for(i=0;i<10;i++)
{
for(ii=i+1;ii<10;ii++)
{
if(valores[i]<valores[ii])
{
valor_temporal=valores[ii];
valores[ii]=valores[i];
valores[i]=valor_temporal;
}
}
}
//mostramos los valores ordenador descendente
cout << "\nLos valores ordenados descendente son: ";
mostrar_datos(valores);
cout << "\n";
cout << "\nLa suma total es : " << valor_suma;
cout << "\nEl valor maximos es : " << valor_mayor;
cout << "\nEl valor minimo es : " << valor_menor;
cout << "\n\n";
}
void mostrar_datos(int valores[])
{
int i;
for(i=0;i<10;i++)
{
cout << valores[i];
if(i!=9) cout << " - ";
}
}
Comentarios sobre la versión: Versión 1 (3)
#include <stdlib.h>
#include <conio.h>
#include <string>
#define N 5 //Define una constante con N=5
using namespace std;
main(){
int tmp,iaux;
int array[N];
//Inicializacion del arreglo, con los 5 elementos
cout<<"INGRESE 5 NUMEROS QUE DESEE ORDENAR "<<"\n\n";
for(int i=0;i<N;i++){
cout<<"INGRESE EL NUMERO EN LA POSICION "<<i<<": ";
cin>>array[i];
}
cout<<"\nNUMEROS ORDENADOS ASCENDENTEMENTE"<<"\n";
//BubbleSort que ordena los numeros ascendentemente.
for(int i=0;i<N;i++){
for(int j=0;j<N-1;j++){
if (array[j] > array[j + 1])
{
iaux = array[j];
array[j] = array[j + 1];
array[j + 1] = iaux;
}
}
}
//Despliega el arreglo que ordena los numeros ascendentemente
for(int i=0;i<N;i++){
cout<<array[i]<<"\n";
}
cout<<"\n\nNUMEROS ORDENADOS DESCENDENTEMENTE"<<"\n";
//BubbleSort que ordena los numeros descendentemente
for(int i=0;i<N;i++){
for(int j=0;j<N-1;j++){
if (array[j] < array[j + 1])
{
iaux = array[j];
array[j] = array[j + 1];
array[j + 1] = iaux;
}
}
}
/*Puede sustituirlo por la funcion por
if(array[j]>array[j+1]){
swap(array[j], array[j+1]); // funcion que hace el intercambio
*/
//Despliega el arreglo que ordena los numeros descendentemente
for(int i=0;i<N;i++){
cout<<array[i]<<"\n";
}
getch();
}